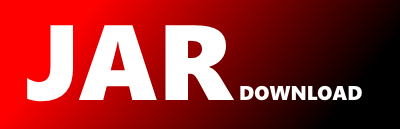
com.danielflower.apprunner.runners.AppRunnerFactoryProvider Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of app-runner Show documentation
Show all versions of app-runner Show documentation
A self-hosted platform-as-a-service that hosts web apps written in Java, Clojure, NodeJS, Python, golang and Scala.
package com.danielflower.apprunner.runners;
import com.danielflower.apprunner.Config;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.io.File;
import java.util.ArrayList;
import java.util.List;
import java.util.Optional;
import java.util.concurrent.*;
import static java.util.stream.Collectors.joining;
public class AppRunnerFactoryProvider {
public static final Logger log = LoggerFactory.getLogger(AppRunnerFactoryProvider.class);
private final List factories;
public AppRunnerFactoryProvider(List factories) {
this.factories = factories;
}
public static AppRunnerFactoryProvider create(Config config) throws ExecutionException, InterruptedException {
// This is done asyncronously because around half the startup time in tests was due to these calls.
ExecutorService executorService = Executors.newFixedThreadPool(6 /* the number of factories */);
List>> futures = new ArrayList<>();
futures.add(executorService.submit(() -> MavenRunnerFactory.createIfAvailable(config)));
futures.add(executorService.submit(() -> NodeRunnerFactory.createIfAvailable(config)));
futures.add(executorService.submit(() -> LeinRunnerFactory.createIfAvailable(config)));
futures.add(executorService.submit(() -> SbtRunnerFactory.createIfAvailable(config)));
futures.add(executorService.submit(() -> GoRunnerFactory.createIfAvailable(config)));
futures.add(executorService.submit(() -> GradleRunnerFactory.createIfAvailable(config)));
futures.add(executorService.submit(() -> PythonRunnerFactory.createIfAvailable(config, 2)));
futures.add(executorService.submit(() -> PythonRunnerFactory.createIfAvailable(config, 3)));
futures.add(executorService.submit(() -> RustRunnerFactory.createIfAvailable(config)));
futures.add(executorService.submit(() -> DotnetRunnerFactory.createIfAvailable(config)));
List factories = new ArrayList<>();
for (Future> future : futures) {
future.get().ifPresent(factories::add);
}
executorService.shutdown();
return new AppRunnerFactoryProvider(factories);
}
public AppRunnerFactory runnerFor(String appName, File projectRoot) throws UnsupportedProjectTypeException {
for (AppRunnerFactory factory : factories) {
if (factory.canRun(projectRoot)) {
return factory;
}
}
throw new UnsupportedProjectTypeException("No app runner found for " + appName);
}
public String describeRunners() {
return factories.stream()
.map(AppRunnerFactory::versionInfo)
.collect(joining(System.lineSeparator()));
}
public List factories() {
return factories;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy