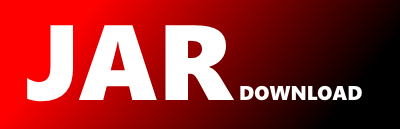
com.datadog.api.client.v2.api.OktaIntegrationApi Maven / Gradle / Ivy
package com.datadog.api.client.v2.api;
import com.datadog.api.client.ApiClient;
import com.datadog.api.client.ApiException;
import com.datadog.api.client.ApiResponse;
import com.datadog.api.client.Pair;
import com.datadog.api.client.v2.model.OktaAccountRequest;
import com.datadog.api.client.v2.model.OktaAccountResponse;
import com.datadog.api.client.v2.model.OktaAccountUpdateRequest;
import com.datadog.api.client.v2.model.OktaAccountsResponse;
import jakarta.ws.rs.client.Invocation;
import jakarta.ws.rs.core.GenericType;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.Map;
import java.util.concurrent.CompletableFuture;
@jakarta.annotation.Generated(
value = "https://github.com/DataDog/datadog-api-client-java/blob/master/.generator")
public class OktaIntegrationApi {
private ApiClient apiClient;
public OktaIntegrationApi() {
this(ApiClient.getDefaultApiClient());
}
public OktaIntegrationApi(ApiClient apiClient) {
this.apiClient = apiClient;
}
/**
* Get the API client.
*
* @return API client
*/
public ApiClient getApiClient() {
return apiClient;
}
/**
* Set the API client.
*
* @param apiClient an instance of API client
*/
public void setApiClient(ApiClient apiClient) {
this.apiClient = apiClient;
}
/**
* Add Okta account.
*
* See {@link #createOktaAccountWithHttpInfo}.
*
* @param body (required)
* @return OktaAccountResponse
* @throws ApiException if fails to make API call
*/
public OktaAccountResponse createOktaAccount(OktaAccountRequest body) throws ApiException {
return createOktaAccountWithHttpInfo(body).getData();
}
/**
* Add Okta account.
*
*
See {@link #createOktaAccountWithHttpInfoAsync}.
*
* @param body (required)
* @return CompletableFuture<OktaAccountResponse>
*/
public CompletableFuture createOktaAccountAsync(OktaAccountRequest body) {
return createOktaAccountWithHttpInfoAsync(body)
.thenApply(
response -> {
return response.getData();
});
}
/**
* Create an Okta account.
*
* @param body (required)
* @return ApiResponse<OktaAccountResponse>
* @throws ApiException if fails to make API call
* @http.response.details
*
* Response details
* Status Code Description Response Headers
* 201 OK -
* 400 Bad Request -
* 403 Forbidden -
* 404 Not Found -
* 429 Too many requests -
*
*/
public ApiResponse createOktaAccountWithHttpInfo(OktaAccountRequest body)
throws ApiException {
Object localVarPostBody = body;
// verify the required parameter 'body' is set
if (body == null) {
throw new ApiException(
400, "Missing the required parameter 'body' when calling createOktaAccount");
}
// create path and map variables
String localVarPath = "/api/v2/integrations/okta/accounts";
Map localVarHeaderParams = new HashMap();
Invocation.Builder builder =
apiClient.createBuilder(
"v2.OktaIntegrationApi.createOktaAccount",
localVarPath,
new ArrayList(),
localVarHeaderParams,
new HashMap(),
new String[] {"application/json"},
new String[] {"apiKeyAuth", "appKeyAuth"});
return apiClient.invokeAPI(
"POST",
builder,
localVarHeaderParams,
new String[] {"application/json"},
localVarPostBody,
new HashMap(),
false,
new GenericType() {});
}
/**
* Add Okta account.
*
* See {@link #createOktaAccountWithHttpInfo}.
*
* @param body (required)
* @return CompletableFuture<ApiResponse<OktaAccountResponse>>
*/
public CompletableFuture> createOktaAccountWithHttpInfoAsync(
OktaAccountRequest body) {
Object localVarPostBody = body;
// verify the required parameter 'body' is set
if (body == null) {
CompletableFuture> result = new CompletableFuture<>();
result.completeExceptionally(
new ApiException(
400, "Missing the required parameter 'body' when calling createOktaAccount"));
return result;
}
// create path and map variables
String localVarPath = "/api/v2/integrations/okta/accounts";
Map localVarHeaderParams = new HashMap();
Invocation.Builder builder;
try {
builder =
apiClient.createBuilder(
"v2.OktaIntegrationApi.createOktaAccount",
localVarPath,
new ArrayList(),
localVarHeaderParams,
new HashMap(),
new String[] {"application/json"},
new String[] {"apiKeyAuth", "appKeyAuth"});
} catch (ApiException ex) {
CompletableFuture> result = new CompletableFuture<>();
result.completeExceptionally(ex);
return result;
}
return apiClient.invokeAPIAsync(
"POST",
builder,
localVarHeaderParams,
new String[] {"application/json"},
localVarPostBody,
new HashMap(),
false,
new GenericType() {});
}
/**
* Delete Okta account.
*
* See {@link #deleteOktaAccountWithHttpInfo}.
*
* @param accountId None (required)
* @throws ApiException if fails to make API call
*/
public void deleteOktaAccount(String accountId) throws ApiException {
deleteOktaAccountWithHttpInfo(accountId);
}
/**
* Delete Okta account.
*
*
See {@link #deleteOktaAccountWithHttpInfoAsync}.
*
* @param accountId None (required)
* @return CompletableFuture
*/
public CompletableFuture deleteOktaAccountAsync(String accountId) {
return deleteOktaAccountWithHttpInfoAsync(accountId)
.thenApply(
response -> {
return response.getData();
});
}
/**
* Delete an Okta account.
*
* @param accountId None (required)
* @return ApiResponse<Void>
* @throws ApiException if fails to make API call
* @http.response.details
*
* Response details
* Status Code Description Response Headers
* 204 OK -
* 400 Bad Request -
* 403 Forbidden -
* 404 Not Found -
* 429 Too many requests -
*
*/
public ApiResponse deleteOktaAccountWithHttpInfo(String accountId) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'accountId' is set
if (accountId == null) {
throw new ApiException(
400, "Missing the required parameter 'accountId' when calling deleteOktaAccount");
}
// create path and map variables
String localVarPath =
"/api/v2/integrations/okta/accounts/{account_id}"
.replaceAll("\\{" + "account_id" + "\\}", apiClient.escapeString(accountId.toString()));
Map localVarHeaderParams = new HashMap();
Invocation.Builder builder =
apiClient.createBuilder(
"v2.OktaIntegrationApi.deleteOktaAccount",
localVarPath,
new ArrayList(),
localVarHeaderParams,
new HashMap(),
new String[] {"*/*"},
new String[] {"apiKeyAuth", "appKeyAuth"});
return apiClient.invokeAPI(
"DELETE",
builder,
localVarHeaderParams,
new String[] {},
localVarPostBody,
new HashMap(),
false,
null);
}
/**
* Delete Okta account.
*
* See {@link #deleteOktaAccountWithHttpInfo}.
*
* @param accountId None (required)
* @return CompletableFuture<ApiResponse<Void>>
*/
public CompletableFuture> deleteOktaAccountWithHttpInfoAsync(String accountId) {
Object localVarPostBody = null;
// verify the required parameter 'accountId' is set
if (accountId == null) {
CompletableFuture> result = new CompletableFuture<>();
result.completeExceptionally(
new ApiException(
400, "Missing the required parameter 'accountId' when calling deleteOktaAccount"));
return result;
}
// create path and map variables
String localVarPath =
"/api/v2/integrations/okta/accounts/{account_id}"
.replaceAll("\\{" + "account_id" + "\\}", apiClient.escapeString(accountId.toString()));
Map localVarHeaderParams = new HashMap();
Invocation.Builder builder;
try {
builder =
apiClient.createBuilder(
"v2.OktaIntegrationApi.deleteOktaAccount",
localVarPath,
new ArrayList(),
localVarHeaderParams,
new HashMap(),
new String[] {"*/*"},
new String[] {"apiKeyAuth", "appKeyAuth"});
} catch (ApiException ex) {
CompletableFuture> result = new CompletableFuture<>();
result.completeExceptionally(ex);
return result;
}
return apiClient.invokeAPIAsync(
"DELETE",
builder,
localVarHeaderParams,
new String[] {},
localVarPostBody,
new HashMap(),
false,
null);
}
/**
* Get Okta account.
*
* See {@link #getOktaAccountWithHttpInfo}.
*
* @param accountId None (required)
* @return OktaAccountResponse
* @throws ApiException if fails to make API call
*/
public OktaAccountResponse getOktaAccount(String accountId) throws ApiException {
return getOktaAccountWithHttpInfo(accountId).getData();
}
/**
* Get Okta account.
*
*
See {@link #getOktaAccountWithHttpInfoAsync}.
*
* @param accountId None (required)
* @return CompletableFuture<OktaAccountResponse>
*/
public CompletableFuture getOktaAccountAsync(String accountId) {
return getOktaAccountWithHttpInfoAsync(accountId)
.thenApply(
response -> {
return response.getData();
});
}
/**
* Get an Okta account.
*
* @param accountId None (required)
* @return ApiResponse<OktaAccountResponse>
* @throws ApiException if fails to make API call
* @http.response.details
*
* Response details
* Status Code Description Response Headers
* 200 OK -
* 400 Bad Request -
* 403 Forbidden -
* 404 Not Found -
* 429 Too many requests -
*
*/
public ApiResponse getOktaAccountWithHttpInfo(String accountId)
throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'accountId' is set
if (accountId == null) {
throw new ApiException(
400, "Missing the required parameter 'accountId' when calling getOktaAccount");
}
// create path and map variables
String localVarPath =
"/api/v2/integrations/okta/accounts/{account_id}"
.replaceAll("\\{" + "account_id" + "\\}", apiClient.escapeString(accountId.toString()));
Map localVarHeaderParams = new HashMap();
Invocation.Builder builder =
apiClient.createBuilder(
"v2.OktaIntegrationApi.getOktaAccount",
localVarPath,
new ArrayList(),
localVarHeaderParams,
new HashMap(),
new String[] {"application/json"},
new String[] {"apiKeyAuth", "appKeyAuth"});
return apiClient.invokeAPI(
"GET",
builder,
localVarHeaderParams,
new String[] {},
localVarPostBody,
new HashMap(),
false,
new GenericType() {});
}
/**
* Get Okta account.
*
* See {@link #getOktaAccountWithHttpInfo}.
*
* @param accountId None (required)
* @return CompletableFuture<ApiResponse<OktaAccountResponse>>
*/
public CompletableFuture> getOktaAccountWithHttpInfoAsync(
String accountId) {
Object localVarPostBody = null;
// verify the required parameter 'accountId' is set
if (accountId == null) {
CompletableFuture> result = new CompletableFuture<>();
result.completeExceptionally(
new ApiException(
400, "Missing the required parameter 'accountId' when calling getOktaAccount"));
return result;
}
// create path and map variables
String localVarPath =
"/api/v2/integrations/okta/accounts/{account_id}"
.replaceAll("\\{" + "account_id" + "\\}", apiClient.escapeString(accountId.toString()));
Map localVarHeaderParams = new HashMap();
Invocation.Builder builder;
try {
builder =
apiClient.createBuilder(
"v2.OktaIntegrationApi.getOktaAccount",
localVarPath,
new ArrayList(),
localVarHeaderParams,
new HashMap(),
new String[] {"application/json"},
new String[] {"apiKeyAuth", "appKeyAuth"});
} catch (ApiException ex) {
CompletableFuture> result = new CompletableFuture<>();
result.completeExceptionally(ex);
return result;
}
return apiClient.invokeAPIAsync(
"GET",
builder,
localVarHeaderParams,
new String[] {},
localVarPostBody,
new HashMap(),
false,
new GenericType() {});
}
/**
* List Okta accounts.
*
* See {@link #listOktaAccountsWithHttpInfo}.
*
* @return OktaAccountsResponse
* @throws ApiException if fails to make API call
*/
public OktaAccountsResponse listOktaAccounts() throws ApiException {
return listOktaAccountsWithHttpInfo().getData();
}
/**
* List Okta accounts.
*
*
See {@link #listOktaAccountsWithHttpInfoAsync}.
*
* @return CompletableFuture<OktaAccountsResponse>
*/
public CompletableFuture listOktaAccountsAsync() {
return listOktaAccountsWithHttpInfoAsync()
.thenApply(
response -> {
return response.getData();
});
}
/**
* List Okta accounts.
*
* @return ApiResponse<OktaAccountsResponse>
* @throws ApiException if fails to make API call
* @http.response.details
*
* Response details
* Status Code Description Response Headers
* 200 OK -
* 400 Bad Request -
* 403 Forbidden -
* 404 Not Found -
* 429 Too many requests -
*
*/
public ApiResponse listOktaAccountsWithHttpInfo() throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/api/v2/integrations/okta/accounts";
Map localVarHeaderParams = new HashMap();
Invocation.Builder builder =
apiClient.createBuilder(
"v2.OktaIntegrationApi.listOktaAccounts",
localVarPath,
new ArrayList(),
localVarHeaderParams,
new HashMap(),
new String[] {"application/json"},
new String[] {"apiKeyAuth", "appKeyAuth"});
return apiClient.invokeAPI(
"GET",
builder,
localVarHeaderParams,
new String[] {},
localVarPostBody,
new HashMap(),
false,
new GenericType() {});
}
/**
* List Okta accounts.
*
* See {@link #listOktaAccountsWithHttpInfo}.
*
* @return CompletableFuture<ApiResponse<OktaAccountsResponse>>
*/
public CompletableFuture> listOktaAccountsWithHttpInfoAsync() {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/api/v2/integrations/okta/accounts";
Map localVarHeaderParams = new HashMap();
Invocation.Builder builder;
try {
builder =
apiClient.createBuilder(
"v2.OktaIntegrationApi.listOktaAccounts",
localVarPath,
new ArrayList(),
localVarHeaderParams,
new HashMap(),
new String[] {"application/json"},
new String[] {"apiKeyAuth", "appKeyAuth"});
} catch (ApiException ex) {
CompletableFuture> result = new CompletableFuture<>();
result.completeExceptionally(ex);
return result;
}
return apiClient.invokeAPIAsync(
"GET",
builder,
localVarHeaderParams,
new String[] {},
localVarPostBody,
new HashMap(),
false,
new GenericType() {});
}
/**
* Update Okta account.
*
* See {@link #updateOktaAccountWithHttpInfo}.
*
* @param accountId None (required)
* @param body (required)
* @return OktaAccountResponse
* @throws ApiException if fails to make API call
*/
public OktaAccountResponse updateOktaAccount(String accountId, OktaAccountUpdateRequest body)
throws ApiException {
return updateOktaAccountWithHttpInfo(accountId, body).getData();
}
/**
* Update Okta account.
*
*
See {@link #updateOktaAccountWithHttpInfoAsync}.
*
* @param accountId None (required)
* @param body (required)
* @return CompletableFuture<OktaAccountResponse>
*/
public CompletableFuture updateOktaAccountAsync(
String accountId, OktaAccountUpdateRequest body) {
return updateOktaAccountWithHttpInfoAsync(accountId, body)
.thenApply(
response -> {
return response.getData();
});
}
/**
* Update an Okta account.
*
* @param accountId None (required)
* @param body (required)
* @return ApiResponse<OktaAccountResponse>
* @throws ApiException if fails to make API call
* @http.response.details
*
* Response details
* Status Code Description Response Headers
* 200 OK -
* 400 Bad Request -
* 403 Forbidden -
* 404 Not Found -
* 429 Too many requests -
*
*/
public ApiResponse updateOktaAccountWithHttpInfo(
String accountId, OktaAccountUpdateRequest body) throws ApiException {
Object localVarPostBody = body;
// verify the required parameter 'accountId' is set
if (accountId == null) {
throw new ApiException(
400, "Missing the required parameter 'accountId' when calling updateOktaAccount");
}
// verify the required parameter 'body' is set
if (body == null) {
throw new ApiException(
400, "Missing the required parameter 'body' when calling updateOktaAccount");
}
// create path and map variables
String localVarPath =
"/api/v2/integrations/okta/accounts/{account_id}"
.replaceAll("\\{" + "account_id" + "\\}", apiClient.escapeString(accountId.toString()));
Map localVarHeaderParams = new HashMap();
Invocation.Builder builder =
apiClient.createBuilder(
"v2.OktaIntegrationApi.updateOktaAccount",
localVarPath,
new ArrayList(),
localVarHeaderParams,
new HashMap(),
new String[] {"application/json"},
new String[] {"apiKeyAuth", "appKeyAuth"});
return apiClient.invokeAPI(
"PATCH",
builder,
localVarHeaderParams,
new String[] {"application/json"},
localVarPostBody,
new HashMap(),
false,
new GenericType() {});
}
/**
* Update Okta account.
*
* See {@link #updateOktaAccountWithHttpInfo}.
*
* @param accountId None (required)
* @param body (required)
* @return CompletableFuture<ApiResponse<OktaAccountResponse>>
*/
public CompletableFuture> updateOktaAccountWithHttpInfoAsync(
String accountId, OktaAccountUpdateRequest body) {
Object localVarPostBody = body;
// verify the required parameter 'accountId' is set
if (accountId == null) {
CompletableFuture> result = new CompletableFuture<>();
result.completeExceptionally(
new ApiException(
400, "Missing the required parameter 'accountId' when calling updateOktaAccount"));
return result;
}
// verify the required parameter 'body' is set
if (body == null) {
CompletableFuture> result = new CompletableFuture<>();
result.completeExceptionally(
new ApiException(
400, "Missing the required parameter 'body' when calling updateOktaAccount"));
return result;
}
// create path and map variables
String localVarPath =
"/api/v2/integrations/okta/accounts/{account_id}"
.replaceAll("\\{" + "account_id" + "\\}", apiClient.escapeString(accountId.toString()));
Map localVarHeaderParams = new HashMap();
Invocation.Builder builder;
try {
builder =
apiClient.createBuilder(
"v2.OktaIntegrationApi.updateOktaAccount",
localVarPath,
new ArrayList(),
localVarHeaderParams,
new HashMap(),
new String[] {"application/json"},
new String[] {"apiKeyAuth", "appKeyAuth"});
} catch (ApiException ex) {
CompletableFuture> result = new CompletableFuture<>();
result.completeExceptionally(ex);
return result;
}
return apiClient.invokeAPIAsync(
"PATCH",
builder,
localVarHeaderParams,
new String[] {"application/json"},
localVarPostBody,
new HashMap(),
false,
new GenericType() {});
}
}