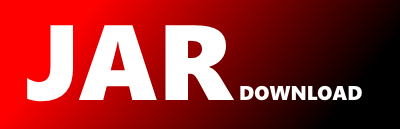
com.databricks.sdk.core.BodyLogger Maven / Gradle / Ivy
package com.databricks.sdk.core;
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.core.TreeNode;
import com.fasterxml.jackson.databind.JsonNode;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.databind.node.ArrayNode;
import java.util.*;
class BodyLogger {
private final Set redactKeys =
new HashSet() {
{
add("string_value");
add("token_value");
add("content");
}
};
private int maxBytes = 1023;
private int debugTruncateBytes = 96;
private final ObjectMapper mapper;
public BodyLogger(ObjectMapper mapper, int maxBytes, int debugTruncateBytes) {
this.mapper = mapper;
if (maxBytes == 0) {
maxBytes = 1024;
}
if (debugTruncateBytes > maxBytes) {
maxBytes = debugTruncateBytes;
}
this.maxBytes = maxBytes;
this.debugTruncateBytes = debugTruncateBytes;
}
private List mapKeys(TreeNode node) {
List keys = new ArrayList<>();
node.fieldNames().forEachRemaining(keys::add);
Collections.sort(keys);
return keys;
}
public String redactedDump(String body) {
if (body == null || body.isEmpty()) {
return "";
}
try {
JsonNode rootNode = mapper.readTree(body);
Object result = recursiveMarshal(rootNode, maxBytes);
return mapper.writerWithDefaultPrettyPrinter().writeValueAsString(result);
} catch (JsonProcessingException e) {
// Unable to unmarshal means the body isn't JSON
return onlyNBytes(body, maxBytes);
}
}
private Object recursiveMarshal(JsonNode node, int budget) {
if (node.isObject()) {
return recursiveMarshalObject(node, budget);
}
if (node.isArray()) {
return recursiveMarshalArray((ArrayNode) node, budget);
}
if (node.isTextual()) {
return onlyNBytes(node.asText(), debugTruncateBytes);
}
if (node.isNumber()) {
return node.asLong();
}
if (node.isDouble()) {
return node.asDouble();
}
if (node.isBoolean()) {
return node.asBoolean();
}
return node.asToken().asString();
}
private List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy