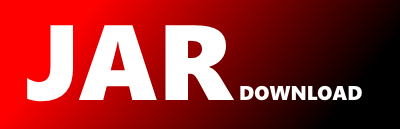
com.daioware.thread.ThreadPool Maven / Gradle / Ivy
package com.daioware.thread;
import java.util.List;
import com.daioware.collections.concurrent.ConcurrentLinkedList;
public class ThreadPool {
private List threadsRunning;
private List queue;
private int simThreads;
private int maxInQueue;
private boolean active;
public ThreadPool() {
this(5,5);
}
public ThreadPool(int simThreads,int maxInQueue) {
threadsRunning=new ConcurrentLinkedList<>();
queue=new ConcurrentLinkedList<>();
setSimThreads(5);
setMaxInQueue(maxInQueue);
setActive(true);
activateRunnable();
}
public void activateRunnable() {
ThreadPool pool=this;
new Thread(new Runnable() {
@Override
public synchronized void run() {
while(isActive()) {
try {
synchronized (pool) {
pool.wait();
}
new Thread(()->pool.checkUpdates()).start();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
}).start();
}
public int getMaxInQueue() {
return maxInQueue;
}
public void setMaxInQueue(int maxInQueue) {
this.maxInQueue = maxInQueue;
}
private synchronized void checkUpdates() {
for(int i=getSimThreads()-threadsRunning.size();i>0 && !queue.isEmpty();i--) {
run(queue.remove(0));
}
}
protected void run(Runnable r) {
ChildThread c=new ChildThread(this,r);
threadsRunning.add(c);
new Thread(c).start();
}
public int getSimThreads() {
return simThreads;
}
public void setSimThreads(int simThreads) {
this.simThreads = simThreads;
}
public boolean isActive() {
return active;
}
public void setActive(boolean active) {
this.active = active;
if(!active) {
synchronized (this) {
notify();
}
}
}
public synchronized boolean exec(Runnable r) {
ChildThread child=new ChildThread(this,r);
queue.add(child);
if(queue.size()=1 || queue.size()>=1) {
try {
wait();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
setActive(false);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy