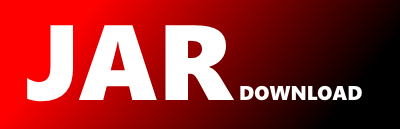
com.databasesandlife.util.CompositeIterator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of java-common Show documentation
Show all versions of java-common Show documentation
Utility classes developed at Adrian Smith Software (A.S.S.)
package com.databasesandlife.util;
import java.util.Iterator;
import java.util.NoSuchElementException;
/**
* Takes an array of iterators, and returns their results one after another.
*
* @author This source is copyright Adrian Smith and licensed under the LGPL 3.
* @see Project on GitHub
*/
public class CompositeIterator implements Iterator {
Iterator[] children;
int nextElementFrom = 0; // set to children.length means finished
public CompositeIterator(Iterator[] children) {
this.children = children;
advanceIfNecessary();
}
protected void advanceIfNecessary() {
while (nextElementFrom < children.length && !children[nextElementFrom].hasNext()) nextElementFrom++;
}
public boolean hasNext() {
return nextElementFrom < children.length;
}
public T next() {
if ( ! hasNext()) throw new NoSuchElementException();
var result = children[nextElementFrom].next();
advanceIfNecessary();
return result;
}
public void remove() {
throw new UnsupportedOperationException("Not supported yet.");
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy