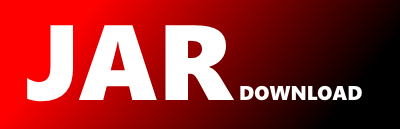
com.datadog.api.client.v2.api.ApiManagementApi Maven / Gradle / Ivy
package com.datadog.api.client.v2.api;
import com.datadog.api.client.ApiClient;
import com.datadog.api.client.ApiException;
import com.datadog.api.client.ApiResponse;
import com.datadog.api.client.Pair;
import com.datadog.api.client.v2.model.CreateOpenAPIResponse;
import com.datadog.api.client.v2.model.ListAPIsResponse;
import com.datadog.api.client.v2.model.UpdateOpenAPIResponse;
import jakarta.ws.rs.client.Invocation;
import jakarta.ws.rs.core.GenericType;
import java.io.File;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.UUID;
import java.util.concurrent.CompletableFuture;
@jakarta.annotation.Generated(
value = "https://github.com/DataDog/datadog-api-client-java/blob/master/.generator")
public class ApiManagementApi {
private ApiClient apiClient;
public ApiManagementApi() {
this(ApiClient.getDefaultApiClient());
}
public ApiManagementApi(ApiClient apiClient) {
this.apiClient = apiClient;
}
/**
* Get the API client.
*
* @return API client
*/
public ApiClient getApiClient() {
return apiClient;
}
/**
* Set the API client.
*
* @param apiClient an instance of API client
*/
public void setApiClient(ApiClient apiClient) {
this.apiClient = apiClient;
}
/** Manage optional parameters to createOpenAPI. */
public static class CreateOpenAPIOptionalParameters {
private File openapiSpecFile;
/**
* Set openapiSpecFile.
*
* @param openapiSpecFile Binary OpenAPI
spec file (optional)
* @return CreateOpenAPIOptionalParameters
*/
public CreateOpenAPIOptionalParameters openapiSpecFile(File openapiSpecFile) {
this.openapiSpecFile = openapiSpecFile;
return this;
}
}
/**
* Create a new API.
*
* See {@link #createOpenAPIWithHttpInfo}.
*
* @return CreateOpenAPIResponse
* @throws ApiException if fails to make API call
*/
public CreateOpenAPIResponse createOpenAPI() throws ApiException {
return createOpenAPIWithHttpInfo(new CreateOpenAPIOptionalParameters()).getData();
}
/**
* Create a new API.
*
*
See {@link #createOpenAPIWithHttpInfoAsync}.
*
* @return CompletableFuture<CreateOpenAPIResponse>
*/
public CompletableFuture createOpenAPIAsync() {
return createOpenAPIWithHttpInfoAsync(new CreateOpenAPIOptionalParameters())
.thenApply(
response -> {
return response.getData();
});
}
/**
* Create a new API.
*
* See {@link #createOpenAPIWithHttpInfo}.
*
* @param parameters Optional parameters for the request.
* @return CreateOpenAPIResponse
* @throws ApiException if fails to make API call
*/
public CreateOpenAPIResponse createOpenAPI(CreateOpenAPIOptionalParameters parameters)
throws ApiException {
return createOpenAPIWithHttpInfo(parameters).getData();
}
/**
* Create a new API.
*
*
See {@link #createOpenAPIWithHttpInfoAsync}.
*
* @param parameters Optional parameters for the request.
* @return CompletableFuture<CreateOpenAPIResponse>
*/
public CompletableFuture createOpenAPIAsync(
CreateOpenAPIOptionalParameters parameters) {
return createOpenAPIWithHttpInfoAsync(parameters)
.thenApply(
response -> {
return response.getData();
});
}
/**
* Create a new API from the OpenAPI
* specification given. See the API
* Catalog documentation for additional information about the possible metadata. It returns
* the created API ID.
*
* @param parameters Optional parameters for the request.
* @return ApiResponse<CreateOpenAPIResponse>
* @throws ApiException if fails to make API call
* @http.response.details
*
* Response details
* Status Code Description Response Headers
* 201 API created successfully -
* 400 Bad request -
* 403 Forbidden -
* 429 Too many requests -
*
*/
public ApiResponse createOpenAPIWithHttpInfo(
CreateOpenAPIOptionalParameters parameters) throws ApiException {
// Check if unstable operation is enabled
String operationId = "createOpenAPI";
if (apiClient.isUnstableOperationEnabled("v2." + operationId)) {
apiClient.getLogger().warning(String.format("Using unstable operation '%s'", operationId));
} else {
throw new ApiException(0, String.format("Unstable operation '%s' is disabled", operationId));
}
Object localVarPostBody = null;
File openapiSpecFile = parameters.openapiSpecFile;
// create path and map variables
String localVarPath = "/api/v2/apicatalog/openapi";
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
if (openapiSpecFile != null) {
localVarFormParams.put("openapi_spec_file", openapiSpecFile);
}
Invocation.Builder builder =
apiClient.createBuilder(
"v2.ApiManagementApi.createOpenAPI",
localVarPath,
new ArrayList(),
localVarHeaderParams,
new HashMap(),
new String[] {"application/json"},
new String[] {"AuthZ", "apiKeyAuth", "appKeyAuth"});
return apiClient.invokeAPI(
"POST",
builder,
localVarHeaderParams,
new String[] {"multipart/form-data"},
localVarPostBody,
localVarFormParams,
false,
new GenericType() {});
}
/**
* Create a new API.
*
* See {@link #createOpenAPIWithHttpInfo}.
*
* @param parameters Optional parameters for the request.
* @return CompletableFuture<ApiResponse<CreateOpenAPIResponse>>
*/
public CompletableFuture> createOpenAPIWithHttpInfoAsync(
CreateOpenAPIOptionalParameters parameters) {
// Check if unstable operation is enabled
String operationId = "createOpenAPI";
if (apiClient.isUnstableOperationEnabled("v2." + operationId)) {
apiClient.getLogger().warning(String.format("Using unstable operation '%s'", operationId));
} else {
CompletableFuture> result = new CompletableFuture<>();
result.completeExceptionally(
new ApiException(0, String.format("Unstable operation '%s' is disabled", operationId)));
return result;
}
Object localVarPostBody = null;
File openapiSpecFile = parameters.openapiSpecFile;
// create path and map variables
String localVarPath = "/api/v2/apicatalog/openapi";
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
if (openapiSpecFile != null) {
localVarFormParams.put("openapi_spec_file", openapiSpecFile);
}
Invocation.Builder builder;
try {
builder =
apiClient.createBuilder(
"v2.ApiManagementApi.createOpenAPI",
localVarPath,
new ArrayList(),
localVarHeaderParams,
new HashMap(),
new String[] {"application/json"},
new String[] {"AuthZ", "apiKeyAuth", "appKeyAuth"});
} catch (ApiException ex) {
CompletableFuture> result = new CompletableFuture<>();
result.completeExceptionally(ex);
return result;
}
return apiClient.invokeAPIAsync(
"POST",
builder,
localVarHeaderParams,
new String[] {"multipart/form-data"},
localVarPostBody,
localVarFormParams,
false,
new GenericType() {});
}
/**
* Delete an API.
*
* See {@link #deleteOpenAPIWithHttpInfo}.
*
* @param id ID of the API to delete (required)
* @throws ApiException if fails to make API call
*/
public void deleteOpenAPI(UUID id) throws ApiException {
deleteOpenAPIWithHttpInfo(id);
}
/**
* Delete an API.
*
*
See {@link #deleteOpenAPIWithHttpInfoAsync}.
*
* @param id ID of the API to delete (required)
* @return CompletableFuture
*/
public CompletableFuture deleteOpenAPIAsync(UUID id) {
return deleteOpenAPIWithHttpInfoAsync(id)
.thenApply(
response -> {
return response.getData();
});
}
/**
* Delete a specific API by ID.
*
* @param id ID of the API to delete (required)
* @return ApiResponse<Void>
* @throws ApiException if fails to make API call
* @http.response.details
*
* Response details
* Status Code Description Response Headers
* 204 API deleted successfully -
* 400 Bad request -
* 403 Forbidden -
* 404 API not found error -
* 429 Too many requests -
*
*/
public ApiResponse deleteOpenAPIWithHttpInfo(UUID id) throws ApiException {
// Check if unstable operation is enabled
String operationId = "deleteOpenAPI";
if (apiClient.isUnstableOperationEnabled("v2." + operationId)) {
apiClient.getLogger().warning(String.format("Using unstable operation '%s'", operationId));
} else {
throw new ApiException(0, String.format("Unstable operation '%s' is disabled", operationId));
}
Object localVarPostBody = null;
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException(400, "Missing the required parameter 'id' when calling deleteOpenAPI");
}
// create path and map variables
String localVarPath =
"/api/v2/apicatalog/api/{id}"
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()));
Map localVarHeaderParams = new HashMap();
Invocation.Builder builder =
apiClient.createBuilder(
"v2.ApiManagementApi.deleteOpenAPI",
localVarPath,
new ArrayList(),
localVarHeaderParams,
new HashMap(),
new String[] {"*/*"},
new String[] {"AuthZ", "apiKeyAuth", "appKeyAuth"});
return apiClient.invokeAPI(
"DELETE",
builder,
localVarHeaderParams,
new String[] {},
localVarPostBody,
new HashMap(),
false,
null);
}
/**
* Delete an API.
*
* See {@link #deleteOpenAPIWithHttpInfo}.
*
* @param id ID of the API to delete (required)
* @return CompletableFuture<ApiResponse<Void>>
*/
public CompletableFuture> deleteOpenAPIWithHttpInfoAsync(UUID id) {
// Check if unstable operation is enabled
String operationId = "deleteOpenAPI";
if (apiClient.isUnstableOperationEnabled("v2." + operationId)) {
apiClient.getLogger().warning(String.format("Using unstable operation '%s'", operationId));
} else {
CompletableFuture> result = new CompletableFuture<>();
result.completeExceptionally(
new ApiException(0, String.format("Unstable operation '%s' is disabled", operationId)));
return result;
}
Object localVarPostBody = null;
// verify the required parameter 'id' is set
if (id == null) {
CompletableFuture> result = new CompletableFuture<>();
result.completeExceptionally(
new ApiException(400, "Missing the required parameter 'id' when calling deleteOpenAPI"));
return result;
}
// create path and map variables
String localVarPath =
"/api/v2/apicatalog/api/{id}"
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()));
Map localVarHeaderParams = new HashMap();
Invocation.Builder builder;
try {
builder =
apiClient.createBuilder(
"v2.ApiManagementApi.deleteOpenAPI",
localVarPath,
new ArrayList(),
localVarHeaderParams,
new HashMap(),
new String[] {"*/*"},
new String[] {"AuthZ", "apiKeyAuth", "appKeyAuth"});
} catch (ApiException ex) {
CompletableFuture> result = new CompletableFuture<>();
result.completeExceptionally(ex);
return result;
}
return apiClient.invokeAPIAsync(
"DELETE",
builder,
localVarHeaderParams,
new String[] {},
localVarPostBody,
new HashMap(),
false,
null);
}
/**
* Get an API.
*
* See {@link #getOpenAPIWithHttpInfo}.
*
* @param id ID of the API to retrieve (required)
* @return File
* @throws ApiException if fails to make API call
*/
public File getOpenAPI(UUID id) throws ApiException {
return getOpenAPIWithHttpInfo(id).getData();
}
/**
* Get an API.
*
*
See {@link #getOpenAPIWithHttpInfoAsync}.
*
* @param id ID of the API to retrieve (required)
* @return CompletableFuture<File>
*/
public CompletableFuture getOpenAPIAsync(UUID id) {
return getOpenAPIWithHttpInfoAsync(id)
.thenApply(
response -> {
return response.getData();
});
}
/**
* Retrieve information about a specific API in OpenAPI format file.
*
* @param id ID of the API to retrieve (required)
* @return ApiResponse<File>
* @throws ApiException if fails to make API call
* @http.response.details
*
* Response details
* Status Code Description Response Headers
* 200 OK -
* 400 Bad request -
* 403 Forbidden -
* 404 API not found error -
* 429 Too many requests -
*
*/
public ApiResponse getOpenAPIWithHttpInfo(UUID id) throws ApiException {
// Check if unstable operation is enabled
String operationId = "getOpenAPI";
if (apiClient.isUnstableOperationEnabled("v2." + operationId)) {
apiClient.getLogger().warning(String.format("Using unstable operation '%s'", operationId));
} else {
throw new ApiException(0, String.format("Unstable operation '%s' is disabled", operationId));
}
Object localVarPostBody = null;
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException(400, "Missing the required parameter 'id' when calling getOpenAPI");
}
// create path and map variables
String localVarPath =
"/api/v2/apicatalog/api/{id}/openapi"
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()));
Map localVarHeaderParams = new HashMap();
Invocation.Builder builder =
apiClient.createBuilder(
"v2.ApiManagementApi.getOpenAPI",
localVarPath,
new ArrayList(),
localVarHeaderParams,
new HashMap(),
new String[] {"multipart/form-data", "application/json"},
new String[] {"AuthZ", "apiKeyAuth", "appKeyAuth"});
return apiClient.invokeAPI(
"GET",
builder,
localVarHeaderParams,
new String[] {},
localVarPostBody,
new HashMap(),
false,
new GenericType() {});
}
/**
* Get an API.
*
* See {@link #getOpenAPIWithHttpInfo}.
*
* @param id ID of the API to retrieve (required)
* @return CompletableFuture<ApiResponse<File>>
*/
public CompletableFuture> getOpenAPIWithHttpInfoAsync(UUID id) {
// Check if unstable operation is enabled
String operationId = "getOpenAPI";
if (apiClient.isUnstableOperationEnabled("v2." + operationId)) {
apiClient.getLogger().warning(String.format("Using unstable operation '%s'", operationId));
} else {
CompletableFuture> result = new CompletableFuture<>();
result.completeExceptionally(
new ApiException(0, String.format("Unstable operation '%s' is disabled", operationId)));
return result;
}
Object localVarPostBody = null;
// verify the required parameter 'id' is set
if (id == null) {
CompletableFuture> result = new CompletableFuture<>();
result.completeExceptionally(
new ApiException(400, "Missing the required parameter 'id' when calling getOpenAPI"));
return result;
}
// create path and map variables
String localVarPath =
"/api/v2/apicatalog/api/{id}/openapi"
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()));
Map localVarHeaderParams = new HashMap();
Invocation.Builder builder;
try {
builder =
apiClient.createBuilder(
"v2.ApiManagementApi.getOpenAPI",
localVarPath,
new ArrayList(),
localVarHeaderParams,
new HashMap(),
new String[] {"multipart/form-data", "application/json"},
new String[] {"AuthZ", "apiKeyAuth", "appKeyAuth"});
} catch (ApiException ex) {
CompletableFuture> result = new CompletableFuture<>();
result.completeExceptionally(ex);
return result;
}
return apiClient.invokeAPIAsync(
"GET",
builder,
localVarHeaderParams,
new String[] {},
localVarPostBody,
new HashMap(),
false,
new GenericType() {});
}
/** Manage optional parameters to listAPIs. */
public static class ListAPIsOptionalParameters {
private String query;
private Long pageLimit;
private Long pageOffset;
/**
* Set query.
*
* @param query Filter APIs by name (optional)
* @return ListAPIsOptionalParameters
*/
public ListAPIsOptionalParameters query(String query) {
this.query = query;
return this;
}
/**
* Set pageLimit.
*
* @param pageLimit Number of items per page. (optional, default to 20)
* @return ListAPIsOptionalParameters
*/
public ListAPIsOptionalParameters pageLimit(Long pageLimit) {
this.pageLimit = pageLimit;
return this;
}
/**
* Set pageOffset.
*
* @param pageOffset Offset for pagination. (optional, default to 0)
* @return ListAPIsOptionalParameters
*/
public ListAPIsOptionalParameters pageOffset(Long pageOffset) {
this.pageOffset = pageOffset;
return this;
}
}
/**
* List APIs.
*
* See {@link #listAPIsWithHttpInfo}.
*
* @return ListAPIsResponse
* @throws ApiException if fails to make API call
*/
public ListAPIsResponse listAPIs() throws ApiException {
return listAPIsWithHttpInfo(new ListAPIsOptionalParameters()).getData();
}
/**
* List APIs.
*
*
See {@link #listAPIsWithHttpInfoAsync}.
*
* @return CompletableFuture<ListAPIsResponse>
*/
public CompletableFuture listAPIsAsync() {
return listAPIsWithHttpInfoAsync(new ListAPIsOptionalParameters())
.thenApply(
response -> {
return response.getData();
});
}
/**
* List APIs.
*
* See {@link #listAPIsWithHttpInfo}.
*
* @param parameters Optional parameters for the request.
* @return ListAPIsResponse
* @throws ApiException if fails to make API call
*/
public ListAPIsResponse listAPIs(ListAPIsOptionalParameters parameters) throws ApiException {
return listAPIsWithHttpInfo(parameters).getData();
}
/**
* List APIs.
*
*
See {@link #listAPIsWithHttpInfoAsync}.
*
* @param parameters Optional parameters for the request.
* @return CompletableFuture<ListAPIsResponse>
*/
public CompletableFuture listAPIsAsync(ListAPIsOptionalParameters parameters) {
return listAPIsWithHttpInfoAsync(parameters)
.thenApply(
response -> {
return response.getData();
});
}
/**
* List APIs and their IDs.
*
* @param parameters Optional parameters for the request.
* @return ApiResponse<ListAPIsResponse>
* @throws ApiException if fails to make API call
* @http.response.details
*
* Response details
* Status Code Description Response Headers
* 200 OK -
* 400 Bad request -
* 403 Forbidden -
* 429 Too many requests -
*
*/
public ApiResponse listAPIsWithHttpInfo(ListAPIsOptionalParameters parameters)
throws ApiException {
// Check if unstable operation is enabled
String operationId = "listAPIs";
if (apiClient.isUnstableOperationEnabled("v2." + operationId)) {
apiClient.getLogger().warning(String.format("Using unstable operation '%s'", operationId));
} else {
throw new ApiException(0, String.format("Unstable operation '%s' is disabled", operationId));
}
Object localVarPostBody = null;
String query = parameters.query;
Long pageLimit = parameters.pageLimit;
Long pageOffset = parameters.pageOffset;
// create path and map variables
String localVarPath = "/api/v2/apicatalog/api";
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPairs("", "query", query));
localVarQueryParams.addAll(apiClient.parameterToPairs("", "page[limit]", pageLimit));
localVarQueryParams.addAll(apiClient.parameterToPairs("", "page[offset]", pageOffset));
Invocation.Builder builder =
apiClient.createBuilder(
"v2.ApiManagementApi.listAPIs",
localVarPath,
localVarQueryParams,
localVarHeaderParams,
new HashMap(),
new String[] {"application/json"},
new String[] {"AuthZ", "apiKeyAuth", "appKeyAuth"});
return apiClient.invokeAPI(
"GET",
builder,
localVarHeaderParams,
new String[] {},
localVarPostBody,
new HashMap(),
false,
new GenericType() {});
}
/**
* List APIs.
*
* See {@link #listAPIsWithHttpInfo}.
*
* @param parameters Optional parameters for the request.
* @return CompletableFuture<ApiResponse<ListAPIsResponse>>
*/
public CompletableFuture> listAPIsWithHttpInfoAsync(
ListAPIsOptionalParameters parameters) {
// Check if unstable operation is enabled
String operationId = "listAPIs";
if (apiClient.isUnstableOperationEnabled("v2." + operationId)) {
apiClient.getLogger().warning(String.format("Using unstable operation '%s'", operationId));
} else {
CompletableFuture> result = new CompletableFuture<>();
result.completeExceptionally(
new ApiException(0, String.format("Unstable operation '%s' is disabled", operationId)));
return result;
}
Object localVarPostBody = null;
String query = parameters.query;
Long pageLimit = parameters.pageLimit;
Long pageOffset = parameters.pageOffset;
// create path and map variables
String localVarPath = "/api/v2/apicatalog/api";
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPairs("", "query", query));
localVarQueryParams.addAll(apiClient.parameterToPairs("", "page[limit]", pageLimit));
localVarQueryParams.addAll(apiClient.parameterToPairs("", "page[offset]", pageOffset));
Invocation.Builder builder;
try {
builder =
apiClient.createBuilder(
"v2.ApiManagementApi.listAPIs",
localVarPath,
localVarQueryParams,
localVarHeaderParams,
new HashMap(),
new String[] {"application/json"},
new String[] {"AuthZ", "apiKeyAuth", "appKeyAuth"});
} catch (ApiException ex) {
CompletableFuture> result = new CompletableFuture<>();
result.completeExceptionally(ex);
return result;
}
return apiClient.invokeAPIAsync(
"GET",
builder,
localVarHeaderParams,
new String[] {},
localVarPostBody,
new HashMap(),
false,
new GenericType() {});
}
/** Manage optional parameters to updateOpenAPI. */
public static class UpdateOpenAPIOptionalParameters {
private File openapiSpecFile;
/**
* Set openapiSpecFile.
*
* @param openapiSpecFile Binary OpenAPI
spec file (optional)
* @return UpdateOpenAPIOptionalParameters
*/
public UpdateOpenAPIOptionalParameters openapiSpecFile(File openapiSpecFile) {
this.openapiSpecFile = openapiSpecFile;
return this;
}
}
/**
* Update an API.
*
* See {@link #updateOpenAPIWithHttpInfo}.
*
* @param id ID of the API to modify (required)
* @return UpdateOpenAPIResponse
* @throws ApiException if fails to make API call
*/
public UpdateOpenAPIResponse updateOpenAPI(UUID id) throws ApiException {
return updateOpenAPIWithHttpInfo(id, new UpdateOpenAPIOptionalParameters()).getData();
}
/**
* Update an API.
*
*
See {@link #updateOpenAPIWithHttpInfoAsync}.
*
* @param id ID of the API to modify (required)
* @return CompletableFuture<UpdateOpenAPIResponse>
*/
public CompletableFuture updateOpenAPIAsync(UUID id) {
return updateOpenAPIWithHttpInfoAsync(id, new UpdateOpenAPIOptionalParameters())
.thenApply(
response -> {
return response.getData();
});
}
/**
* Update an API.
*
* See {@link #updateOpenAPIWithHttpInfo}.
*
* @param id ID of the API to modify (required)
* @param parameters Optional parameters for the request.
* @return UpdateOpenAPIResponse
* @throws ApiException if fails to make API call
*/
public UpdateOpenAPIResponse updateOpenAPI(UUID id, UpdateOpenAPIOptionalParameters parameters)
throws ApiException {
return updateOpenAPIWithHttpInfo(id, parameters).getData();
}
/**
* Update an API.
*
*
See {@link #updateOpenAPIWithHttpInfoAsync}.
*
* @param id ID of the API to modify (required)
* @param parameters Optional parameters for the request.
* @return CompletableFuture<UpdateOpenAPIResponse>
*/
public CompletableFuture updateOpenAPIAsync(
UUID id, UpdateOpenAPIOptionalParameters parameters) {
return updateOpenAPIWithHttpInfoAsync(id, parameters)
.thenApply(
response -> {
return response.getData();
});
}
/**
* Update information about a specific API. The given content will replace all API content of the
* given ID. The ID is returned by the create API, or can be found in the URL in the API catalog
* UI.
*
* @param id ID of the API to modify (required)
* @param parameters Optional parameters for the request.
* @return ApiResponse<UpdateOpenAPIResponse>
* @throws ApiException if fails to make API call
* @http.response.details
*
* Response details
* Status Code Description Response Headers
* 200 API updated successfully -
* 400 Bad request -
* 403 Forbidden -
* 404 API not found error -
* 429 Too many requests -
*
*/
public ApiResponse updateOpenAPIWithHttpInfo(
UUID id, UpdateOpenAPIOptionalParameters parameters) throws ApiException {
// Check if unstable operation is enabled
String operationId = "updateOpenAPI";
if (apiClient.isUnstableOperationEnabled("v2." + operationId)) {
apiClient.getLogger().warning(String.format("Using unstable operation '%s'", operationId));
} else {
throw new ApiException(0, String.format("Unstable operation '%s' is disabled", operationId));
}
Object localVarPostBody = null;
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException(400, "Missing the required parameter 'id' when calling updateOpenAPI");
}
File openapiSpecFile = parameters.openapiSpecFile;
// create path and map variables
String localVarPath =
"/api/v2/apicatalog/api/{id}/openapi"
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()));
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
if (openapiSpecFile != null) {
localVarFormParams.put("openapi_spec_file", openapiSpecFile);
}
Invocation.Builder builder =
apiClient.createBuilder(
"v2.ApiManagementApi.updateOpenAPI",
localVarPath,
new ArrayList(),
localVarHeaderParams,
new HashMap(),
new String[] {"application/json"},
new String[] {"AuthZ", "apiKeyAuth", "appKeyAuth"});
return apiClient.invokeAPI(
"PUT",
builder,
localVarHeaderParams,
new String[] {"multipart/form-data"},
localVarPostBody,
localVarFormParams,
false,
new GenericType() {});
}
/**
* Update an API.
*
* See {@link #updateOpenAPIWithHttpInfo}.
*
* @param id ID of the API to modify (required)
* @param parameters Optional parameters for the request.
* @return CompletableFuture<ApiResponse<UpdateOpenAPIResponse>>
*/
public CompletableFuture> updateOpenAPIWithHttpInfoAsync(
UUID id, UpdateOpenAPIOptionalParameters parameters) {
// Check if unstable operation is enabled
String operationId = "updateOpenAPI";
if (apiClient.isUnstableOperationEnabled("v2." + operationId)) {
apiClient.getLogger().warning(String.format("Using unstable operation '%s'", operationId));
} else {
CompletableFuture> result = new CompletableFuture<>();
result.completeExceptionally(
new ApiException(0, String.format("Unstable operation '%s' is disabled", operationId)));
return result;
}
Object localVarPostBody = null;
// verify the required parameter 'id' is set
if (id == null) {
CompletableFuture> result = new CompletableFuture<>();
result.completeExceptionally(
new ApiException(400, "Missing the required parameter 'id' when calling updateOpenAPI"));
return result;
}
File openapiSpecFile = parameters.openapiSpecFile;
// create path and map variables
String localVarPath =
"/api/v2/apicatalog/api/{id}/openapi"
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()));
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
if (openapiSpecFile != null) {
localVarFormParams.put("openapi_spec_file", openapiSpecFile);
}
Invocation.Builder builder;
try {
builder =
apiClient.createBuilder(
"v2.ApiManagementApi.updateOpenAPI",
localVarPath,
new ArrayList(),
localVarHeaderParams,
new HashMap(),
new String[] {"application/json"},
new String[] {"AuthZ", "apiKeyAuth", "appKeyAuth"});
} catch (ApiException ex) {
CompletableFuture> result = new CompletableFuture<>();
result.completeExceptionally(ex);
return result;
}
return apiClient.invokeAPIAsync(
"PUT",
builder,
localVarHeaderParams,
new String[] {"multipart/form-data"},
localVarPostBody,
localVarFormParams,
false,
new GenericType() {});
}
}