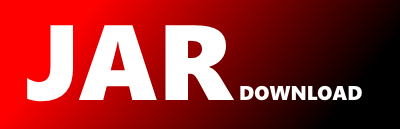
com.databricks.sql.h3Functions.scala Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of databricks-connect Show documentation
Show all versions of databricks-connect Show documentation
Develop locally and connect IDEs, notebook servers and running applications to Databricks clusters.
The newest version!
//
// DATABRICKS CONFIDENTIAL & PROPRIETARY
// __________________
//
// Copyright 2024-present Databricks, Inc.
// All Rights Reserved.
//
// NOTICE: All information contained herein is, and remains the property of Databricks, Inc.
// and its suppliers, if any. The intellectual and technical concepts contained herein are
// proprietary to Databricks, Inc. and its suppliers and may be covered by U.S. and foreign Patents,
// patents in process, and are protected by trade secret and/or copyright law. Dissemination, use,
// or reproduction of this information is strictly forbidden unless prior written permission is
// obtained from Databricks, Inc.
//
// If you view or obtain a copy of this information and believe Databricks, Inc. may not have
// intended it to be made available, please promptly report it to Databricks Legal Department
// @ [email protected].
//
package org.apache.spark.sql
import org.apache.spark.annotation.{Py4JWhitelist, Stable}
import org.apache.spark.sql.Column
import org.apache.spark.sql.functions.lit
@Py4JWhitelist
@Stable
/**
* Scala H3 functions
*
* @groupname h3_funcs H3 grid system related functions
*/
// scalastyle:off: object.name
trait h3Functions {
// scalastyle:on
/**
* Converts an H3 cell ID to a string representing the cell ID as a hexadecimal string.
*
* @group h3_funcs
* @since 3.3.0
*/
@Py4JWhitelist
def h3_h3tostring(e: Column): Column =
Column.fn("h3_h3tostring", lit(e))
/**
* Converts a string representing an H3 cell ID to the corresponding big integer.
*
* @group h3_funcs
* @since 3.3.0
*/
@Py4JWhitelist
def h3_stringtoh3(e: Column): Column =
Column.fn("h3_stringtoh3", lit(e))
/**
* Returns the resolution of the H3 cell ID.
*
* @group h3_funcs
* @since 3.3.0
*/
@Py4JWhitelist
def h3_resolution(e: Column): Column =
Column.fn("h3_resolution", lit(e))
/**
* Returns true if the input represents a valid H3 cell ID.
*
* @group h3_funcs
* @since 3.3.0
*/
@Py4JWhitelist
def h3_isvalid(e: Column): Column =
Column.fn("h3_isvalid", lit(e))
/**
* Returns true if the input H3 cell ID represents a pentagon.
*
* @group h3_funcs
* @since 3.3.0
*/
@Py4JWhitelist
def h3_ispentagon(e: Column): Column =
Column.fn("h3_ispentagon", lit(e))
/**
* Returns the parent H3 cell ID of the input H3 cell ID at the specified resolution.
*
* @group h3_funcs
* @since 3.3.0
*/
@Py4JWhitelist
def h3_toparent(e1: Column, e2: Column): Column =
Column.fn("h3_toparent", lit(e1), lit(e2))
/**
* Returns the children H3 cell IDs of the input H3 cell ID at the specified resolution.
*
* @group h3_funcs
* @since 3.3.0
*/
@Py4JWhitelist
def h3_tochildren(e1: Column, e2: Column): Column =
Column.fn("h3_tochildren", lit(e1), lit(e2))
/**
* Returns the H3 cell ID (as a BIGINT) corresponding to the provided longitude and latitude at
* the specified resolution.
*
* @group h3_funcs
* @since 3.3.0
*/
@Py4JWhitelist
def h3_longlatash3(e1: Column, e2: Column, e3: Column): Column =
Column.fn("h3_longlatash3", lit(e1), lit(e2), lit(e3))
/**
* Returns the H3 cell ID (as a STRING) corresponding to the provided longitude and latitude at
* the specified resolution.
*
* @group h3_funcs
* @since 3.3.0
*/
@Py4JWhitelist
def h3_longlatash3string(e1: Column, e2: Column, e3: Column): Column =
Column.fn("h3_longlatash3string", lit(e1), lit(e2), lit(e3))
/**
* Returns the H3 cell IDs that are within (grid) distance k of the origin cell ID.
*
* @group h3_funcs
* @since 3.3.0
*/
@Py4JWhitelist
def h3_kring(e1: Column, e2: Column): Column =
Column.fn("h3_kring", lit(e1), lit(e2))
/**
* Compacts the input set of H3 cell IDs as best as possible.
*
* @group h3_funcs
* @since 3.3.0
*/
@Py4JWhitelist
def h3_compact(e: Column): Column =
Column.fn("h3_compact", lit(e))
/**
* Uncompacts the input set of H3 cell IDs to the specified resolution.
*
* @group h3_funcs
* @since 3.3.0
*/
@Py4JWhitelist
def h3_uncompact(e1: Column, e2: Column): Column =
Column.fn("h3_uncompact", lit(e1), lit(e2))
/**
* Returns the grid distance between two H3 cell IDs.
*
* @group h3_funcs
* @since 3.3.0
*/
@Py4JWhitelist
def h3_distance(e1: Column, e2: Column): Column =
Column.fn("h3_distance", lit(e1), lit(e2))
/**
* Returns the grid distance between two H3 cell IDs of the same resolution, or NULL if the
* distance is undefined.
*
* @group h3_funcs
* @since 3.5.0
*/
@Py4JWhitelist
def h3_try_distance(e1: Column, e2: Column): Column =
Column.fn("h3_try_distance", lit(e1), lit(e2))
/**
* Returns the input value if it is a valid H3 cell or emits an error otherwise.
*
* @group h3_funcs
* @since 3.3.0
*/
@Py4JWhitelist
def h3_validate(e: Column): Column =
Column.fn("h3_validate", lit(e))
/**
* Returns the input value if it is a valid H3 cell or NULL otherwise.
*
* @group h3_funcs
* @since 3.3.0
*/
@Py4JWhitelist
def h3_try_validate(e: Column): Column =
Column.fn("h3_try_validate", lit(e))
/**
* Returns the boundary of an H3 cell in WKT format.
*
* @group h3_funcs
* @since 3.3.0
*/
@Py4JWhitelist
def h3_boundaryaswkt(e: Column): Column =
Column.fn("h3_boundaryaswkt", lit(e))
/**
* Returns the boundary of an H3 cell in GeoJSON format.
*
* @group h3_funcs
* @since 3.3.0
*/
@Py4JWhitelist
def h3_boundaryasgeojson(e: Column): Column =
Column.fn("h3_boundaryasgeojson", lit(e))
/**
* Returns the boundary of an H3 cell in WKB format.
*
* @group h3_funcs
* @since 3.3.0
*/
@Py4JWhitelist
def h3_boundaryaswkb(e: Column): Column =
Column.fn("h3_boundaryaswkb", lit(e))
/**
* Returns the center of an H3 cell in WKT format.
*
* @group h3_funcs
* @since 3.3.0
*/
@Py4JWhitelist
def h3_centeraswkt(e: Column): Column =
Column.fn("h3_centeraswkt", lit(e))
/**
* Returns the center of an H3 cell in GeoJSON format.
*
* @group h3_funcs
* @since 3.3.0
*/
@Py4JWhitelist
def h3_centerasgeojson(e: Column): Column =
Column.fn("h3_centerasgeojson", lit(e))
/**
* Returns the center of an H3 cell in WKB format.
*
* @group h3_funcs
* @since 3.3.0
*/
@Py4JWhitelist
def h3_centeraswkb(e: Column): Column =
Column.fn("h3_centeraswkb", lit(e))
/**
* Returns an array of H3 cell IDs that form a hollow hexagonal ring centered at the origin H3
* cell and that are at grid distance k from the origin H3 cell.
*
* @group h3_funcs
* @since 3.3.0
*/
@Py4JWhitelist
def h3_hexring(e1: Column, e2: Column): Column =
Column.fn("h3_hexring", lit(e1), lit(e2))
/**
* Returns true if the first H3 cell ID is a child of the second H3 cell ID.
*
* @group h3_funcs
* @since 3.3.0
*/
@Py4JWhitelist
def h3_ischildof(e1: Column, e2: Column): Column =
Column.fn("h3_ischildof", lit(e1), lit(e2))
/**
* Returns an array of cell IDs represented as long integers, corresponding to hexagons or
* pentagons of the specified resolution that are contained by the input areal geography.
* Containment is determined by the cell centroids: a cell is considered to cover the geography if
* the cell's centroid lies inside the areal geography.
* The expression emits an error if the geography is not areal (polygon or multipolygon) or if an
* error is found when parsing the input representation of the geography.
* The acceptable input representations are WKT, GeoJSON, and WKB. In the first two cases the
* input is expected to be of type STRING, whereas in the last case the input is expected to be of
* type BINARY.
*
* @group h3_funcs
* @since 3.3.0
*/
@Py4JWhitelist
def h3_polyfillash3(e1: Column, e2: Column): Column =
Column.fn("h3_polyfillash3", lit(e1), lit(e2))
/**
* Returns an array of cell IDs represented as strings, corresponding to hexagons or pentagons
* of the specified resolution that are contained by the input areal geography.
* Containment is determined by the cell centroids: a cell is considered to cover the geography if
* the cell's centroid lies inside the areal geography.
* The expression emits an error if the geography is not areal (polygon or multipolygon) or if an
* error is found when parsing the input representation of the geography.
* The acceptable input representations are WKT, GeoJSON, and WKB. In the first two cases the
* input is expected to be of type STRING, whereas in the last case the input is expected to be of
* type BINARY.
*
* @group h3_funcs
* @since 3.3.0
*/
@Py4JWhitelist
def h3_polyfillash3string(e1: Column, e2: Column): Column =
Column.fn("h3_polyfillash3string", lit(e1), lit(e2))
/**
* Returns an array of cell IDs represented as long integers, corresponding to hexagons or
* pentagons of the specified resolution that are contained by the input areal geography.
* Containment is determined by the cell centroids: a cell is considered to cover the geography if
* the cell's centroid lies inside the areal geography.
* The expression's value is NULL if the geography is not areal (polygon or multipolygon) or if an
* error is found when parsing the input representation of the geography.
* The acceptable input representations are WKT, GeoJSON, and WKB. In the first two cases the
* input is expected to be of type STRING, whereas in the last case the input is expected to be of
* type BINARY.
*
* @group h3_funcs
* @since 3.3.0
*/
@Py4JWhitelist
def h3_try_polyfillash3(e1: Column, e2: Column): Column =
Column.fn("h3_try_polyfillash3", lit(e1), lit(e2))
/**
* Returns an array of cell IDs represented as strings, corresponding to hexagons or pentagons
* of the specified resolution that are contained by the input areal geography.
* Containment is determined by the cell centroids: a cell is considered to cover the geography if
* the cell's centroid lies inside the areal geography.
* The expression's value is NULL if the geography is not areal (polygon or multipolygon) or if an
* error is found when parsing the input representation of the geography.
* The acceptable input representations are WKT, GeoJSON, and WKB. In the first two cases the
* input is expected to be of type STRING, whereas in the last case the input is expected to be of
* type BINARY.
*
* @group h3_funcs
* @since 3.3.0
*/
@Py4JWhitelist
def h3_try_polyfillash3string(e1: Column, e2: Column): Column =
Column.fn("h3_try_polyfillash3string", lit(e1), lit(e2))
/**
* Returns all H3 cell IDs (represented as long integers or strings) within grid distance k from
* the origin H3 cell ID, along with their distance from the origin H3 cell ID.
* More precisely, the result is an array of structs, where each struct contains an H3 cell
* id (represented as a long integer or string) and its distance from the origin H3 cell ID.
* The type for the H3 cell IDs in the output is the same as the type of the input H3 cell ID
* (first argument of the expression).
*
* @group h3_funcs
* @since 3.3.0
*/
@Py4JWhitelist
def h3_kringdistances(e1: Column, e2: Column): Column =
Column.fn("h3_kringdistances", lit(e1), lit(e2))
/**
* Returns the child of minimum value of the input H3 cell at the specified resolution.
*
* @group h3_funcs
* @since 3.3.0
*/
@Py4JWhitelist
def h3_minchild(e1: Column, e2: Column): Column =
Column.fn("h3_minchild", lit(e1), lit(e2))
/**
* Returns the child of maximum value of the input H3 cell at the specified resolution.
*
* @group h3_funcs
* @since 3.3.0
*/
@Py4JWhitelist
def h3_maxchild(e1: Column, e2: Column): Column =
Column.fn("h3_maxchild", lit(e1), lit(e2))
/**
* Returns the H3 cell ID (as a BIGINT) corresponding to the provided point at the specified
* resolution.
* The expression emits an error if the geography is not a point or if an error is found when
* parsing the input representation of the geography.
* The acceptable input representations are WKT, GeoJSON, and WKB. In the first two cases the
* input is expected to be of type STRING, whereas in the last case the input is expected to be
* of type BINARY.
*
* @group h3_funcs
* @since 3.3.0
*/
@Py4JWhitelist
def h3_pointash3(e1: Column, e2: Column): Column =
Column.fn("h3_pointash3", lit(e1), lit(e2))
/**
* Returns the H3 cell ID (as a STRING) corresponding to the provided point at the specified
* resolution.
* The expression emits an error if the geography is not a point or if an error is found when
* parsing the input representation of the geography.
* The acceptable input representations are WKT, GeoJSON, and WKB. In the first two cases the
* input is expected to be of type STRING, whereas in the last case the input is expected to be
* of type BINARY.
*
* @group h3_funcs
* @since 3.3.0
*/
@Py4JWhitelist
def h3_pointash3string(e1: Column, e2: Column): Column =
Column.fn("h3_pointash3string", lit(e1), lit(e2))
/**
* Returns an array of cell IDs represented as long integers, corresponding to hexagons or
* pentagons of the specified resolution that minimally cover the input areal geography.
* The expression emits an error if the geography is not areal (polygon or multipolygon) or if an
* error is found when parsing the input representation of the geography.
* The acceptable input representations are WKT, GeoJSON, and WKB. In the first two cases the
* input is expected to be of type STRING, whereas in the last case the input is expected to be of
* type BINARY.
*
* @group h3_funcs
* @since 3.4.0
*/
@Py4JWhitelist
def h3_coverash3(e1: Column, e2: Column): Column =
Column.fn("h3_coverash3", lit(e1), lit(e2))
/**
* Returns an array of cell IDs represented as strings, corresponding to hexagons or pentagons
* of the specified resolution that minimally cover the input areal geography.
* The expression emits an error if the geography is not areal (polygon or multipolygon) or if an
* error is found when parsing the input representation of the geography.
* The acceptable input representations are WKT, GeoJSON, and WKB. In the first two cases the
* input is expected to be of type STRING, whereas in the last case the input is expected to be of
* type BINARY.
*
* @group h3_funcs
* @since 3.4.0
*/
@Py4JWhitelist
def h3_coverash3string(e1: Column, e2: Column): Column =
Column.fn("h3_coverash3string", lit(e1), lit(e2))
/**
* Returns an array of structs representing the chips covering geography at the specified
* resolution.
* More precisely, the elements of the returned array are named structs with three fields
* names "cellid", "core", and "chip":
* - The "cellid" field contains one of the H3 cells covering the input geography.
* - The "core" field determines if the boundary polygon of the H3 cell in the "cellid" field
* is contained inside the input geography (this can happen only for areal input geographies).
* If the cell is contained in the input geography the value is set to `true`, and `false`
* otherwise.
* - The "chip" field is the (Cartesian) intersection of the H3 cell polygon, corresponding to the
* H3 cell id in the "cellid" field, with the input geography, represented in WKB format.
* The set of the H3 cells ids returned as the "cellid" elements of the structs in the array is a
* minimal covering set for the input geography.
*
* @group h3_funcs
* @since 3.5.0
*/
def h3_tessellateaswkb(geo: Column, res: Column): Column =
Column.fn("h3_tessellateaswkb", lit(geo), lit(res))
def h3_tessellateaswkb(geo: Column, res: Int): Column =
Column.fn("h3_tessellateaswkb", lit(geo), lit(res))
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy