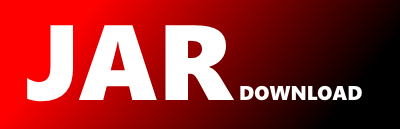
com.datadog.api.client.v2.api.AwsLogsIntegrationApi Maven / Gradle / Ivy
package com.datadog.api.client.v2.api;
import com.datadog.api.client.ApiClient;
import com.datadog.api.client.ApiException;
import com.datadog.api.client.ApiResponse;
import com.datadog.api.client.Pair;
import com.datadog.api.client.v2.model.AWSLogsServicesResponse;
import jakarta.ws.rs.client.Invocation;
import jakarta.ws.rs.core.GenericType;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.Map;
import java.util.concurrent.CompletableFuture;
@jakarta.annotation.Generated(
value = "https://github.com/DataDog/datadog-api-client-java/blob/master/.generator")
public class AwsLogsIntegrationApi {
private ApiClient apiClient;
public AwsLogsIntegrationApi() {
this(ApiClient.getDefaultApiClient());
}
public AwsLogsIntegrationApi(ApiClient apiClient) {
this.apiClient = apiClient;
}
/**
* Get the API client.
*
* @return API client
*/
public ApiClient getApiClient() {
return apiClient;
}
/**
* Set the API client.
*
* @param apiClient an instance of API client
*/
public void setApiClient(ApiClient apiClient) {
this.apiClient = apiClient;
}
/**
* Get list of AWS log ready services.
*
* See {@link #listAWSLogsServicesWithHttpInfo}.
*
* @return AWSLogsServicesResponse
* @throws ApiException if fails to make API call
*/
public AWSLogsServicesResponse listAWSLogsServices() throws ApiException {
return listAWSLogsServicesWithHttpInfo().getData();
}
/**
* Get list of AWS log ready services.
*
*
See {@link #listAWSLogsServicesWithHttpInfoAsync}.
*
* @return CompletableFuture<AWSLogsServicesResponse>
*/
public CompletableFuture listAWSLogsServicesAsync() {
return listAWSLogsServicesWithHttpInfoAsync()
.thenApply(
response -> {
return response.getData();
});
}
/**
* Get a list of AWS services that can send logs to Datadog.
*
* @return ApiResponse<AWSLogsServicesResponse>
* @throws ApiException if fails to make API call
* @http.response.details
*
* Response details
* Status Code Description Response Headers
* 200 AWS Logs Services List object -
* 403 Forbidden -
* 429 Too many requests -
*
*/
public ApiResponse listAWSLogsServicesWithHttpInfo()
throws ApiException {
// Check if unstable operation is enabled
String operationId = "listAWSLogsServices";
if (apiClient.isUnstableOperationEnabled("v2." + operationId)) {
apiClient.getLogger().warning(String.format("Using unstable operation '%s'", operationId));
} else {
throw new ApiException(0, String.format("Unstable operation '%s' is disabled", operationId));
}
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/api/v2/integration/aws/logs/services";
Map localVarHeaderParams = new HashMap();
Invocation.Builder builder =
apiClient.createBuilder(
"v2.AwsLogsIntegrationApi.listAWSLogsServices",
localVarPath,
new ArrayList(),
localVarHeaderParams,
new HashMap(),
new String[] {"application/json"},
new String[] {"apiKeyAuth", "appKeyAuth"});
return apiClient.invokeAPI(
"GET",
builder,
localVarHeaderParams,
new String[] {},
localVarPostBody,
new HashMap(),
false,
new GenericType() {});
}
/**
* Get list of AWS log ready services.
*
* See {@link #listAWSLogsServicesWithHttpInfo}.
*
* @return CompletableFuture<ApiResponse<AWSLogsServicesResponse>>
*/
public CompletableFuture>
listAWSLogsServicesWithHttpInfoAsync() {
// Check if unstable operation is enabled
String operationId = "listAWSLogsServices";
if (apiClient.isUnstableOperationEnabled("v2." + operationId)) {
apiClient.getLogger().warning(String.format("Using unstable operation '%s'", operationId));
} else {
CompletableFuture> result = new CompletableFuture<>();
result.completeExceptionally(
new ApiException(0, String.format("Unstable operation '%s' is disabled", operationId)));
return result;
}
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/api/v2/integration/aws/logs/services";
Map localVarHeaderParams = new HashMap();
Invocation.Builder builder;
try {
builder =
apiClient.createBuilder(
"v2.AwsLogsIntegrationApi.listAWSLogsServices",
localVarPath,
new ArrayList(),
localVarHeaderParams,
new HashMap(),
new String[] {"application/json"},
new String[] {"apiKeyAuth", "appKeyAuth"});
} catch (ApiException ex) {
CompletableFuture> result = new CompletableFuture<>();
result.completeExceptionally(ex);
return result;
}
return apiClient.invokeAPIAsync(
"GET",
builder,
localVarHeaderParams,
new String[] {},
localVarPostBody,
new HashMap(),
false,
new GenericType() {});
}
}