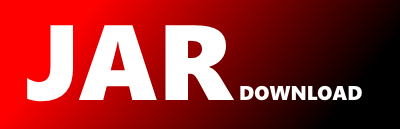
com.databasesandlife.util.WebEncodingUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of java-common Show documentation
Show all versions of java-common Show documentation
Utility classes developed at Adrian Smith Software (A.S.S.)
package com.databasesandlife.util;
import org.slf4j.LoggerFactory;
import java.io.IOException;
import java.io.UnsupportedEncodingException;
import java.net.HttpURLConnection;
import java.net.URLEncoder;
import java.nio.charset.StandardCharsets;
import java.util.HashMap;
import java.util.Map;
import java.util.Map.Entry;
import javax.servlet.http.HttpServletRequest;
/**
* @author This source is copyright Adrian Smith and licensed under the LGPL 3.
* @see Project on GitHub
*/
public class WebEncodingUtils {
public static String encodeHtml(String x) {
x = x.replaceAll("&", "&");
x = x.replaceAll(">", ">");
x = x.replaceAll("<", "<");
x = x.replaceAll("\n", "
");
return x;
}
public static Map getHttpRequestParameterMap(HttpServletRequest request) {
Map result = new HashMap<>();
for (var paramEntry : ((Map) request.getParameterMap()).entrySet())
result.put(paramEntry.getKey(), paramEntry.getValue()[0]);
return result;
}
/**
* @param params values are either String or String[]
* @return "a=b&c=d"
*/
public static CharSequence encodeGetParameters(Map params) {
try {
var result = new StringBuilder();
for (var entry : params.entrySet()) {
String[] values;
if (entry.getValue() instanceof String) values = new String[] { (String) entry.getValue() };
else if (entry.getValue() instanceof String[]) values = (String[]) entry.getValue();
else throw new RuntimeException("Unexpected type of key '" + entry.getKey() + "': " + entry.getValue().getClass());
for (var value : values) {
if (result.length() > 0) result.append("&");
result.append(URLEncoder.encode(entry.getKey(), StandardCharsets.UTF_8.name()));
result.append("=");
result.append(URLEncoder.encode(value, StandardCharsets.UTF_8.name()));
}
}
return result;
}
catch (UnsupportedEncodingException e) { throw new RuntimeException(e); }
}
public static boolean isResponseSuccess(int responseCode) {
return responseCode >= 200 && responseCode < 300;
}
public static boolean isResponseSuccess(HttpURLConnection c) {
try { return isResponseSuccess(c.getResponseCode()); }
catch (IOException e) {
LoggerFactory.getLogger(WebEncodingUtils.class).warn("HTTP request to '" + c.getURL() + "' unsuccessful", e);
return false;
}
}
// For GET parameters:
// See http://java.sun.com/j2se/1.4.2/docs/api/java/net/URLEncoder.html
/** Takes "New York" and returns "new-york" */
public static String beautifyUrl(String x) {
return x.replaceAll("[^\\p{L}\\p{N}]","-").replaceAll("-+", "-") .replaceAll("^-","").replaceAll("-$","").toLowerCase();
}
public static String dotdotdot(String x, int max) {
if (x.length() <= max) return x;
else return x.substring(0, max)+"...";
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy