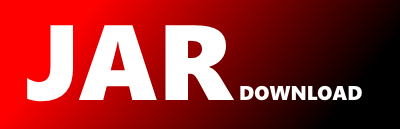
com.databricks.sdk.service.settings.AccountIpAccessListsService Maven / Gradle / Ivy
// Code generated from OpenAPI specs by Databricks SDK Generator. DO NOT EDIT.
package com.databricks.sdk.service.settings;
import com.databricks.sdk.support.Generated;
/**
* The Accounts IP Access List API enables account admins to configure IP access lists for access to
* the account console.
*
* Account IP Access Lists affect web application access and REST API access to the account
* console and account APIs. If the feature is disabled for the account, all access is allowed for
* this account. There is support for allow lists (inclusion) and block lists (exclusion).
*
*
When a connection is attempted: 1. **First, all block lists are checked.** If the connection
* IP address matches any block list, the connection is rejected. 2. **If the connection was not
* rejected by block lists**, the IP address is compared with the allow lists.
*
*
If there is at least one allow list for the account, the connection is allowed only if the IP
* address matches an allow list. If there are no allow lists for the account, all IP addresses are
* allowed.
*
*
For all allow lists and block lists combined, the account supports a maximum of 1000 IP/CIDR
* values, where one CIDR counts as a single value.
*
*
After changes to the account-level IP access lists, it can take a few minutes for changes to
* take effect.
*
*
This is the high-level interface, that contains generated methods.
*
*
Evolving: this interface is under development. Method signatures may change.
*/
@Generated
public interface AccountIpAccessListsService {
/**
* Create access list.
*
*
Creates an IP access list for the account.
*
*
A list can be an allow list or a block list. See the top of this file for a description of
* how the server treats allow lists and block lists at runtime.
*
*
When creating or updating an IP access list:
*
*
* For all allow lists and block lists combined, the API supports a maximum of 1000 IP/CIDR
* values, where one CIDR counts as a single value. Attempts to exceed that number return error
* 400 with `error_code` value `QUOTA_EXCEEDED`. * If the new list would block the calling user's
* current IP, error 400 is returned with `error_code` value `INVALID_STATE`.
*
*
It can take a few minutes for the changes to take effect.
*/
CreateIpAccessListResponse create(CreateIpAccessList createIpAccessList);
/**
* Delete access list.
*
*
Deletes an IP access list, specified by its list ID.
*/
void delete(DeleteAccountIpAccessListRequest deleteAccountIpAccessListRequest);
/**
* Get IP access list.
*
*
Gets an IP access list, specified by its list ID.
*/
GetIpAccessListResponse get(GetAccountIpAccessListRequest getAccountIpAccessListRequest);
/**
* Get access lists.
*
*
Gets all IP access lists for the specified account.
*/
GetIpAccessListsResponse list();
/**
* Replace access list.
*
*
Replaces an IP access list, specified by its ID.
*
*
A list can include allow lists and block lists. See the top of this file for a description
* of how the server treats allow lists and block lists at run time. When replacing an IP access
* list: * For all allow lists and block lists combined, the API supports a maximum of 1000
* IP/CIDR values, where one CIDR counts as a single value. Attempts to exceed that number return
* error 400 with `error_code` value `QUOTA_EXCEEDED`. * If the resulting list would block the
* calling user's current IP, error 400 is returned with `error_code` value `INVALID_STATE`. It
* can take a few minutes for the changes to take effect.
*/
void replace(ReplaceIpAccessList replaceIpAccessList);
/**
* Update access list.
*
*
Updates an existing IP access list, specified by its ID.
*
*
A list can include allow lists and block lists. See the top of this file for a description
* of how the server treats allow lists and block lists at run time.
*
*
When updating an IP access list:
*
*
* For all allow lists and block lists combined, the API supports a maximum of 1000 IP/CIDR
* values, where one CIDR counts as a single value. Attempts to exceed that number return error
* 400 with `error_code` value `QUOTA_EXCEEDED`. * If the updated list would block the calling
* user's current IP, error 400 is returned with `error_code` value `INVALID_STATE`.
*
*
It can take a few minutes for the changes to take effect.
*/
void update(UpdateIpAccessList updateIpAccessList);
}