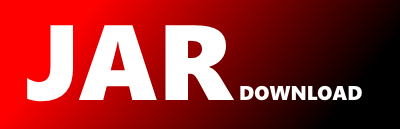
com.daioware.net.http.HttpResponse Maven / Gradle / Ivy
package com.daioware.net.http;
import java.io.File;
import java.io.IOException;
import java.nio.charset.Charset;
import java.util.List;
import java.util.Map;
import com.daioware.commons.wrapper.WrapperString;
import com.daioware.file.MyRandomAccessFile;
import com.daioware.net.http.client.ResponseBodyException;
import com.daioware.net.http.items.HttpHeader;
import com.daioware.net.http.request.HttpRequest;
public class HttpResponse {
private HttpRequest request;
private Map headers;
private List body;
private String version;
private Integer status;
private String statusMessage;
private File file;
public HttpResponse(Map headers, List body, String version,
Integer status, String statusMessage,HttpRequest request) {
this.headers = headers;
this.body = body;
this.version = version;
this.status = status;
this.statusMessage = statusMessage;
this.request=request;
}
public Map getHeaders() {
return headers;
}
public List getBody() {
return body;
}
public String getVersion() {
return version;
}
public Integer getStatus() {
return status;
}
public String getStatusMessage() {
return statusMessage;
}
protected void setHeaders(Map headers) {
this.headers = headers;
}
protected void setBody(List body) {
this.body = body;
}
protected void setVersion(String version) {
this.version = version;
}
protected void setStatus(Integer status) {
this.status = status;
}
protected void setStatusMessage(String statusMessage) {
this.statusMessage = statusMessage;
}
public HttpRequest getRequest() {
return request;
}
public String getBodyAsString() throws ResponseBodyException {
HttpHeader header=headers.get("Content-Type");
WrapperString mime,charsetWrapper;
Charset wrapper;
if(header!=null) {
mime=new WrapperString();
charsetWrapper=new WrapperString();
HttpUtil.parseContentType(mime,charsetWrapper,header.getValue());
if(!HttpUtil.isPlainTextMimeType(mime.value)) {
throw new ResponseBodyException("Mime type '"+mime.value+"' is not valid for plain text");
}
wrapper=Charset.forName(charsetWrapper.value);
}
else {
wrapper=Charset.forName(HttpUtil.DEFAULT_ENCODING);
}
return getBodyAsString(wrapper);
}
public String getBodyAsString(Charset charset) {
byte bytes[]=new byte[body.size()];
int i=0;
for(byte b:body) {
bytes[i++]=b;
}
return new String(bytes,0,bytes.length,charset);
}
public File getBodyAsFile(File file) throws IOException {
if(this.file!=null) {//let's rename the file that has been downloaded previously
if(this.file.renameTo(file)) {
this.file=file;
}
}
else {
this.file=file;
try(MyRandomAccessFile raFile=new MyRandomAccessFile(file, "rw")) {
raFile.setLength(0);
byte bytes[]=new byte[body.size()];
int s=0;
for(byte b:body) {
bytes[s++]=b;
}
raFile.write(bytes);
}
}
return this.file;
}
public File getFile() {
return file;
}
public void setFile(File file) {
this.file = file;
}
public File getBodyAsFile() throws IOException {
File file=getFile();
if(file==null) {
String path=request.getUrl().getPath();
String fileName;
if(path.length()>=1 && path.charAt(path.length()-1)=='/') {
fileName=request.getFileName();
}
else{
fileName=new File(path).getAbsolutePath()+File.separator+request.getFileName();
}
fileName=HttpUtil.decodePath(fileName, request.getEncoding());
file=new File(fileName);
getBodyAsFile(file);
}
//else file already downloaded while downloading the bytes in HttpSender
return file;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy