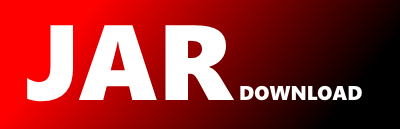
com.darylteo.rx.promises.AbstractPromise Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rxjava-promises-core Show documentation
Show all versions of rxjava-promises-core Show documentation
Promises implementation for RxJava - rxjava-promises-core
The newest version!
package com.darylteo.rx.promises;
import rx.Observable;
import rx.Observer;
import rx.exceptions.OnErrorThrowable;
import rx.functions.*;
import rx.subjects.ReplaySubject;
public abstract class AbstractPromise implements Observer {
public static enum STATE {
PENDING,
FULFILLED,
REJECTED
}
/* Properties */
private AbstractPromise that = this;
private ReplaySubject subject;
private Observable obs;
private STATE state = STATE.PENDING;
private T value = null;
private Throwable reason;
public STATE getState() {
return this.state;
}
public boolean isPending() {
return this.state == STATE.PENDING;
}
public boolean isFulfilled() {
return this.state == STATE.FULFILLED;
}
public boolean isRejected() {
return this.state == STATE.REJECTED;
}
public T getValue() {
return this.value;
}
public Throwable getReason() {
return this.reason;
}
/* Constructor */
public AbstractPromise() {
this(null);
}
public AbstractPromise(Observable source) {
this.subject = ReplaySubject.create();
this.obs = this.subject.last();
if (source != null) {
source.subscribe(this.subject);
}
// promise states
this.obs.subscribe(new Observer() {
@Override
public void onCompleted() {
that.state = STATE.FULFILLED;
}
@Override
public void onError(Throwable reason) {
that.state = STATE.REJECTED;
that.reason = reason;
}
@Override
public void onNext(T value) {
that.value = value;
}
});
}
/* ================== */
/* Main Defer Function */
protected AbstractPromise _then(
final Function onFulfilled,
final Function onRejected,
final Function onFinally) {
// This is the next promise in the chain.
// The handlers you see below will resolve their values and forward them
// to this promise.
final AbstractPromise deferred = this._create();
// Create the Observer
final Observer observer = new Observer() {
@Override
public void onCompleted() {
this.evaluate();
}
@Override
public void onError(Throwable reason) {
this.evaluate();
}
@Override
public void onNext(T value) {
}
private void evaluate() {
try {
// onfinally and onFulfilled/onRejected are mutually exclusive
// note: this implementation of finally is closer to "onComplete" rather than "finallyDo"
// in that it fires immediately when the previous Promise is fulfilled, rather than
// for the entire sequence of Observables to complete its sequence.
if (onFinally != null) {
evaluateFinally();
return;
}
// No finally block was provided, thus we need to evaluate fulfillment
// or rejection.
// If the appropriate handler is not provided, it is forwarded to the
// next promise
if (that.state == STATE.FULFILLED) {
evaluateFulfilled();
return;
}
if (that.state == STATE.REJECTED) {
evaluateRejected();
return;
}
} catch (Throwable e) {
// On any exception in the handlers above, we should throw the
// exception to the next promise
deferred.reject(e);
}
}
private void evaluateFinally() {
AbstractPromise> result = callFinally();
if (result != null) {
// the finally block returned a promise, so we need to delay
// fulfillment of the next promise until the returned promise is
// fulfilled
((AbstractPromise super Object>) result)._then(
new Action1
© 2015 - 2025 Weber Informatics LLC | Privacy Policy