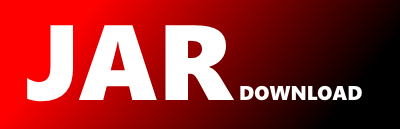
com.databricks.sql.stFunctions.scala Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of databricks-connect Show documentation
Show all versions of databricks-connect Show documentation
Develop locally and connect IDEs, notebook servers and running applications to Databricks clusters.
The newest version!
//
// DATABRICKS CONFIDENTIAL & PROPRIETARY
// __________________
//
// Copyright 2024-present Databricks, Inc.
// All Rights Reserved.
//
// NOTICE: All information contained herein is, and remains the property of Databricks, Inc.
// and its suppliers, if any. The intellectual and technical concepts contained herein are
// proprietary to Databricks, Inc. and its suppliers and may be covered by U.S. and foreign Patents,
// patents in process, and are protected by trade secret and/or copyright law. Dissemination, use,
// or reproduction of this information is strictly forbidden unless prior written permission is
// obtained from Databricks, Inc.
//
// If you view or obtain a copy of this information and believe Databricks, Inc. may not have
// intended it to be made available, please promptly report it to Databricks Legal Department
// @ [email protected].
//
package org.apache.spark.sql
import org.apache.spark.annotation.{Py4JWhitelist, Stable}
import org.apache.spark.sql.Column
import org.apache.spark.sql.functions.lit
@Py4JWhitelist
@Stable
/**
* Scala ST functions
*
* @groupname st_funcs ST geospatial functions
*/
// scalastyle:off: object.name
trait stFunctions {
// scalastyle:on
/**
* Returns the area of the input BINARY, GEOGRAPHY, GEOMETRY, or STRING value.
*
* @group st_funcs
* @since 3.5.0
*/
def st_area(geo: Column): Column =
Column.fn("st_area", lit(geo))
/**
* Returns the input GEOGRAPHY or GEOMETRY value in little endian WKB format.
*
* @group st_funcs
* @since 3.5.0
*/
def st_asbinary(geo: Column): Column =
Column.fn("st_asbinary", lit(geo))
/**
* Returns the input GEOGRAPHY or GEOMETRY value in WKB format using the specified endianness.
*
* @group st_funcs
* @since 3.5.0
*/
def st_asbinary(geo: Column, endianness: Column): Column =
Column.fn("st_asbinary", lit(geo), lit(endianness))
def st_asbinary(geo: Column, endianness: String): Column =
Column.fn("st_asbinary", lit(geo), lit(endianness))
/**
* Returns the input GEOMETRY value in little endian EWKB format.
*
* @group st_funcs
* @since 3.5.0
*/
def st_asewkb(geo: Column): Column =
Column.fn("st_asewkb", lit(geo))
/**
* Returns the input GEOMETRY value in EWKB format using the specified endianness.
*
* @group st_funcs
* @since 3.5.0
*/
def st_asewkb(geo: Column, endianness: Column): Column =
Column.fn("st_asewkb", lit(geo), lit(endianness))
def st_asewkb(geo: Column, endianness: String): Column =
Column.fn("st_asewkb", lit(geo), lit(endianness))
/**
* Returns the input GEOGRAPHY or GEOMETRY value in GeoJSON format using a precision equal to 15.
*
* @group st_funcs
* @since 3.5.0
*/
def st_asgeojson(geo: Column): Column =
Column.fn("st_asgeojson", lit(geo))
/**
* Returns the input GEOGRAPHY or GEOMETRY value in GeoJSON format using the specified precision.
*
* @group st_funcs
* @since 3.5.0
*/
def st_asgeojson(geo: Column, precision: Column): Column =
Column.fn("st_asgeojson", lit(geo), lit(precision))
def st_asgeojson(geo: Column, precision: Int): Column =
Column.fn("st_asgeojson", lit(geo), lit(precision))
/**
* Returns the input GEOGRAPHY or GEOMETRY value in WKT format using a precision equal to 15.
*
* @group st_funcs
* @since 3.5.0
*/
def st_astext(geo: Column): Column =
Column.fn("st_astext", lit(geo))
/**
* Returns the input GEOGRAPHY or GEOMETRY value in WKT format using the specified precision.
*
* @group st_funcs
* @since 3.5.0
*/
def st_astext(geo: Column, precision: Column): Column =
Column.fn("st_astext", lit(geo), lit(precision))
def st_astext(geo: Column, precision: Int): Column =
Column.fn("st_astext", lit(geo), lit(precision))
/**
* Returns the input GEOGRAPHY or GEOMETRY value in EWKT format using a precision equal to 15.
*
* @group st_funcs
* @since 4.0.0
*/
def st_asewkt(geo: Column): Column =
Column.fn("st_asewkt", lit(geo))
/**
* Returns the input GEOGRAPHY or GEOMETRY value in EWKT format using the specified precision.
*
* @group st_funcs
* @since 4.0.0
*/
def st_asewkt(geo: Column, precision: Column): Column =
Column.fn("st_asewkt", lit(geo), lit(precision))
def st_asewkt(geo: Column, precision: Int): Column =
Column.fn("st_asewkt", lit(geo), lit(precision))
/**
* Returns the buffer of the input geometry using the specified radius.
*
* @group st_funcs
* @since 3.5.0
*/
def st_buffer(geo: Column, radius: Column): Column =
Column.fn("st_buffer", lit(geo), lit(radius))
/**
* Returns the centroid of the input geometry as a 2D point geometry.
*
* @group st_funcs
* @since 3.5.0
*/
def st_centroid(geo: Column): Column =
Column.fn("st_centroid", lit(geo))
/**
* Returns `true` if the first geometry contains the second geometry.
*
* @group st_funcs
* @since 3.5.0
*/
def st_contains(geo1: Column, geo2: Column): Column =
Column.fn("st_contains", lit(geo1), lit(geo2))
/**
* Returns the convex hull of the input geometry as a geometry.
*
* @group st_funcs
* @since 3.5.0
*/
def st_convexhull(geo: Column): Column =
Column.fn("st_convexhull", lit(geo))
/**
* Returns the point-set different of the two input geometries as a 2D geometry.
*
* @group st_funcs
* @since 3.5.0
*/
def st_difference(geo1: Column, geo2: Column): Column =
Column.fn("st_difference", lit(geo1), lit(geo2))
/**
* Returns the topological dimension of the 2D projection of the input geometry.
*
* @group st_funcs
* @since 4.0.0
*/
def st_dimension(geo: Column): Column =
Column.fn("st_dimension", lit(geo))
/**
* Returns the 2D Cartesian distance between the two input geometries. The units of the result are
* those of the coordinates of the input geometries.
*
* @group st_funcs
* @since 3.5.0
*/
def st_distance(geo1: Column, geo2: Column): Column =
Column.fn("st_distance", lit(geo1), lit(geo2))
/**
* Returns the spherical distance (in meters) between two point geometries, measured on a sphere
* whose radius is the mean radius of the WGS84 ellipsoid.
*
* @group st_funcs
* @since 3.5.0
*/
def st_distancesphere(geo1: Column, geo2: Column): Column =
Column.fn("st_distancesphere", lit(geo1), lit(geo2))
/**
* Returns the geodesic distance (in meters) between two point geometries on the WGS84 ellipsoid.
* The coordinates of the points are assumed to be in degrees.
*
* @group st_funcs
* @since 3.5.0
*/
def st_distancespheroid(geo1: Column, geo2: Column): Column =
Column.fn("st_distancespheroid", lit(geo1), lit(geo2))
/**
* Returns the last point of the input GEOGRAPHY or GEOMETRY value, if the input geospatial
* value is a non-empty linestring. Otherwise, returns NULL. The SRID value of the output point
* geography or geometry is the same as that of the input value.
*
* @group st_funcs
* @since 4.0.0
*/
def st_endpoint(geo: Column): Column =
Column.fn("st_endpoint", lit(geo))
/**
* Returns a 2D Cartesian geometry representing the 2D axis-aligned minimum bounding box
* (envelope) of the input geometry, if one exists. In the case the input geometry is empty
* (that is it has zero non-empty points) it is returned as is.
*
* @group st_funcs
* @since 3.5.0
*/
def st_envelope(geo: Column): Column =
Column.fn("st_envelope", lit(geo))
/**
* Swaps the X and Y coordinates of the input geometry.
*
* @group st_funcs
* @since 4.0.0
*/
def st_flipcoordinates(geo: Column): Column =
Column.fn("st_flipcoordinates", lit(geo))
/**
* Returns the 2D geodesic area of the input BINARY or STRING value representing a geography.
* The result is in square meters.
*
* @group st_funcs
* @since 3.5.0
*/
def st_geogarea(e: Column): Column =
Column.fn("st_geogarea", lit(e))
/**
* Parses the GeoJSON description of a geography and returns the corresponding GEOGRAPHY value.
* The SRID value of the returned GEOGRAPHY value is 4326.
*
* @group st_funcs
* @since 3.5.0
*/
def st_geogfromgeojson(geojson: Column): Column =
Column.fn("st_geogfromgeojson", lit(geojson))
/**
* Parses the WKT description of a geography and returns the corresponding GEOGRAPHY value. The
* SRID value of the returned GEOGRAPHY value is 4326.
*
* @group st_funcs
* @since 3.5.0
*/
def st_geogfromtext(wkt: Column): Column =
Column.fn("st_geogfromtext", lit(wkt))
/**
* Parses the WKB description of a geography and returns the corresponding GEOGRAPHY value. The
* SRID value of the returned GEOGRAPHY value is 4326.
*
* @group st_funcs
* @since 3.5.0
*/
def st_geogfromwkb(wkb: Column): Column =
Column.fn("st_geogfromwkb", lit(wkb))
/**
* Returns the geodesic length of the input BINARY or STRING value representing a geography.
* The result is in meters.
*
* @group st_funcs
* @since 3.5.0
*/
def st_geoglength(e: Column): Column =
Column.fn("st_geoglength", lit(e))
/**
* Returns the geodesic perimeter of the input BINARY or STRING value representing a geography.
* The result is in meters
*
* @group st_funcs
* @since 3.5.0
*/
def st_geogperimeter(e: Column): Column =
Column.fn("st_geogperimeter", lit(e))
/**
* Returns the geohash corresponding to the input geometry and the precision, or NULL if the
* geometry is empty.
*
* @group st_funcs
* @since 3.5.0
*/
def st_geohash(geo: Column, precision: Column): Column =
Column.fn("st_geohash", lit(geo), lit(precision))
def st_geohash(geo: Column, precision: Int): Column =
Column.fn("st_geohash", lit(geo), lit(precision))
/**
* Returns the geohash corresponding to the input geometry, or NULL if the geometry is empty.
* The precision of the returned geohash value is at most 12.
*
* @group st_funcs
* @since 3.5.0
*/
def st_geohash(geo: Column): Column =
Column.fn("st_geohash", lit(geo))
/**
* Returns the 1-based n-th element of the input geometry as a GEOMETRY value, if the input is a
* multipoint, a multilinestring, a multipolygon, or a geometry collection, or NULL if the element
* does not exist. Returns the input as a GEOMETRY value, if the input is a non-empty point,
* linestring, or polygon and the value of the index is equal to 1, otherwise returns NULL.
*
* @group st_funcs
* @since 3.5.0
*/
def st_geometryn(geo: Column, n: Column): Column =
Column.fn("st_geometryn", lit(geo), lit(n))
def st_geometryn(geo: Column, n: Int): Column =
Column.fn("st_geometryn", lit(geo), lit(n))
/**
* Returns the type of the input GEOGRAPHY or GEOMETRY value as a string.
*
* @group st_funcs
* @since 3.5.0
*/
def st_geometrytype(geo: Column): Column =
Column.fn("st_geometrytype", lit(geo))
/**
* Parses the EWKB description of a geometry and returns the corresponding GEOMETRY value. The
* SRID value of the returned GEOMETRY value is the one prescribed in the EWKB description, or
* 0 if there is no SRID value in the EWKB description.
*
* @group st_funcs
* @since 3.5.0
*/
def st_geomfromewkb(ewkb: Column): Column =
Column.fn("st_geomfromewkb", lit(ewkb))
/**
* Returns the geohash grid box corresponding to the input geohash value as a 2D polygon geometry.
*
* @group st_funcs
* @since 3.5.0
*/
def st_geomfromgeohash(geohash: Column): Column =
Column.fn("st_geomfromgeohash", lit(geohash))
/**
* Parses the GeoJSON description of a geometry and returns the corresponding GEOMETRY value. The
* SRID value of the returned GEOMETRY value is 4326.
*
* @group st_funcs
* @since 3.5.0
*/
def st_geomfromgeojson(geojson: Column): Column =
Column.fn("st_geomfromgeojson", lit(geojson))
/**
* Parses the WKT description of a geometry and returns the corresponding GEOMETRY value. The SRID
* value of the returned GEOMETRY value is 0.
*
* @group st_funcs
* @since 3.5.0
*/
def st_geomfromtext(wkt: Column): Column =
Column.fn("st_geomfromtext", lit(wkt))
/**
* Parses the WKT description of a geometry and returns the corresponding GEOMETRY value. The SRID
* value of the returned GEOMETRY value is the provided SRID.
*
* @group st_funcs
* @since 3.5.0
*/
def st_geomfromtext(wkt: Column, srid: Column): Column =
Column.fn("st_geomfromtext", lit(wkt), lit(srid))
def st_geomfromtext(wkt: Column, srid: Int): Column =
Column.fn("st_geomfromtext", lit(wkt), lit(srid))
/**
* Parses the WKB description of a geometry and returns the corresponding GEOMETRY value. The SRID
* value of the returned GEOGRAPHY value is 0.
*
* @group st_funcs
* @since 3.5.0
*/
def st_geomfromwkb(wkb: Column): Column =
Column.fn("st_geomfromwkb", lit(wkb))
/**
* Parses the WKB description of a geometry and returns the corresponding GEOMETRY value. The SRID
* value of the returned GEOGRAPHY value is the provided SRID.
*
* @group st_funcs
* @since 3.5.0
*/
def st_geomfromwkb(wkb: Column, srid: Column): Column =
Column.fn("st_geomfromwkb", lit(wkb), lit(srid))
def st_geomfromwkb(wkb: Column, srid: Int): Column =
Column.fn("st_geomfromwkb", lit(wkb), lit(srid))
/**
* Returns the point-set intersection of the two input geometries as a 2D geometry.
*
* @group st_funcs
* @since 3.5.0
*/
def st_intersection(geo1: Column, geo2: Column): Column =
Column.fn("st_intersection", lit(geo1), lit(geo2))
/**
* Returns `true` if the two geometries intersect.
*
* @group st_funcs
* @since 3.5.0
*/
def st_intersects(geo1: Column, geo2: Column): Column =
Column.fn("st_intersects", lit(geo1), lit(geo2))
/**
* Returns true if the input geography or geometry value does not contain any non-empty points.
*
* @group st_funcs
* @since 3.5.0
*/
def st_isempty(geo: Column): Column =
Column.fn("st_isempty", lit(geo))
/**
* Returns true if the input geometry is a valid geometry in the OGC sense.
* See: go/geometric-validity for details of what geometric validity means in the OGC sense.
*
* @group st_funcs
* @since 3.5.0
*/
def st_isvalid(geo: Column): Column =
Column.fn("st_isvalid", lit(geo))
/**
* Returns the length of the input BINARY, GEOGRAPHY, GEOMETRY, or STRING value.
*
* @group st_funcs
* @since 3.5.0
*/
def st_length(e: Column): Column =
Column.fn("st_length", lit(e))
/**
* Returns a linestring geometry whose points are the non-empty points of the geometries in the
* input array of geometries, which are expected to be points, linestrings, or multipoints.
*
* @group st_funcs
* @since 3.5.0
*/
def st_makeline(geoArray: Column): Column =
Column.fn("st_makeline", lit(geoArray))
/**
* Constructs a polygon from the input outer boundary, represented as a closed linestring.
*
* @group st_funcs
* @since 3.5.0
*/
def st_makepolygon(geo: Column): Column =
Column.fn("st_makepolygon", lit(geo))
/**
* Constructs a polygon from the input outer boundary and array of inner boundaries, represented
* as closed linestrings.
*
* @group st_funcs
* @since 3.5.0
*/
def st_makepolygon(geo: Column, geoArray: Column): Column =
Column.fn("st_makepolygon", lit(geo), lit(geoArray))
/**
* Returns the geometry as a MULTI* geometry collection. If the geometry is already a collection,
* it is returned unchanged.
*
* @group st_funcs
* @since 4.0.0
*/
def st_multi(geo: Column): Column =
Column.fn("st_multi", lit(geo))
/**
* Returns the coordinate dimension (that is the number of coordinates of the points) of the input
* geography or geometry value.
*
* @group st_funcs
* @since 3.5.0
*/
def st_ndims(geo: Column): Column =
Column.fn("st_ndims", lit(geo))
/**
* Returns the number of non-empty points in the input geometry or geography value.
*
* @group st_funcs
* @since 3.5.0
*/
def st_npoints(geo: Column): Column =
Column.fn("st_npoints", lit(geo))
/**
* Returns the number of geometries in the input geometry.
*
* @group st_funcs
* @since 3.5.0
*/
def st_numgeometries(geo: Column): Column =
Column.fn("st_numgeometries", lit(geo))
/**
* Returns the perimeter of the input BINARY, GEOGRAPHY, GEOMETRY, or STRING value.
*
* @group st_funcs
* @since 3.5.0
*/
def st_perimeter(e: Column): Column =
Column.fn("st_perimeter", lit(e))
/**
* Returns a 2D point GEOMETRY with the given x and y coordinates and SRID value. The SRID value
* of the returned point geometry is set to 0.
*
* @group st_funcs
* @since 3.5.0
*/
def st_point(x: Column, y: Column): Column =
Column.fn("st_point", lit(x), lit(y))
/**
* Returns a 2D point GEOMETRY with the given x and y coordinates and SRID value.
*
* @group st_funcs
* @since 3.5.0
*/
def st_point(x: Column, y: Column, srid: Column): Column =
Column.fn("st_point", lit(x), lit(y), lit(srid))
def st_point(x: Column, y: Column, srid: Int): Column =
Column.fn("st_point", lit(x), lit(y), lit(srid))
/**
* Returns the center of the geohash grid box corresponding to the input geohash value as a 2D
* point geometry.
*
* @group st_funcs
* @since 3.5.0
*/
def st_pointfromgeohash(geohash: Column): Column =
Column.fn("st_pointfromgeohash", lit(geohash))
/**
* Returns the 1-based indexed n-th point of the input GEOGRAPHY or GEOMETRY value, if the index
* is valid and the input geospatial value is a non-empty linestring. Otherwise, returns NULL. The
* SRID value of the output point geography or geometry is the same as that of the input value.
*
* @group st_funcs
* @since 4.0.0
*/
def st_pointn(geo: Column, n: Column): Column =
Column.fn("st_pointn", lit(geo), lit(n))
def st_pointn(geo: Column, n: Int): Column =
Column.fn("st_pointn", lit(geo), lit(n))
/**
* Rotates the input geometry around the Z axis by the given rotation angle (in radians).
*
* @group st_funcs
* @since 3.5.0
*/
def st_rotate(geo: Column, angle: Column): Column =
Column.fn("st_rotate", lit(geo), lit(angle))
def st_rotate(geo: Column, angle: Double): Column =
Column.fn("st_rotate", lit(geo), lit(angle))
/**
* Scales the input geometry using in the X and Y directions using the provided scaling factors.
*
* @group st_funcs
* @since 3.5.0
*/
def st_scale(geo: Column, xFactor: Column, yFactor: Column): Column =
Column.fn("st_scale", lit(geo), lit(xFactor), lit(yFactor))
def st_scale(geo: Column, xFactor: Double, yFactor: Double): Column =
Column.fn("st_scale", lit(geo), lit(xFactor), lit(yFactor))
/**
* Scales the input geometry using in the X, Y, and Z directions using the provided scaling
* factors.
