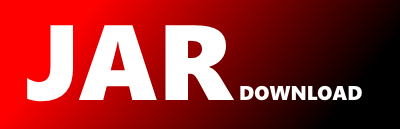
com.darylteo.rx.promises.java.Promise Maven / Gradle / Ivy
package com.darylteo.rx.promises.java;
import java.util.LinkedHashMap;
import rx.Observer;
import rx.Subscription;
import rx.util.functions.Function;
import com.darylteo.rx.promises.AbstractPromise;
import com.darylteo.rx.promises.java.Promise;
import com.darylteo.rx.promises.java.functions.FinallyAction;
import com.darylteo.rx.promises.java.functions.FinallyFunction;
import com.darylteo.rx.promises.java.functions.PromiseAction;
import com.darylteo.rx.promises.java.functions.PromiseFunction;
import com.darylteo.rx.promises.java.functions.RepromiseFunction;
/**
* A Promise represents a request that will be fulfilled sometime in the future, most usually by an asynchrous task executed on the Vert.x Event Loop. It allows you to assign handlers to deal with the return results of asynchronus tasks, and to flatten "pyramids of doom" or "callback hell".
*
* Promise Rules
*
* - A Promise represents a value that is set in some future time (usually in another cycle of the event loop)
* - Each promise has three components: onFulfilled, onRejected, and onFinally
* - If the promise is fulfilled, onFulfilled is called with the value of the promise.
* - If the promise cannot be fulfilled for some reason, it is then rejected. onRejected is called with the reason for the rejection
* - A promise may be further deferred, at which point a new promise is provided. This can lead to a chain of promises.
* - If a promise if fulfilled, but onFulfilled is not provided, then the promise is fulfilled with the same value.
* - If a promise is rejected, but onRejected is not provided, then the next promise is rejected with the same reason
* - If onFinally is provided, it is resolved first before either fulfilling or rejecting the next promise (see previous two points)
*
- Either onFulfilled, or onRejected may return a new promise (i.e. a repromise) . When this happens, the subsequently created promise will be fulfilled with the value of the repromise when it is eventually fulfilled.
*
*
* Type-Safe Rules
*
* - All the type-safety rules are related to the output type of onFulfilled.
* - If onFulfilled returns type T, then onRejected must either return T, or null. This is facilitated through the use of PromiseFunction, and PromiseAction respectively.
* - As per the previous rule, if onFulfilled is an PromiseAction, then onRejected must also be an PromiseAction.
* - You may use a RepromiseFunction in place of a PromiseFunction that returns T.
* - If onFulfilled is not provided, it is assumed that it is defined in a future handler. As such, onRejected may not change the return type.
* - onFinally must be a Repromise or an Action. It does not accept any values. This is facilitated through the use of FinallyFunction and FinallyAction respectively.
*
*
* @author Daryl Teo
*
* @param T
* - the data type of the result contained by this Promise.
*/
public class Promise extends AbstractPromise {
public static Promise defer() {
return new Promise();
}
public Promise() {
super(new LinkedHashMap>());
}
/* ================== */
/* Strictly Typed Defer Methods */
// then(onFulfilled)
public Promise then(PromiseFunction onFulfilled) {
return this.promise(onFulfilled, null, null);
}
public Promise then(RepromiseFunction onFulfilled) {
return this.promise(onFulfilled, null, null);
}
public Promise then(PromiseAction onFulfilled) {
return this.promise(onFulfilled, null, null);
}
// then(onFulfilled, onRejected)
public Promise then(PromiseFunction onFulfilled,
PromiseFunction onRejected) {
return this.promise(onFulfilled, onRejected, null);
}
public Promise then(PromiseFunction onFulfilled,
RepromiseFunction onRejected) {
return this.promise(onFulfilled, onRejected, null);
}
public Promise then(PromiseFunction onFulfilled,
PromiseAction onRejected) {
return this.promise(onFulfilled, onRejected, null);
}
public Promise then(RepromiseFunction onFulfilled,
PromiseFunction onRejected) {
return this.promise(onFulfilled, onRejected, null);
}
public Promise then(RepromiseFunction onFulfilled,
RepromiseFunction onRejected) {
return this.promise(onFulfilled, onRejected, null);
}
public Promise then(RepromiseFunction onFulfilled,
PromiseAction onRejected) {
return this.promise(onFulfilled, onRejected, null);
}
public Promise then(PromiseAction onFulfilled,
PromiseAction onRejected) {
return this.promise(onFulfilled, onRejected, null);
}
// fail(onRejected)
public Promise fail(PromiseFunction onRejected) {
return this.promise(null, onRejected, null);
}
public Promise fail(RepromiseFunction onRejected) {
return this.promise(null, onRejected, null);
}
public Promise fail(PromiseAction onRejected) {
return this.promise(null, onRejected, null);
}
// fin(onFinally)
public Promise fin(FinallyFunction> onFinally) {
return this.promise(null, null, onFinally);
}
public Promise fin(FinallyAction onFinally) {
return this.promise(null, null, onFinally);
}
@SuppressWarnings("unchecked")
protected Promise promise(Function onFulfilled, Function onRejected, Function onFinally) {
return (Promise) super._then(onFulfilled, onRejected, onFinally);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy