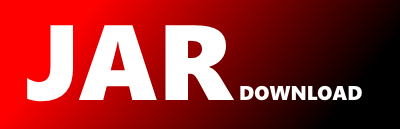
com.databricks.sdk.core.GrpcTranscodingQueryParamsSerializer Maven / Gradle / Ivy
package com.databricks.sdk.core;
import com.databricks.sdk.support.QueryParam;
import com.fasterxml.jackson.annotation.JsonProperty;
import java.lang.reflect.Field;
import java.util.*;
/**
* Serializes an object into a list of query parameter entries compatible with gRPC-transcoding.
*
* The Databricks REST API uses gRPC transcoding to expose gRPC services as REST APIs. This
* serializer is used to serialize objects into a map of query parameter entries that can be used to
* invoke a gRPC service via REST.
*
*
See the
* documentation for gRPC transcoding for more details.
*/
public class GrpcTranscodingQueryParamsSerializer {
public static class QueryParamPair {
private final String key;
private final String value;
public QueryParamPair(String key, String value) {
this.key = key;
this.value = value;
}
public String getKey() {
return key;
}
public String getValue() {
return value;
}
}
/**
* Serializes an object into a map of query parameter values compatible with gRPC-transcoding.
*
*
This method respects the QueryParam and JsonProperty annotations on the object's fields when
* serializing the field name. If both annotations are present, the value of the QueryParam
* annotation is used.
*
*
The returned object does not contain any top-level fields that are not annotated with
* QueryParam. All nested fields are included, even if they are not annotated with QueryParam.
*
* @param o The object to serialize.
* @return A list of query parameter entries compatible with gRPC-transcoding.
*/
public static List serialize(Object o) {
Map flattened = flattenObject(o, true);
List result = new ArrayList<>();
for (Map.Entry entry : flattened.entrySet()) {
String key = entry.getKey();
Object value = entry.getValue();
if (value instanceof Collection) {
for (Object v : (Collection