*
* @group st_funcs
* @since 3.5.0
*/
def st_scale(geo: Column, xFactor: Column, yFactor: Column, zFactor: Column): Column =
Column.fn("st_scale", lit(geo), lit(xFactor), lit(yFactor), lit(zFactor))
def st_scale(geo: Column, xFactor: Double, yFactor: Double, zFactor: Double): Column =
Column.fn("st_scale", lit(geo), lit(xFactor), lit(yFactor), lit(zFactor))
/**
* Returns a new GEOMETRY value whose SRID is the specified SRID value.
*
* @group st_funcs
* @since 3.5.0
*/
def st_setsrid(geo: Column, newSrid: Column): Column =
Column.fn("st_setsrid", lit(geo), lit(newSrid))
def st_setsrid(geo: Column, newSrid: Int): Column =
Column.fn("st_setsrid", lit(geo), lit(newSrid))
/**
* Simplifies the input geometry using the Douglas-Peucker algorithm (see:
* https://en.wikipedia.org/wiki/Ramer%E2%80%93Douglas%E2%80%93Peucker_algorithm)
*
* @group st_funcs
* @since 3.5.0
*/
def st_simplify(geo: Column, threshold: Column): Column =
Column.fn("st_simplify", lit(geo), lit(threshold))
def st_simplify(geo: Column, threshold: Double): Column =
Column.fn("st_simplify", lit(geo), lit(threshold))
/**
* Returns the SRID of the input GEOGRAPHY or GEOMETRY value.
*
* @group st_funcs
* @since 3.5.0
*/
def st_srid(geo: Column): Column =
Column.fn("st_srid", lit(geo))
/**
* Returns the first point of the input GEOGRAPHY or GEOMETRY value, if the input geospatial
* value is a non-empty linestring. Otherwise, returns NULL. The SRID value of the output point
* geography or geometry is the same as that of the input value.
*
* @group st_funcs
* @since 4.0.0
*/
def st_startpoint(geo: Column): Column =
Column.fn("st_startpoint", lit(geo))
/**
* Transforms the X and Y coordinates of the input geometry from the current coordinate reference
* system (CRS) to the coordinate reference system described by the provided SRID value.
*
* @group st_funcs
* @since 3.5.0
*/
def st_transform(geo: Column, srid: Column): Column =
Column.fn("st_transform", lit(geo), lit(srid))
def st_transform(geo: Column, srid: Int): Column =
Column.fn("st_transform", lit(geo), lit(srid))
/**
* Translates the input geometry using in the X and Y directions using the provided offsets.
*
* @group st_funcs
* @since 3.5.0
*/
def st_translate(geo: Column, xOffset: Column, yOffset: Column): Column =
Column.fn("st_translate", lit(geo), lit(xOffset), lit(yOffset))
def st_translate(geo: Column, xOffset: Double, yOffset: Double): Column =
Column.fn("st_translate", lit(geo), lit(xOffset), lit(yOffset))
/**
* Translates the input geometry using in the X, Y, and Z directions using the provided offsets.
*
* @group st_funcs
* @since 3.5.0
*/
def st_translate(geo: Column, xOffset: Column, yOffset: Column, zOffset: Column): Column =
Column.fn("st_translate", lit(geo), lit(xOffset), lit(yOffset), lit(zOffset))
def st_translate(geo: Column, xOffset: Double, yOffset: Double, zOffset: Double): Column =
Column.fn("st_translate", lit(geo), lit(xOffset), lit(yOffset), lit(zOffset))
/**
* Returns the point-set union of the two input geometries as a 2D geometry.
*
* @group st_funcs
* @since 3.5.0
*/
def st_union(geo1: Column, geo2: Column): Column =
Column.fn("st_union", lit(geo1), lit(geo2))
/**
*
* @group st_funcs
* @since 3.5.0
*/
def st_union_agg(geo: Column): Column = {
Column.fn("st_union_agg", lit(geo))
}
/**
* Returns the X coordinate of the input point geometry, or NULL if the point is empty.
*
* @group st_funcs
* @since 3.5.0
*/
def st_x(geo: Column): Column =
Column.fn("st_x", lit(geo))
/**
* Returns the maximum X coordinate of the input geometry, or NULL if the geometry is empty.
*
* @group st_funcs
* @since 3.5.0
*/
def st_xmax(geo: Column): Column =
Column.fn("st_xmax", lit(geo))
/**
* Returns the minimum X coordinate of the input geometry, or NULL if the geometry is empty.
*
* @group st_funcs
* @since 3.5.0
*/
def st_xmin(geo: Column): Column =
Column.fn("st_xmin", lit(geo))
/**
* Returns the Y coordinate of the input point geometry, or NULL if the point is empty.
*
* @group st_funcs
* @since 3.5.0
*/
def st_y(geo: Column): Column =
Column.fn("st_y", lit(geo))
/**
* Returns the maximum Y coordinate of the input geometry, or NULL if the geometry is empty.
*
* @group st_funcs
* @since 3.5.0
*/
def st_ymax(geo: Column): Column =
Column.fn("st_ymax", lit(geo))
/**
* Returns the minimum Y coordinate of the input geometry, or NULL if the geometry is empty.
*
* @group st_funcs
* @since 3.5.0
*/
def st_ymin(geo: Column): Column =
Column.fn("st_ymin", lit(geo))
/**
* Returns the Z coordinate of the input point geometry, or NULL if the point is empty or
* does not have a Z coordinate.
*
* @group st_funcs
* @since 4.0.0
*/
def st_z(geo: Column): Column =
Column.fn("st_z", lit(geo))
/**
* Returns the maximum Z coordinate of the input geometry, or NULL if the geometry is empty or
* does not have a Z coordinate.
*
* @group st_funcs
* @since 3.5.0
*/
def st_zmax(geo: Column): Column =
Column.fn("st_zmax", lit(geo))
/**
* Returns the minimum Z coordinate of the input geometry, or NULL if the geometry is empty or
* does not have a Z coordinate.
*
* @group st_funcs
* @since 3.5.0
*/
def st_zmin(geo: Column): Column =
Column.fn("st_zmin", lit(geo))
/**
* Returns the M coordinate of the input point geometry, or NULL if the point is empty or
* does not have an M coordinate.
*
* @group st_funcs
* @since 4.0.0
*/
def st_m(geo: Column): Column =
Column.fn("st_m", lit(geo))
/**
* Parses the input BINARY or STRING value and returns the corresponding GEOGRAPHY value.
* The input expected to be one of the standard geospatial formats: GeoJSON (STRING input value),
* WKB (BINARY input value), or WKT(STRING input value). The SRID value of the returned GEOGRAPHY
* value is 4326.
*
* @group st_funcs
* @since 3.5.0
*/
def to_geography(geoRep: Column): Column =
Column.fn("to_geography", lit(geoRep))
/**
* Parses the input BINARY or STRING value and returns the corresponding GEOMETRY value.
* The input expected to be one of the standard geospatial formats: GeoJSON (STRING input value),
* WKB (BINARY input value), or WKT(STRING input value). The SRID value of the returned GEOMETRY
* value is 4326 if the input is GeoJSON, and 0 otherwise.
*
* @group st_funcs
* @since 3.5.0
*/
def to_geometry(geoRep: Column): Column =
Column.fn("to_geometry", lit(geoRep))
/**
* Parses the input BINARY or STRING value and returns the corresponding GEOGRAPHY value.
* The input expected to be one of the standard geospatial formats: GeoJSON (STRING input value),
* WKB (BINARY input value), or WKT(STRING input value). The SRID value of the returned GEOGRAPHY
* value is 4326. NULL is returned if the input is invalid.
*
* @group st_funcs
* @since 3.5.0
*/
def try_to_geography(geoRep: Column): Column =
Column.fn("try_to_geography", lit(geoRep))
/**
* Parses the input BINARY or STRING value and returns the corresponding GEOMETRY value.
* The input expected to be one of the standard geospatial formats: GeoJSON (STRING input value),
* WKB (BINARY input value), or WKT(STRING input value). The SRID value of the returned GEOMETRY
* value is 4326 if the input is GeoJSON, and 0 otherwise. NULL is returned if the input is
* invalid.
*
* @group st_funcs
* @since 3.5.0
*/
def try_to_geometry(geoRep: Column): Column =
Column.fn("try_to_geometry", lit(geoRep))
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy