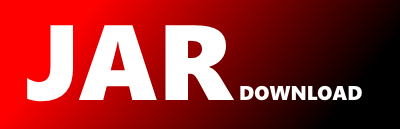
com.datadog.api.client.v1.api.ServiceLevelObjectivesApi Maven / Gradle / Ivy
package com.datadog.api.client.v1.api;
import com.datadog.api.client.ApiClient;
import com.datadog.api.client.ApiException;
import com.datadog.api.client.ApiResponse;
import com.datadog.api.client.PaginationIterable;
import com.datadog.api.client.Pair;
import com.datadog.api.client.v1.model.CheckCanDeleteSLOResponse;
import com.datadog.api.client.v1.model.SLOBulkDeleteResponse;
import com.datadog.api.client.v1.model.SLOCorrectionListResponse;
import com.datadog.api.client.v1.model.SLODeleteResponse;
import com.datadog.api.client.v1.model.SLOHistoryResponse;
import com.datadog.api.client.v1.model.SLOListResponse;
import com.datadog.api.client.v1.model.SLOResponse;
import com.datadog.api.client.v1.model.SLOTimeframe;
import com.datadog.api.client.v1.model.SearchSLOResponse;
import com.datadog.api.client.v1.model.ServiceLevelObjective;
import com.datadog.api.client.v1.model.ServiceLevelObjectiveRequest;
import jakarta.ws.rs.client.Invocation;
import jakarta.ws.rs.core.GenericType;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import java.util.concurrent.CompletableFuture;
@jakarta.annotation.Generated(
value = "https://github.com/DataDog/datadog-api-client-java/blob/master/.generator")
public class ServiceLevelObjectivesApi {
private ApiClient apiClient;
public ServiceLevelObjectivesApi() {
this(ApiClient.getDefaultApiClient());
}
public ServiceLevelObjectivesApi(ApiClient apiClient) {
this.apiClient = apiClient;
}
/**
* Get the API client.
*
* @return API client
*/
public ApiClient getApiClient() {
return apiClient;
}
/**
* Set the API client.
*
* @param apiClient an instance of API client
*/
public void setApiClient(ApiClient apiClient) {
this.apiClient = apiClient;
}
/**
* Check if SLOs can be safely deleted.
*
* See {@link #checkCanDeleteSLOWithHttpInfo}.
*
* @param ids A comma separated list of the IDs of the service level objectives objects.
* (required)
* @return CheckCanDeleteSLOResponse
* @throws ApiException if fails to make API call
*/
public CheckCanDeleteSLOResponse checkCanDeleteSLO(String ids) throws ApiException {
return checkCanDeleteSLOWithHttpInfo(ids).getData();
}
/**
* Check if SLOs can be safely deleted.
*
*
See {@link #checkCanDeleteSLOWithHttpInfoAsync}.
*
* @param ids A comma separated list of the IDs of the service level objectives objects.
* (required)
* @return CompletableFuture<CheckCanDeleteSLOResponse>
*/
public CompletableFuture checkCanDeleteSLOAsync(String ids) {
return checkCanDeleteSLOWithHttpInfoAsync(ids)
.thenApply(
response -> {
return response.getData();
});
}
/**
* Check if an SLO can be safely deleted. For example, assure an SLO can be deleted without
* disrupting a dashboard.
*
* @param ids A comma separated list of the IDs of the service level objectives objects.
* (required)
* @return ApiResponse<CheckCanDeleteSLOResponse>
* @throws ApiException if fails to make API call
* @http.response.details
*
* Response details
* Status Code Description Response Headers
* 200 OK -
* 400 Bad Request -
* 403 Forbidden -
* 409 Conflict -
* 429 Too many requests -
*
*/
public ApiResponse checkCanDeleteSLOWithHttpInfo(String ids)
throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'ids' is set
if (ids == null) {
throw new ApiException(
400, "Missing the required parameter 'ids' when calling checkCanDeleteSLO");
}
// create path and map variables
String localVarPath = "/api/v1/slo/can_delete";
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPairs("", "ids", ids));
Invocation.Builder builder =
apiClient.createBuilder(
"v1.ServiceLevelObjectivesApi.checkCanDeleteSLO",
localVarPath,
localVarQueryParams,
localVarHeaderParams,
new HashMap(),
new String[] {"application/json"},
new String[] {"AuthZ", "apiKeyAuth", "appKeyAuth"});
return apiClient.invokeAPI(
"GET",
builder,
localVarHeaderParams,
new String[] {},
localVarPostBody,
new HashMap(),
false,
new GenericType() {});
}
/**
* Check if SLOs can be safely deleted.
*
* See {@link #checkCanDeleteSLOWithHttpInfo}.
*
* @param ids A comma separated list of the IDs of the service level objectives objects.
* (required)
* @return CompletableFuture<ApiResponse<CheckCanDeleteSLOResponse>>
*/
public CompletableFuture>
checkCanDeleteSLOWithHttpInfoAsync(String ids) {
Object localVarPostBody = null;
// verify the required parameter 'ids' is set
if (ids == null) {
CompletableFuture> result = new CompletableFuture<>();
result.completeExceptionally(
new ApiException(
400, "Missing the required parameter 'ids' when calling checkCanDeleteSLO"));
return result;
}
// create path and map variables
String localVarPath = "/api/v1/slo/can_delete";
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPairs("", "ids", ids));
Invocation.Builder builder;
try {
builder =
apiClient.createBuilder(
"v1.ServiceLevelObjectivesApi.checkCanDeleteSLO",
localVarPath,
localVarQueryParams,
localVarHeaderParams,
new HashMap(),
new String[] {"application/json"},
new String[] {"AuthZ", "apiKeyAuth", "appKeyAuth"});
} catch (ApiException ex) {
CompletableFuture> result = new CompletableFuture<>();
result.completeExceptionally(ex);
return result;
}
return apiClient.invokeAPIAsync(
"GET",
builder,
localVarHeaderParams,
new String[] {},
localVarPostBody,
new HashMap(),
false,
new GenericType() {});
}
/**
* Create an SLO object.
*
* See {@link #createSLOWithHttpInfo}.
*
* @param body Service level objective request object. (required)
* @return SLOListResponse
* @throws ApiException if fails to make API call
*/
public SLOListResponse createSLO(ServiceLevelObjectiveRequest body) throws ApiException {
return createSLOWithHttpInfo(body).getData();
}
/**
* Create an SLO object.
*
*
See {@link #createSLOWithHttpInfoAsync}.
*
* @param body Service level objective request object. (required)
* @return CompletableFuture<SLOListResponse>
*/
public CompletableFuture createSLOAsync(ServiceLevelObjectiveRequest body) {
return createSLOWithHttpInfoAsync(body)
.thenApply(
response -> {
return response.getData();
});
}
/**
* Create a service level objective object.
*
* @param body Service level objective request object. (required)
* @return ApiResponse<SLOListResponse>
* @throws ApiException if fails to make API call
* @http.response.details
*
* Response details
* Status Code Description Response Headers
* 200 OK -
* 400 Bad Request -
* 403 Forbidden -
* 429 Too many requests -
*
*/
public ApiResponse createSLOWithHttpInfo(ServiceLevelObjectiveRequest body)
throws ApiException {
Object localVarPostBody = body;
// verify the required parameter 'body' is set
if (body == null) {
throw new ApiException(400, "Missing the required parameter 'body' when calling createSLO");
}
// create path and map variables
String localVarPath = "/api/v1/slo";
Map localVarHeaderParams = new HashMap();
Invocation.Builder builder =
apiClient.createBuilder(
"v1.ServiceLevelObjectivesApi.createSLO",
localVarPath,
new ArrayList(),
localVarHeaderParams,
new HashMap(),
new String[] {"application/json"},
new String[] {"AuthZ", "apiKeyAuth", "appKeyAuth"});
return apiClient.invokeAPI(
"POST",
builder,
localVarHeaderParams,
new String[] {"application/json"},
localVarPostBody,
new HashMap(),
false,
new GenericType() {});
}
/**
* Create an SLO object.
*
* See {@link #createSLOWithHttpInfo}.
*
* @param body Service level objective request object. (required)
* @return CompletableFuture<ApiResponse<SLOListResponse>>
*/
public CompletableFuture> createSLOWithHttpInfoAsync(
ServiceLevelObjectiveRequest body) {
Object localVarPostBody = body;
// verify the required parameter 'body' is set
if (body == null) {
CompletableFuture> result = new CompletableFuture<>();
result.completeExceptionally(
new ApiException(400, "Missing the required parameter 'body' when calling createSLO"));
return result;
}
// create path and map variables
String localVarPath = "/api/v1/slo";
Map localVarHeaderParams = new HashMap();
Invocation.Builder builder;
try {
builder =
apiClient.createBuilder(
"v1.ServiceLevelObjectivesApi.createSLO",
localVarPath,
new ArrayList(),
localVarHeaderParams,
new HashMap(),
new String[] {"application/json"},
new String[] {"AuthZ", "apiKeyAuth", "appKeyAuth"});
} catch (ApiException ex) {
CompletableFuture> result = new CompletableFuture<>();
result.completeExceptionally(ex);
return result;
}
return apiClient.invokeAPIAsync(
"POST",
builder,
localVarHeaderParams,
new String[] {"application/json"},
localVarPostBody,
new HashMap(),
false,
new GenericType() {});
}
/** Manage optional parameters to deleteSLO. */
public static class DeleteSLOOptionalParameters {
private String force;
/**
* Set force.
*
* @param force Delete the monitor even if it's referenced by other resources (for example SLO,
* composite monitor). (optional)
* @return DeleteSLOOptionalParameters
*/
public DeleteSLOOptionalParameters force(String force) {
this.force = force;
return this;
}
}
/**
* Delete an SLO.
*
* See {@link #deleteSLOWithHttpInfo}.
*
* @param sloId The ID of the service level objective. (required)
* @return SLODeleteResponse
* @throws ApiException if fails to make API call
*/
public SLODeleteResponse deleteSLO(String sloId) throws ApiException {
return deleteSLOWithHttpInfo(sloId, new DeleteSLOOptionalParameters()).getData();
}
/**
* Delete an SLO.
*
*
See {@link #deleteSLOWithHttpInfoAsync}.
*
* @param sloId The ID of the service level objective. (required)
* @return CompletableFuture<SLODeleteResponse>
*/
public CompletableFuture deleteSLOAsync(String sloId) {
return deleteSLOWithHttpInfoAsync(sloId, new DeleteSLOOptionalParameters())
.thenApply(
response -> {
return response.getData();
});
}
/**
* Delete an SLO.
*
* See {@link #deleteSLOWithHttpInfo}.
*
* @param sloId The ID of the service level objective. (required)
* @param parameters Optional parameters for the request.
* @return SLODeleteResponse
* @throws ApiException if fails to make API call
*/
public SLODeleteResponse deleteSLO(String sloId, DeleteSLOOptionalParameters parameters)
throws ApiException {
return deleteSLOWithHttpInfo(sloId, parameters).getData();
}
/**
* Delete an SLO.
*
*
See {@link #deleteSLOWithHttpInfoAsync}.
*
* @param sloId The ID of the service level objective. (required)
* @param parameters Optional parameters for the request.
* @return CompletableFuture<SLODeleteResponse>
*/
public CompletableFuture deleteSLOAsync(
String sloId, DeleteSLOOptionalParameters parameters) {
return deleteSLOWithHttpInfoAsync(sloId, parameters)
.thenApply(
response -> {
return response.getData();
});
}
/**
* Permanently delete the specified service level objective object.
*
* If an SLO is used in a dashboard, the DELETE /v1/slo/
endpoint returns a 409
* conflict error because the SLO is referenced in a dashboard.
*
* @param sloId The ID of the service level objective. (required)
* @param parameters Optional parameters for the request.
* @return ApiResponse<SLODeleteResponse>
* @throws ApiException if fails to make API call
* @http.response.details
*
* Response details
* Status Code Description Response Headers
* 200 OK -
* 403 Forbidden -
* 404 Not found -
* 409 Conflict -
* 429 Too many requests -
*
*/
public ApiResponse deleteSLOWithHttpInfo(
String sloId, DeleteSLOOptionalParameters parameters) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'sloId' is set
if (sloId == null) {
throw new ApiException(400, "Missing the required parameter 'sloId' when calling deleteSLO");
}
String force = parameters.force;
// create path and map variables
String localVarPath =
"/api/v1/slo/{slo_id}"
.replaceAll("\\{" + "slo_id" + "\\}", apiClient.escapeString(sloId.toString()));
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPairs("", "force", force));
Invocation.Builder builder =
apiClient.createBuilder(
"v1.ServiceLevelObjectivesApi.deleteSLO",
localVarPath,
localVarQueryParams,
localVarHeaderParams,
new HashMap(),
new String[] {"application/json"},
new String[] {"AuthZ", "apiKeyAuth", "appKeyAuth"});
return apiClient.invokeAPI(
"DELETE",
builder,
localVarHeaderParams,
new String[] {},
localVarPostBody,
new HashMap(),
false,
new GenericType() {});
}
/**
* Delete an SLO.
*
* See {@link #deleteSLOWithHttpInfo}.
*
* @param sloId The ID of the service level objective. (required)
* @param parameters Optional parameters for the request.
* @return CompletableFuture<ApiResponse<SLODeleteResponse>>
*/
public CompletableFuture> deleteSLOWithHttpInfoAsync(
String sloId, DeleteSLOOptionalParameters parameters) {
Object localVarPostBody = null;
// verify the required parameter 'sloId' is set
if (sloId == null) {
CompletableFuture> result = new CompletableFuture<>();
result.completeExceptionally(
new ApiException(400, "Missing the required parameter 'sloId' when calling deleteSLO"));
return result;
}
String force = parameters.force;
// create path and map variables
String localVarPath =
"/api/v1/slo/{slo_id}"
.replaceAll("\\{" + "slo_id" + "\\}", apiClient.escapeString(sloId.toString()));
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPairs("", "force", force));
Invocation.Builder builder;
try {
builder =
apiClient.createBuilder(
"v1.ServiceLevelObjectivesApi.deleteSLO",
localVarPath,
localVarQueryParams,
localVarHeaderParams,
new HashMap(),
new String[] {"application/json"},
new String[] {"AuthZ", "apiKeyAuth", "appKeyAuth"});
} catch (ApiException ex) {
CompletableFuture> result = new CompletableFuture<>();
result.completeExceptionally(ex);
return result;
}
return apiClient.invokeAPIAsync(
"DELETE",
builder,
localVarHeaderParams,
new String[] {},
localVarPostBody,
new HashMap(),
false,
new GenericType() {});
}
/**
* Bulk Delete SLO Timeframes.
*
* See {@link #deleteSLOTimeframeInBulkWithHttpInfo}.
*
* @param body Delete multiple service level objective objects request body. (required)
* @return SLOBulkDeleteResponse
* @throws ApiException if fails to make API call
*/
public SLOBulkDeleteResponse deleteSLOTimeframeInBulk(Map> body)
throws ApiException {
return deleteSLOTimeframeInBulkWithHttpInfo(body).getData();
}
/**
* Bulk Delete SLO Timeframes.
*
* See {@link #deleteSLOTimeframeInBulkWithHttpInfoAsync}.
*
* @param body Delete multiple service level objective objects request body. (required)
* @return CompletableFuture<SLOBulkDeleteResponse>
*/
public CompletableFuture deleteSLOTimeframeInBulkAsync(
Map> body) {
return deleteSLOTimeframeInBulkWithHttpInfoAsync(body)
.thenApply(
response -> {
return response.getData();
});
}
/**
* Delete (or partially delete) multiple service level objective objects.
*
* This endpoint facilitates deletion of one or more thresholds for one or more service level
* objective objects. If all thresholds are deleted, the service level objective object is deleted
* as well.
*
* @param body Delete multiple service level objective objects request body. (required)
* @return ApiResponse<SLOBulkDeleteResponse>
* @throws ApiException if fails to make API call
* @http.response.details
*
* Response details
* Status Code Description Response Headers
* 200 OK -
* 400 Bad Request -
* 403 Forbidden -
* 429 Too many requests -
*
*/
public ApiResponse deleteSLOTimeframeInBulkWithHttpInfo(
Map> body) throws ApiException {
Object localVarPostBody = body;
// verify the required parameter 'body' is set
if (body == null) {
throw new ApiException(
400, "Missing the required parameter 'body' when calling deleteSLOTimeframeInBulk");
}
// create path and map variables
String localVarPath = "/api/v1/slo/bulk_delete";
Map localVarHeaderParams = new HashMap();
Invocation.Builder builder =
apiClient.createBuilder(
"v1.ServiceLevelObjectivesApi.deleteSLOTimeframeInBulk",
localVarPath,
new ArrayList(),
localVarHeaderParams,
new HashMap(),
new String[] {"application/json"},
new String[] {"AuthZ", "apiKeyAuth", "appKeyAuth"});
return apiClient.invokeAPI(
"POST",
builder,
localVarHeaderParams,
new String[] {"application/json"},
localVarPostBody,
new HashMap(),
false,
new GenericType() {});
}
/**
* Bulk Delete SLO Timeframes.
*
* See {@link #deleteSLOTimeframeInBulkWithHttpInfo}.
*
* @param body Delete multiple service level objective objects request body. (required)
* @return CompletableFuture<ApiResponse<SLOBulkDeleteResponse>>
*/
public CompletableFuture>
deleteSLOTimeframeInBulkWithHttpInfoAsync(Map> body) {
Object localVarPostBody = body;
// verify the required parameter 'body' is set
if (body == null) {
CompletableFuture> result = new CompletableFuture<>();
result.completeExceptionally(
new ApiException(
400, "Missing the required parameter 'body' when calling deleteSLOTimeframeInBulk"));
return result;
}
// create path and map variables
String localVarPath = "/api/v1/slo/bulk_delete";
Map localVarHeaderParams = new HashMap();
Invocation.Builder builder;
try {
builder =
apiClient.createBuilder(
"v1.ServiceLevelObjectivesApi.deleteSLOTimeframeInBulk",
localVarPath,
new ArrayList(),
localVarHeaderParams,
new HashMap(),
new String[] {"application/json"},
new String[] {"AuthZ", "apiKeyAuth", "appKeyAuth"});
} catch (ApiException ex) {
CompletableFuture> result = new CompletableFuture<>();
result.completeExceptionally(ex);
return result;
}
return apiClient.invokeAPIAsync(
"POST",
builder,
localVarHeaderParams,
new String[] {"application/json"},
localVarPostBody,
new HashMap(),
false,
new GenericType() {});
}
/** Manage optional parameters to getSLO. */
public static class GetSLOOptionalParameters {
private Boolean withConfiguredAlertIds;
/**
* Set withConfiguredAlertIds.
*
* @param withConfiguredAlertIds Get the IDs of SLO monitors that reference this SLO. (optional)
* @return GetSLOOptionalParameters
*/
public GetSLOOptionalParameters withConfiguredAlertIds(Boolean withConfiguredAlertIds) {
this.withConfiguredAlertIds = withConfiguredAlertIds;
return this;
}
}
/**
* Get an SLO's details.
*
* See {@link #getSLOWithHttpInfo}.
*
* @param sloId The ID of the service level objective object. (required)
* @return SLOResponse
* @throws ApiException if fails to make API call
*/
public SLOResponse getSLO(String sloId) throws ApiException {
return getSLOWithHttpInfo(sloId, new GetSLOOptionalParameters()).getData();
}
/**
* Get an SLO's details.
*
*
See {@link #getSLOWithHttpInfoAsync}.
*
* @param sloId The ID of the service level objective object. (required)
* @return CompletableFuture<SLOResponse>
*/
public CompletableFuture getSLOAsync(String sloId) {
return getSLOWithHttpInfoAsync(sloId, new GetSLOOptionalParameters())
.thenApply(
response -> {
return response.getData();
});
}
/**
* Get an SLO's details.
*
* See {@link #getSLOWithHttpInfo}.
*
* @param sloId The ID of the service level objective object. (required)
* @param parameters Optional parameters for the request.
* @return SLOResponse
* @throws ApiException if fails to make API call
*/
public SLOResponse getSLO(String sloId, GetSLOOptionalParameters parameters) throws ApiException {
return getSLOWithHttpInfo(sloId, parameters).getData();
}
/**
* Get an SLO's details.
*
*
See {@link #getSLOWithHttpInfoAsync}.
*
* @param sloId The ID of the service level objective object. (required)
* @param parameters Optional parameters for the request.
* @return CompletableFuture<SLOResponse>
*/
public CompletableFuture getSLOAsync(
String sloId, GetSLOOptionalParameters parameters) {
return getSLOWithHttpInfoAsync(sloId, parameters)
.thenApply(
response -> {
return response.getData();
});
}
/**
* Get a service level objective object.
*
* @param sloId The ID of the service level objective object. (required)
* @param parameters Optional parameters for the request.
* @return ApiResponse<SLOResponse>
* @throws ApiException if fails to make API call
* @http.response.details
*
* Response details
* Status Code Description Response Headers
* 200 OK -
* 403 Forbidden -
* 404 Not found -
* 429 Too many requests -
*
*/
public ApiResponse getSLOWithHttpInfo(
String sloId, GetSLOOptionalParameters parameters) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'sloId' is set
if (sloId == null) {
throw new ApiException(400, "Missing the required parameter 'sloId' when calling getSLO");
}
Boolean withConfiguredAlertIds = parameters.withConfiguredAlertIds;
// create path and map variables
String localVarPath =
"/api/v1/slo/{slo_id}"
.replaceAll("\\{" + "slo_id" + "\\}", apiClient.escapeString(sloId.toString()));
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
localVarQueryParams.addAll(
apiClient.parameterToPairs("", "with_configured_alert_ids", withConfiguredAlertIds));
Invocation.Builder builder =
apiClient.createBuilder(
"v1.ServiceLevelObjectivesApi.getSLO",
localVarPath,
localVarQueryParams,
localVarHeaderParams,
new HashMap(),
new String[] {"application/json"},
new String[] {"AuthZ", "apiKeyAuth", "appKeyAuth"});
return apiClient.invokeAPI(
"GET",
builder,
localVarHeaderParams,
new String[] {},
localVarPostBody,
new HashMap(),
false,
new GenericType() {});
}
/**
* Get an SLO's details.
*
* See {@link #getSLOWithHttpInfo}.
*
* @param sloId The ID of the service level objective object. (required)
* @param parameters Optional parameters for the request.
* @return CompletableFuture<ApiResponse<SLOResponse>>
*/
public CompletableFuture> getSLOWithHttpInfoAsync(
String sloId, GetSLOOptionalParameters parameters) {
Object localVarPostBody = null;
// verify the required parameter 'sloId' is set
if (sloId == null) {
CompletableFuture> result = new CompletableFuture<>();
result.completeExceptionally(
new ApiException(400, "Missing the required parameter 'sloId' when calling getSLO"));
return result;
}
Boolean withConfiguredAlertIds = parameters.withConfiguredAlertIds;
// create path and map variables
String localVarPath =
"/api/v1/slo/{slo_id}"
.replaceAll("\\{" + "slo_id" + "\\}", apiClient.escapeString(sloId.toString()));
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
localVarQueryParams.addAll(
apiClient.parameterToPairs("", "with_configured_alert_ids", withConfiguredAlertIds));
Invocation.Builder builder;
try {
builder =
apiClient.createBuilder(
"v1.ServiceLevelObjectivesApi.getSLO",
localVarPath,
localVarQueryParams,
localVarHeaderParams,
new HashMap(),
new String[] {"application/json"},
new String[] {"AuthZ", "apiKeyAuth", "appKeyAuth"});
} catch (ApiException ex) {
CompletableFuture> result = new CompletableFuture<>();
result.completeExceptionally(ex);
return result;
}
return apiClient.invokeAPIAsync(
"GET",
builder,
localVarHeaderParams,
new String[] {},
localVarPostBody,
new HashMap(),
false,
new GenericType() {});
}
/**
* Get Corrections For an SLO.
*
* See {@link #getSLOCorrectionsWithHttpInfo}.
*
* @param sloId The ID of the service level objective object. (required)
* @return SLOCorrectionListResponse
* @throws ApiException if fails to make API call
*/
public SLOCorrectionListResponse getSLOCorrections(String sloId) throws ApiException {
return getSLOCorrectionsWithHttpInfo(sloId).getData();
}
/**
* Get Corrections For an SLO.
*
*
See {@link #getSLOCorrectionsWithHttpInfoAsync}.
*
* @param sloId The ID of the service level objective object. (required)
* @return CompletableFuture<SLOCorrectionListResponse>
*/
public CompletableFuture getSLOCorrectionsAsync(String sloId) {
return getSLOCorrectionsWithHttpInfoAsync(sloId)
.thenApply(
response -> {
return response.getData();
});
}
/**
* Get corrections applied to an SLO
*
* @param sloId The ID of the service level objective object. (required)
* @return ApiResponse<SLOCorrectionListResponse>
* @throws ApiException if fails to make API call
* @http.response.details
*
* Response details
* Status Code Description Response Headers
* 200 OK -
* 400 Bad Request -
* 403 Forbidden -
* 404 Not Found -
* 429 Too many requests -
*
*/
public ApiResponse getSLOCorrectionsWithHttpInfo(String sloId)
throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'sloId' is set
if (sloId == null) {
throw new ApiException(
400, "Missing the required parameter 'sloId' when calling getSLOCorrections");
}
// create path and map variables
String localVarPath =
"/api/v1/slo/{slo_id}/corrections"
.replaceAll("\\{" + "slo_id" + "\\}", apiClient.escapeString(sloId.toString()));
Map localVarHeaderParams = new HashMap();
Invocation.Builder builder =
apiClient.createBuilder(
"v1.ServiceLevelObjectivesApi.getSLOCorrections",
localVarPath,
new ArrayList(),
localVarHeaderParams,
new HashMap(),
new String[] {"application/json"},
new String[] {"AuthZ", "apiKeyAuth", "appKeyAuth"});
return apiClient.invokeAPI(
"GET",
builder,
localVarHeaderParams,
new String[] {},
localVarPostBody,
new HashMap(),
false,
new GenericType() {});
}
/**
* Get Corrections For an SLO.
*
* See {@link #getSLOCorrectionsWithHttpInfo}.
*
* @param sloId The ID of the service level objective object. (required)
* @return CompletableFuture<ApiResponse<SLOCorrectionListResponse>>
*/
public CompletableFuture>
getSLOCorrectionsWithHttpInfoAsync(String sloId) {
Object localVarPostBody = null;
// verify the required parameter 'sloId' is set
if (sloId == null) {
CompletableFuture> result = new CompletableFuture<>();
result.completeExceptionally(
new ApiException(
400, "Missing the required parameter 'sloId' when calling getSLOCorrections"));
return result;
}
// create path and map variables
String localVarPath =
"/api/v1/slo/{slo_id}/corrections"
.replaceAll("\\{" + "slo_id" + "\\}", apiClient.escapeString(sloId.toString()));
Map localVarHeaderParams = new HashMap();
Invocation.Builder builder;
try {
builder =
apiClient.createBuilder(
"v1.ServiceLevelObjectivesApi.getSLOCorrections",
localVarPath,
new ArrayList(),
localVarHeaderParams,
new HashMap(),
new String[] {"application/json"},
new String[] {"AuthZ", "apiKeyAuth", "appKeyAuth"});
} catch (ApiException ex) {
CompletableFuture> result = new CompletableFuture<>();
result.completeExceptionally(ex);
return result;
}
return apiClient.invokeAPIAsync(
"GET",
builder,
localVarHeaderParams,
new String[] {},
localVarPostBody,
new HashMap(),
false,
new GenericType() {});
}
/** Manage optional parameters to getSLOHistory. */
public static class GetSLOHistoryOptionalParameters {
private Double target;
private Boolean applyCorrection;
/**
* Set target.
*
* @param target The SLO target. If target
is passed in, the response will include
* the remaining error budget and a timeframe value of custom
. (optional)
* @return GetSLOHistoryOptionalParameters
*/
public GetSLOHistoryOptionalParameters target(Double target) {
this.target = target;
return this;
}
/**
* Set applyCorrection.
*
* @param applyCorrection Defaults to true
. If any SLO corrections are applied and
* this parameter is set to false
, then the corrections will not be applied and
* the SLI values will not be affected. (optional)
* @return GetSLOHistoryOptionalParameters
*/
public GetSLOHistoryOptionalParameters applyCorrection(Boolean applyCorrection) {
this.applyCorrection = applyCorrection;
return this;
}
}
/**
* Get an SLO's history.
*
* See {@link #getSLOHistoryWithHttpInfo}.
*
* @param sloId The ID of the service level objective object. (required)
* @param fromTs The from
timestamp for the query window in epoch seconds. (required)
* @param toTs The to
timestamp for the query window in epoch seconds. (required)
* @return SLOHistoryResponse
* @throws ApiException if fails to make API call
*/
public SLOHistoryResponse getSLOHistory(String sloId, Long fromTs, Long toTs)
throws ApiException {
return getSLOHistoryWithHttpInfo(sloId, fromTs, toTs, new GetSLOHistoryOptionalParameters())
.getData();
}
/**
* Get an SLO's history.
*
*
See {@link #getSLOHistoryWithHttpInfoAsync}.
*
* @param sloId The ID of the service level objective object. (required)
* @param fromTs The from
timestamp for the query window in epoch seconds. (required)
* @param toTs The to
timestamp for the query window in epoch seconds. (required)
* @return CompletableFuture<SLOHistoryResponse>
*/
public CompletableFuture getSLOHistoryAsync(
String sloId, Long fromTs, Long toTs) {
return getSLOHistoryWithHttpInfoAsync(
sloId, fromTs, toTs, new GetSLOHistoryOptionalParameters())
.thenApply(
response -> {
return response.getData();
});
}
/**
* Get an SLO's history.
*
* See {@link #getSLOHistoryWithHttpInfo}.
*
* @param sloId The ID of the service level objective object. (required)
* @param fromTs The from
timestamp for the query window in epoch seconds. (required)
* @param toTs The to
timestamp for the query window in epoch seconds. (required)
* @param parameters Optional parameters for the request.
* @return SLOHistoryResponse
* @throws ApiException if fails to make API call
*/
public SLOHistoryResponse getSLOHistory(
String sloId, Long fromTs, Long toTs, GetSLOHistoryOptionalParameters parameters)
throws ApiException {
return getSLOHistoryWithHttpInfo(sloId, fromTs, toTs, parameters).getData();
}
/**
* Get an SLO's history.
*
*
See {@link #getSLOHistoryWithHttpInfoAsync}.
*
* @param sloId The ID of the service level objective object. (required)
* @param fromTs The from
timestamp for the query window in epoch seconds. (required)
* @param toTs The to
timestamp for the query window in epoch seconds. (required)
* @param parameters Optional parameters for the request.
* @return CompletableFuture<SLOHistoryResponse>
*/
public CompletableFuture getSLOHistoryAsync(
String sloId, Long fromTs, Long toTs, GetSLOHistoryOptionalParameters parameters) {
return getSLOHistoryWithHttpInfoAsync(sloId, fromTs, toTs, parameters)
.thenApply(
response -> {
return response.getData();
});
}
/**
* Get a specific SLO’s history, regardless of its SLO type.
*
* The detailed history data is structured according to the source data type. For example,
* metric data is included for event SLOs that use the metric source, and monitor SLO types
* include the monitor transition history.
*
*
Note: There are different response formats for event based and time based
* SLOs. Examples of both are shown.
*
* @param sloId The ID of the service level objective object. (required)
* @param fromTs The from
timestamp for the query window in epoch seconds. (required)
* @param toTs The to
timestamp for the query window in epoch seconds. (required)
* @param parameters Optional parameters for the request.
* @return ApiResponse<SLOHistoryResponse>
* @throws ApiException if fails to make API call
* @http.response.details
*
* Response details
* Status Code Description Response Headers
* 200 OK -
* 400 Bad Request -
* 403 Forbidden -
* 404 Not Found -
* 429 Too many requests -
*
*/
public ApiResponse getSLOHistoryWithHttpInfo(
String sloId, Long fromTs, Long toTs, GetSLOHistoryOptionalParameters parameters)
throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'sloId' is set
if (sloId == null) {
throw new ApiException(
400, "Missing the required parameter 'sloId' when calling getSLOHistory");
}
// verify the required parameter 'fromTs' is set
if (fromTs == null) {
throw new ApiException(
400, "Missing the required parameter 'fromTs' when calling getSLOHistory");
}
// verify the required parameter 'toTs' is set
if (toTs == null) {
throw new ApiException(
400, "Missing the required parameter 'toTs' when calling getSLOHistory");
}
Double target = parameters.target;
Boolean applyCorrection = parameters.applyCorrection;
// create path and map variables
String localVarPath =
"/api/v1/slo/{slo_id}/history"
.replaceAll("\\{" + "slo_id" + "\\}", apiClient.escapeString(sloId.toString()));
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPairs("", "from_ts", fromTs));
localVarQueryParams.addAll(apiClient.parameterToPairs("", "to_ts", toTs));
localVarQueryParams.addAll(apiClient.parameterToPairs("", "target", target));
localVarQueryParams.addAll(apiClient.parameterToPairs("", "apply_correction", applyCorrection));
Invocation.Builder builder =
apiClient.createBuilder(
"v1.ServiceLevelObjectivesApi.getSLOHistory",
localVarPath,
localVarQueryParams,
localVarHeaderParams,
new HashMap(),
new String[] {"application/json"},
new String[] {"AuthZ", "apiKeyAuth", "appKeyAuth"});
return apiClient.invokeAPI(
"GET",
builder,
localVarHeaderParams,
new String[] {},
localVarPostBody,
new HashMap(),
false,
new GenericType() {});
}
/**
* Get an SLO's history.
*
* See {@link #getSLOHistoryWithHttpInfo}.
*
* @param sloId The ID of the service level objective object. (required)
* @param fromTs The from
timestamp for the query window in epoch seconds. (required)
* @param toTs The to
timestamp for the query window in epoch seconds. (required)
* @param parameters Optional parameters for the request.
* @return CompletableFuture<ApiResponse<SLOHistoryResponse>>
*/
public CompletableFuture> getSLOHistoryWithHttpInfoAsync(
String sloId, Long fromTs, Long toTs, GetSLOHistoryOptionalParameters parameters) {
Object localVarPostBody = null;
// verify the required parameter 'sloId' is set
if (sloId == null) {
CompletableFuture> result = new CompletableFuture<>();
result.completeExceptionally(
new ApiException(
400, "Missing the required parameter 'sloId' when calling getSLOHistory"));
return result;
}
// verify the required parameter 'fromTs' is set
if (fromTs == null) {
CompletableFuture> result = new CompletableFuture<>();
result.completeExceptionally(
new ApiException(
400, "Missing the required parameter 'fromTs' when calling getSLOHistory"));
return result;
}
// verify the required parameter 'toTs' is set
if (toTs == null) {
CompletableFuture> result = new CompletableFuture<>();
result.completeExceptionally(
new ApiException(
400, "Missing the required parameter 'toTs' when calling getSLOHistory"));
return result;
}
Double target = parameters.target;
Boolean applyCorrection = parameters.applyCorrection;
// create path and map variables
String localVarPath =
"/api/v1/slo/{slo_id}/history"
.replaceAll("\\{" + "slo_id" + "\\}", apiClient.escapeString(sloId.toString()));
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPairs("", "from_ts", fromTs));
localVarQueryParams.addAll(apiClient.parameterToPairs("", "to_ts", toTs));
localVarQueryParams.addAll(apiClient.parameterToPairs("", "target", target));
localVarQueryParams.addAll(apiClient.parameterToPairs("", "apply_correction", applyCorrection));
Invocation.Builder builder;
try {
builder =
apiClient.createBuilder(
"v1.ServiceLevelObjectivesApi.getSLOHistory",
localVarPath,
localVarQueryParams,
localVarHeaderParams,
new HashMap(),
new String[] {"application/json"},
new String[] {"AuthZ", "apiKeyAuth", "appKeyAuth"});
} catch (ApiException ex) {
CompletableFuture> result = new CompletableFuture<>();
result.completeExceptionally(ex);
return result;
}
return apiClient.invokeAPIAsync(
"GET",
builder,
localVarHeaderParams,
new String[] {},
localVarPostBody,
new HashMap(),
false,
new GenericType() {});
}
/** Manage optional parameters to listSLOs. */
public static class ListSLOsOptionalParameters {
private String ids;
private String query;
private String tagsQuery;
private String metricsQuery;
private Long limit;
private Long offset;
/**
* Set ids.
*
* @param ids A comma separated list of the IDs of the service level objectives objects.
* (optional)
* @return ListSLOsOptionalParameters
*/
public ListSLOsOptionalParameters ids(String ids) {
this.ids = ids;
return this;
}
/**
* Set query.
*
* @param query The query string to filter results based on SLO names. (optional)
* @return ListSLOsOptionalParameters
*/
public ListSLOsOptionalParameters query(String query) {
this.query = query;
return this;
}
/**
* Set tagsQuery.
*
* @param tagsQuery The query string to filter results based on a single SLO tag. (optional)
* @return ListSLOsOptionalParameters
*/
public ListSLOsOptionalParameters tagsQuery(String tagsQuery) {
this.tagsQuery = tagsQuery;
return this;
}
/**
* Set metricsQuery.
*
* @param metricsQuery The query string to filter results based on SLO numerator and
* denominator. (optional)
* @return ListSLOsOptionalParameters
*/
public ListSLOsOptionalParameters metricsQuery(String metricsQuery) {
this.metricsQuery = metricsQuery;
return this;
}
/**
* Set limit.
*
* @param limit The number of SLOs to return in the response. (optional, default to 1000)
* @return ListSLOsOptionalParameters
*/
public ListSLOsOptionalParameters limit(Long limit) {
this.limit = limit;
return this;
}
/**
* Set offset.
*
* @param offset The specific offset to use as the beginning of the returned response.
* (optional)
* @return ListSLOsOptionalParameters
*/
public ListSLOsOptionalParameters offset(Long offset) {
this.offset = offset;
return this;
}
}
/**
* Get all SLOs.
*
* See {@link #listSLOsWithHttpInfo}.
*
* @return SLOListResponse
* @throws ApiException if fails to make API call
*/
public SLOListResponse listSLOs() throws ApiException {
return listSLOsWithHttpInfo(new ListSLOsOptionalParameters()).getData();
}
/**
* Get all SLOs.
*
*
See {@link #listSLOsWithHttpInfoAsync}.
*
* @return CompletableFuture<SLOListResponse>
*/
public CompletableFuture listSLOsAsync() {
return listSLOsWithHttpInfoAsync(new ListSLOsOptionalParameters())
.thenApply(
response -> {
return response.getData();
});
}
/**
* Get all SLOs.
*
* See {@link #listSLOsWithHttpInfo}.
*
* @param parameters Optional parameters for the request.
* @return SLOListResponse
* @throws ApiException if fails to make API call
*/
public SLOListResponse listSLOs(ListSLOsOptionalParameters parameters) throws ApiException {
return listSLOsWithHttpInfo(parameters).getData();
}
/**
* Get all SLOs.
*
*
See {@link #listSLOsWithHttpInfoAsync}.
*
* @param parameters Optional parameters for the request.
* @return CompletableFuture<SLOListResponse>
*/
public CompletableFuture listSLOsAsync(ListSLOsOptionalParameters parameters) {
return listSLOsWithHttpInfoAsync(parameters)
.thenApply(
response -> {
return response.getData();
});
}
/**
* Get all SLOs.
*
* See {@link #listSLOsWithHttpInfo}.
*
* @return PaginationIterable<ServiceLevelObjective>
*/
public PaginationIterable listSLOsWithPagination() {
ListSLOsOptionalParameters parameters = new ListSLOsOptionalParameters();
return listSLOsWithPagination(parameters);
}
/**
* Get all SLOs.
*
* See {@link #listSLOsWithHttpInfo}.
*
* @return SLOListResponse
*/
public PaginationIterable listSLOsWithPagination(
ListSLOsOptionalParameters parameters) {
String resultsPath = "getData";
String valueGetterPath = "";
String valueSetterPath = "offset";
Boolean valueSetterParamOptional = true;
Long limit;
if (parameters.limit == null) {
limit = 1000l;
parameters.limit(limit);
} else {
limit = parameters.limit;
}
LinkedHashMap args = new LinkedHashMap();
args.put("optionalParams", parameters);
PaginationIterable iterator =
new PaginationIterable(
this,
"listSLOs",
resultsPath,
valueGetterPath,
valueSetterPath,
valueSetterParamOptional,
true,
limit,
args);
return iterator;
}
/**
* Get a list of service level objective objects for your organization.
*
* @param parameters Optional parameters for the request.
* @return ApiResponse<SLOListResponse>
* @throws ApiException if fails to make API call
* @http.response.details
*
* Response details
* Status Code Description Response Headers
* 200 OK -
* 400 Bad Request -
* 403 Forbidden -
* 404 Not Found -
* 429 Too many requests -
*
*/
public ApiResponse listSLOsWithHttpInfo(ListSLOsOptionalParameters parameters)
throws ApiException {
Object localVarPostBody = null;
String ids = parameters.ids;
String query = parameters.query;
String tagsQuery = parameters.tagsQuery;
String metricsQuery = parameters.metricsQuery;
Long limit = parameters.limit;
Long offset = parameters.offset;
// create path and map variables
String localVarPath = "/api/v1/slo";
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPairs("", "ids", ids));
localVarQueryParams.addAll(apiClient.parameterToPairs("", "query", query));
localVarQueryParams.addAll(apiClient.parameterToPairs("", "tags_query", tagsQuery));
localVarQueryParams.addAll(apiClient.parameterToPairs("", "metrics_query", metricsQuery));
localVarQueryParams.addAll(apiClient.parameterToPairs("", "limit", limit));
localVarQueryParams.addAll(apiClient.parameterToPairs("", "offset", offset));
Invocation.Builder builder =
apiClient.createBuilder(
"v1.ServiceLevelObjectivesApi.listSLOs",
localVarPath,
localVarQueryParams,
localVarHeaderParams,
new HashMap(),
new String[] {"application/json"},
new String[] {"AuthZ", "apiKeyAuth", "appKeyAuth"});
return apiClient.invokeAPI(
"GET",
builder,
localVarHeaderParams,
new String[] {},
localVarPostBody,
new HashMap(),
false,
new GenericType() {});
}
/**
* Get all SLOs.
*
* See {@link #listSLOsWithHttpInfo}.
*
* @param parameters Optional parameters for the request.
* @return CompletableFuture<ApiResponse<SLOListResponse>>
*/
public CompletableFuture> listSLOsWithHttpInfoAsync(
ListSLOsOptionalParameters parameters) {
Object localVarPostBody = null;
String ids = parameters.ids;
String query = parameters.query;
String tagsQuery = parameters.tagsQuery;
String metricsQuery = parameters.metricsQuery;
Long limit = parameters.limit;
Long offset = parameters.offset;
// create path and map variables
String localVarPath = "/api/v1/slo";
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPairs("", "ids", ids));
localVarQueryParams.addAll(apiClient.parameterToPairs("", "query", query));
localVarQueryParams.addAll(apiClient.parameterToPairs("", "tags_query", tagsQuery));
localVarQueryParams.addAll(apiClient.parameterToPairs("", "metrics_query", metricsQuery));
localVarQueryParams.addAll(apiClient.parameterToPairs("", "limit", limit));
localVarQueryParams.addAll(apiClient.parameterToPairs("", "offset", offset));
Invocation.Builder builder;
try {
builder =
apiClient.createBuilder(
"v1.ServiceLevelObjectivesApi.listSLOs",
localVarPath,
localVarQueryParams,
localVarHeaderParams,
new HashMap(),
new String[] {"application/json"},
new String[] {"AuthZ", "apiKeyAuth", "appKeyAuth"});
} catch (ApiException ex) {
CompletableFuture> result = new CompletableFuture<>();
result.completeExceptionally(ex);
return result;
}
return apiClient.invokeAPIAsync(
"GET",
builder,
localVarHeaderParams,
new String[] {},
localVarPostBody,
new HashMap(),
false,
new GenericType() {});
}
/** Manage optional parameters to searchSLO. */
public static class SearchSLOOptionalParameters {
private String query;
private Long pageSize;
private Long pageNumber;
private Boolean includeFacets;
/**
* Set query.
*
* @param query The query string to filter results based on SLO names. Some examples of queries
* include service:<service-name>
and <slo-name>
.
* (optional)
* @return SearchSLOOptionalParameters
*/
public SearchSLOOptionalParameters query(String query) {
this.query = query;
return this;
}
/**
* Set pageSize.
*
* @param pageSize The number of files to return in the response [default=10]
.
* (optional)
* @return SearchSLOOptionalParameters
*/
public SearchSLOOptionalParameters pageSize(Long pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* Set pageNumber.
*
* @param pageNumber The identifier of the first page to return. This parameter is used for the
* pagination feature [default=0]
. (optional)
* @return SearchSLOOptionalParameters
*/
public SearchSLOOptionalParameters pageNumber(Long pageNumber) {
this.pageNumber = pageNumber;
return this;
}
/**
* Set includeFacets.
*
* @param includeFacets Whether or not to return facet information in the response
* [default=false]
. (optional)
* @return SearchSLOOptionalParameters
*/
public SearchSLOOptionalParameters includeFacets(Boolean includeFacets) {
this.includeFacets = includeFacets;
return this;
}
}
/**
* Search for SLOs.
*
* See {@link #searchSLOWithHttpInfo}.
*
* @return SearchSLOResponse
* @throws ApiException if fails to make API call
*/
public SearchSLOResponse searchSLO() throws ApiException {
return searchSLOWithHttpInfo(new SearchSLOOptionalParameters()).getData();
}
/**
* Search for SLOs.
*
*
See {@link #searchSLOWithHttpInfoAsync}.
*
* @return CompletableFuture<SearchSLOResponse>
*/
public CompletableFuture searchSLOAsync() {
return searchSLOWithHttpInfoAsync(new SearchSLOOptionalParameters())
.thenApply(
response -> {
return response.getData();
});
}
/**
* Search for SLOs.
*
* See {@link #searchSLOWithHttpInfo}.
*
* @param parameters Optional parameters for the request.
* @return SearchSLOResponse
* @throws ApiException if fails to make API call
*/
public SearchSLOResponse searchSLO(SearchSLOOptionalParameters parameters) throws ApiException {
return searchSLOWithHttpInfo(parameters).getData();
}
/**
* Search for SLOs.
*
*
See {@link #searchSLOWithHttpInfoAsync}.
*
* @param parameters Optional parameters for the request.
* @return CompletableFuture<SearchSLOResponse>
*/
public CompletableFuture searchSLOAsync(
SearchSLOOptionalParameters parameters) {
return searchSLOWithHttpInfoAsync(parameters)
.thenApply(
response -> {
return response.getData();
});
}
/**
* Get a list of service level objective objects for your organization.
*
* @param parameters Optional parameters for the request.
* @return ApiResponse<SearchSLOResponse>
* @throws ApiException if fails to make API call
* @http.response.details
*
* Response details
* Status Code Description Response Headers
* 200 OK -
* 400 Bad Request -
* 403 Forbidden -
* 429 Too many requests -
*
*/
public ApiResponse searchSLOWithHttpInfo(
SearchSLOOptionalParameters parameters) throws ApiException {
Object localVarPostBody = null;
String query = parameters.query;
Long pageSize = parameters.pageSize;
Long pageNumber = parameters.pageNumber;
Boolean includeFacets = parameters.includeFacets;
// create path and map variables
String localVarPath = "/api/v1/slo/search";
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPairs("", "query", query));
localVarQueryParams.addAll(apiClient.parameterToPairs("", "page[size]", pageSize));
localVarQueryParams.addAll(apiClient.parameterToPairs("", "page[number]", pageNumber));
localVarQueryParams.addAll(apiClient.parameterToPairs("", "include_facets", includeFacets));
Invocation.Builder builder =
apiClient.createBuilder(
"v1.ServiceLevelObjectivesApi.searchSLO",
localVarPath,
localVarQueryParams,
localVarHeaderParams,
new HashMap(),
new String[] {"application/json"},
new String[] {"AuthZ", "apiKeyAuth", "appKeyAuth"});
return apiClient.invokeAPI(
"GET",
builder,
localVarHeaderParams,
new String[] {},
localVarPostBody,
new HashMap(),
false,
new GenericType() {});
}
/**
* Search for SLOs.
*
* See {@link #searchSLOWithHttpInfo}.
*
* @param parameters Optional parameters for the request.
* @return CompletableFuture<ApiResponse<SearchSLOResponse>>
*/
public CompletableFuture> searchSLOWithHttpInfoAsync(
SearchSLOOptionalParameters parameters) {
Object localVarPostBody = null;
String query = parameters.query;
Long pageSize = parameters.pageSize;
Long pageNumber = parameters.pageNumber;
Boolean includeFacets = parameters.includeFacets;
// create path and map variables
String localVarPath = "/api/v1/slo/search";
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPairs("", "query", query));
localVarQueryParams.addAll(apiClient.parameterToPairs("", "page[size]", pageSize));
localVarQueryParams.addAll(apiClient.parameterToPairs("", "page[number]", pageNumber));
localVarQueryParams.addAll(apiClient.parameterToPairs("", "include_facets", includeFacets));
Invocation.Builder builder;
try {
builder =
apiClient.createBuilder(
"v1.ServiceLevelObjectivesApi.searchSLO",
localVarPath,
localVarQueryParams,
localVarHeaderParams,
new HashMap(),
new String[] {"application/json"},
new String[] {"AuthZ", "apiKeyAuth", "appKeyAuth"});
} catch (ApiException ex) {
CompletableFuture> result = new CompletableFuture<>();
result.completeExceptionally(ex);
return result;
}
return apiClient.invokeAPIAsync(
"GET",
builder,
localVarHeaderParams,
new String[] {},
localVarPostBody,
new HashMap(),
false,
new GenericType() {});
}
/**
* Update an SLO.
*
* See {@link #updateSLOWithHttpInfo}.
*
* @param sloId The ID of the service level objective object. (required)
* @param body The edited service level objective request object. (required)
* @return SLOListResponse
* @throws ApiException if fails to make API call
*/
public SLOListResponse updateSLO(String sloId, ServiceLevelObjective body) throws ApiException {
return updateSLOWithHttpInfo(sloId, body).getData();
}
/**
* Update an SLO.
*
*
See {@link #updateSLOWithHttpInfoAsync}.
*
* @param sloId The ID of the service level objective object. (required)
* @param body The edited service level objective request object. (required)
* @return CompletableFuture<SLOListResponse>
*/
public CompletableFuture updateSLOAsync(
String sloId, ServiceLevelObjective body) {
return updateSLOWithHttpInfoAsync(sloId, body)
.thenApply(
response -> {
return response.getData();
});
}
/**
* Update the specified service level objective object.
*
* @param sloId The ID of the service level objective object. (required)
* @param body The edited service level objective request object. (required)
* @return ApiResponse<SLOListResponse>
* @throws ApiException if fails to make API call
* @http.response.details
*
* Response details
* Status Code Description Response Headers
* 200 OK -
* 400 Bad Request -
* 403 Forbidden -
* 404 Not Found -
* 429 Too many requests -
*
*/
public ApiResponse updateSLOWithHttpInfo(
String sloId, ServiceLevelObjective body) throws ApiException {
Object localVarPostBody = body;
// verify the required parameter 'sloId' is set
if (sloId == null) {
throw new ApiException(400, "Missing the required parameter 'sloId' when calling updateSLO");
}
// verify the required parameter 'body' is set
if (body == null) {
throw new ApiException(400, "Missing the required parameter 'body' when calling updateSLO");
}
// create path and map variables
String localVarPath =
"/api/v1/slo/{slo_id}"
.replaceAll("\\{" + "slo_id" + "\\}", apiClient.escapeString(sloId.toString()));
Map localVarHeaderParams = new HashMap();
Invocation.Builder builder =
apiClient.createBuilder(
"v1.ServiceLevelObjectivesApi.updateSLO",
localVarPath,
new ArrayList(),
localVarHeaderParams,
new HashMap(),
new String[] {"application/json"},
new String[] {"AuthZ", "apiKeyAuth", "appKeyAuth"});
return apiClient.invokeAPI(
"PUT",
builder,
localVarHeaderParams,
new String[] {"application/json"},
localVarPostBody,
new HashMap(),
false,
new GenericType() {});
}
/**
* Update an SLO.
*
* See {@link #updateSLOWithHttpInfo}.
*
* @param sloId The ID of the service level objective object. (required)
* @param body The edited service level objective request object. (required)
* @return CompletableFuture<ApiResponse<SLOListResponse>>
*/
public CompletableFuture> updateSLOWithHttpInfoAsync(
String sloId, ServiceLevelObjective body) {
Object localVarPostBody = body;
// verify the required parameter 'sloId' is set
if (sloId == null) {
CompletableFuture> result = new CompletableFuture<>();
result.completeExceptionally(
new ApiException(400, "Missing the required parameter 'sloId' when calling updateSLO"));
return result;
}
// verify the required parameter 'body' is set
if (body == null) {
CompletableFuture> result = new CompletableFuture<>();
result.completeExceptionally(
new ApiException(400, "Missing the required parameter 'body' when calling updateSLO"));
return result;
}
// create path and map variables
String localVarPath =
"/api/v1/slo/{slo_id}"
.replaceAll("\\{" + "slo_id" + "\\}", apiClient.escapeString(sloId.toString()));
Map localVarHeaderParams = new HashMap();
Invocation.Builder builder;
try {
builder =
apiClient.createBuilder(
"v1.ServiceLevelObjectivesApi.updateSLO",
localVarPath,
new ArrayList(),
localVarHeaderParams,
new HashMap(),
new String[] {"application/json"},
new String[] {"AuthZ", "apiKeyAuth", "appKeyAuth"});
} catch (ApiException ex) {
CompletableFuture> result = new CompletableFuture<>();
result.completeExceptionally(ex);
return result;
}
return apiClient.invokeAPIAsync(
"PUT",
builder,
localVarHeaderParams,
new String[] {"application/json"},
localVarPostBody,
new HashMap(),
false,
new GenericType() {});
}
}