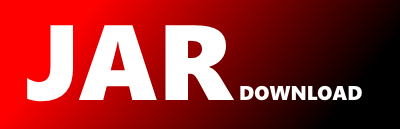
com.datadog.api.client.v2.api.PowerpackApi Maven / Gradle / Ivy
package com.datadog.api.client.v2.api;
import com.datadog.api.client.ApiClient;
import com.datadog.api.client.ApiException;
import com.datadog.api.client.ApiResponse;
import com.datadog.api.client.PaginationIterable;
import com.datadog.api.client.Pair;
import com.datadog.api.client.v2.model.ListPowerpacksResponse;
import com.datadog.api.client.v2.model.Powerpack;
import com.datadog.api.client.v2.model.PowerpackData;
import com.datadog.api.client.v2.model.PowerpackResponse;
import jakarta.ws.rs.client.Invocation;
import jakarta.ws.rs.core.GenericType;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import java.util.concurrent.CompletableFuture;
@jakarta.annotation.Generated(
value = "https://github.com/DataDog/datadog-api-client-java/blob/master/.generator")
public class PowerpackApi {
private ApiClient apiClient;
public PowerpackApi() {
this(ApiClient.getDefaultApiClient());
}
public PowerpackApi(ApiClient apiClient) {
this.apiClient = apiClient;
}
/**
* Get the API client.
*
* @return API client
*/
public ApiClient getApiClient() {
return apiClient;
}
/**
* Set the API client.
*
* @param apiClient an instance of API client
*/
public void setApiClient(ApiClient apiClient) {
this.apiClient = apiClient;
}
/**
* Create a new powerpack.
*
* See {@link #createPowerpackWithHttpInfo}.
*
* @param body Create a powerpack request body. (required)
* @return PowerpackResponse
* @throws ApiException if fails to make API call
*/
public PowerpackResponse createPowerpack(Powerpack body) throws ApiException {
return createPowerpackWithHttpInfo(body).getData();
}
/**
* Create a new powerpack.
*
*
See {@link #createPowerpackWithHttpInfoAsync}.
*
* @param body Create a powerpack request body. (required)
* @return CompletableFuture<PowerpackResponse>
*/
public CompletableFuture createPowerpackAsync(Powerpack body) {
return createPowerpackWithHttpInfoAsync(body)
.thenApply(
response -> {
return response.getData();
});
}
/**
* Create a powerpack.
*
* @param body Create a powerpack request body. (required)
* @return ApiResponse<PowerpackResponse>
* @throws ApiException if fails to make API call
* @http.response.details
*
* Response details
* Status Code Description Response Headers
* 200 OK -
* 400 Bad Request -
* 429 Too many requests -
*
*/
public ApiResponse createPowerpackWithHttpInfo(Powerpack body)
throws ApiException {
Object localVarPostBody = body;
// verify the required parameter 'body' is set
if (body == null) {
throw new ApiException(
400, "Missing the required parameter 'body' when calling createPowerpack");
}
// create path and map variables
String localVarPath = "/api/v2/powerpacks";
Map localVarHeaderParams = new HashMap();
Invocation.Builder builder =
apiClient.createBuilder(
"v2.PowerpackApi.createPowerpack",
localVarPath,
new ArrayList(),
localVarHeaderParams,
new HashMap(),
new String[] {"application/json"},
new String[] {"AuthZ", "apiKeyAuth", "appKeyAuth"});
return apiClient.invokeAPI(
"POST",
builder,
localVarHeaderParams,
new String[] {"application/json"},
localVarPostBody,
new HashMap(),
false,
new GenericType() {});
}
/**
* Create a new powerpack.
*
* See {@link #createPowerpackWithHttpInfo}.
*
* @param body Create a powerpack request body. (required)
* @return CompletableFuture<ApiResponse<PowerpackResponse>>
*/
public CompletableFuture> createPowerpackWithHttpInfoAsync(
Powerpack body) {
Object localVarPostBody = body;
// verify the required parameter 'body' is set
if (body == null) {
CompletableFuture> result = new CompletableFuture<>();
result.completeExceptionally(
new ApiException(
400, "Missing the required parameter 'body' when calling createPowerpack"));
return result;
}
// create path and map variables
String localVarPath = "/api/v2/powerpacks";
Map localVarHeaderParams = new HashMap();
Invocation.Builder builder;
try {
builder =
apiClient.createBuilder(
"v2.PowerpackApi.createPowerpack",
localVarPath,
new ArrayList(),
localVarHeaderParams,
new HashMap(),
new String[] {"application/json"},
new String[] {"AuthZ", "apiKeyAuth", "appKeyAuth"});
} catch (ApiException ex) {
CompletableFuture> result = new CompletableFuture<>();
result.completeExceptionally(ex);
return result;
}
return apiClient.invokeAPIAsync(
"POST",
builder,
localVarHeaderParams,
new String[] {"application/json"},
localVarPostBody,
new HashMap(),
false,
new GenericType() {});
}
/**
* Delete a powerpack.
*
* See {@link #deletePowerpackWithHttpInfo}.
*
* @param powerpackId Powerpack id (required)
* @throws ApiException if fails to make API call
*/
public void deletePowerpack(String powerpackId) throws ApiException {
deletePowerpackWithHttpInfo(powerpackId);
}
/**
* Delete a powerpack.
*
*
See {@link #deletePowerpackWithHttpInfoAsync}.
*
* @param powerpackId Powerpack id (required)
* @return CompletableFuture
*/
public CompletableFuture deletePowerpackAsync(String powerpackId) {
return deletePowerpackWithHttpInfoAsync(powerpackId)
.thenApply(
response -> {
return response.getData();
});
}
/**
* Delete a powerpack.
*
* @param powerpackId Powerpack id (required)
* @return ApiResponse<Void>
* @throws ApiException if fails to make API call
* @http.response.details
*
* Response details
* Status Code Description Response Headers
* 204 OK -
* 404 Powerpack Not Found -
* 429 Too many requests -
*
*/
public ApiResponse deletePowerpackWithHttpInfo(String powerpackId) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'powerpackId' is set
if (powerpackId == null) {
throw new ApiException(
400, "Missing the required parameter 'powerpackId' when calling deletePowerpack");
}
// create path and map variables
String localVarPath =
"/api/v2/powerpacks/{powerpack_id}"
.replaceAll(
"\\{" + "powerpack_id" + "\\}", apiClient.escapeString(powerpackId.toString()));
Map localVarHeaderParams = new HashMap();
Invocation.Builder builder =
apiClient.createBuilder(
"v2.PowerpackApi.deletePowerpack",
localVarPath,
new ArrayList(),
localVarHeaderParams,
new HashMap(),
new String[] {"*/*"},
new String[] {"AuthZ", "apiKeyAuth", "appKeyAuth"});
return apiClient.invokeAPI(
"DELETE",
builder,
localVarHeaderParams,
new String[] {},
localVarPostBody,
new HashMap(),
false,
null);
}
/**
* Delete a powerpack.
*
* See {@link #deletePowerpackWithHttpInfo}.
*
* @param powerpackId Powerpack id (required)
* @return CompletableFuture<ApiResponse<Void>>
*/
public CompletableFuture> deletePowerpackWithHttpInfoAsync(String powerpackId) {
Object localVarPostBody = null;
// verify the required parameter 'powerpackId' is set
if (powerpackId == null) {
CompletableFuture> result = new CompletableFuture<>();
result.completeExceptionally(
new ApiException(
400, "Missing the required parameter 'powerpackId' when calling deletePowerpack"));
return result;
}
// create path and map variables
String localVarPath =
"/api/v2/powerpacks/{powerpack_id}"
.replaceAll(
"\\{" + "powerpack_id" + "\\}", apiClient.escapeString(powerpackId.toString()));
Map localVarHeaderParams = new HashMap();
Invocation.Builder builder;
try {
builder =
apiClient.createBuilder(
"v2.PowerpackApi.deletePowerpack",
localVarPath,
new ArrayList(),
localVarHeaderParams,
new HashMap(),
new String[] {"*/*"},
new String[] {"AuthZ", "apiKeyAuth", "appKeyAuth"});
} catch (ApiException ex) {
CompletableFuture> result = new CompletableFuture<>();
result.completeExceptionally(ex);
return result;
}
return apiClient.invokeAPIAsync(
"DELETE",
builder,
localVarHeaderParams,
new String[] {},
localVarPostBody,
new HashMap(),
false,
null);
}
/**
* Get a Powerpack.
*
* See {@link #getPowerpackWithHttpInfo}.
*
* @param powerpackId ID of the powerpack. (required)
* @return PowerpackResponse
* @throws ApiException if fails to make API call
*/
public PowerpackResponse getPowerpack(String powerpackId) throws ApiException {
return getPowerpackWithHttpInfo(powerpackId).getData();
}
/**
* Get a Powerpack.
*
*
See {@link #getPowerpackWithHttpInfoAsync}.
*
* @param powerpackId ID of the powerpack. (required)
* @return CompletableFuture<PowerpackResponse>
*/
public CompletableFuture getPowerpackAsync(String powerpackId) {
return getPowerpackWithHttpInfoAsync(powerpackId)
.thenApply(
response -> {
return response.getData();
});
}
/**
* Get a powerpack.
*
* @param powerpackId ID of the powerpack. (required)
* @return ApiResponse<PowerpackResponse>
* @throws ApiException if fails to make API call
* @http.response.details
*
* Response details
* Status Code Description Response Headers
* 200 OK -
* 404 Powerpack Not Found. -
* 429 Too many requests -
*
*/
public ApiResponse getPowerpackWithHttpInfo(String powerpackId)
throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'powerpackId' is set
if (powerpackId == null) {
throw new ApiException(
400, "Missing the required parameter 'powerpackId' when calling getPowerpack");
}
// create path and map variables
String localVarPath =
"/api/v2/powerpacks/{powerpack_id}"
.replaceAll(
"\\{" + "powerpack_id" + "\\}", apiClient.escapeString(powerpackId.toString()));
Map localVarHeaderParams = new HashMap();
Invocation.Builder builder =
apiClient.createBuilder(
"v2.PowerpackApi.getPowerpack",
localVarPath,
new ArrayList(),
localVarHeaderParams,
new HashMap(),
new String[] {"application/json"},
new String[] {"AuthZ", "apiKeyAuth", "appKeyAuth"});
return apiClient.invokeAPI(
"GET",
builder,
localVarHeaderParams,
new String[] {},
localVarPostBody,
new HashMap(),
false,
new GenericType() {});
}
/**
* Get a Powerpack.
*
* See {@link #getPowerpackWithHttpInfo}.
*
* @param powerpackId ID of the powerpack. (required)
* @return CompletableFuture<ApiResponse<PowerpackResponse>>
*/
public CompletableFuture> getPowerpackWithHttpInfoAsync(
String powerpackId) {
Object localVarPostBody = null;
// verify the required parameter 'powerpackId' is set
if (powerpackId == null) {
CompletableFuture> result = new CompletableFuture<>();
result.completeExceptionally(
new ApiException(
400, "Missing the required parameter 'powerpackId' when calling getPowerpack"));
return result;
}
// create path and map variables
String localVarPath =
"/api/v2/powerpacks/{powerpack_id}"
.replaceAll(
"\\{" + "powerpack_id" + "\\}", apiClient.escapeString(powerpackId.toString()));
Map localVarHeaderParams = new HashMap();
Invocation.Builder builder;
try {
builder =
apiClient.createBuilder(
"v2.PowerpackApi.getPowerpack",
localVarPath,
new ArrayList(),
localVarHeaderParams,
new HashMap(),
new String[] {"application/json"},
new String[] {"AuthZ", "apiKeyAuth", "appKeyAuth"});
} catch (ApiException ex) {
CompletableFuture> result = new CompletableFuture<>();
result.completeExceptionally(ex);
return result;
}
return apiClient.invokeAPIAsync(
"GET",
builder,
localVarHeaderParams,
new String[] {},
localVarPostBody,
new HashMap(),
false,
new GenericType() {});
}
/** Manage optional parameters to listPowerpacks. */
public static class ListPowerpacksOptionalParameters {
private Long pageLimit;
private Long pageOffset;
/**
* Set pageLimit.
*
* @param pageLimit Maximum number of powerpacks in the response. (optional, default to 25)
* @return ListPowerpacksOptionalParameters
*/
public ListPowerpacksOptionalParameters pageLimit(Long pageLimit) {
this.pageLimit = pageLimit;
return this;
}
/**
* Set pageOffset.
*
* @param pageOffset Specific offset to use as the beginning of the returned page. (optional,
* default to 0)
* @return ListPowerpacksOptionalParameters
*/
public ListPowerpacksOptionalParameters pageOffset(Long pageOffset) {
this.pageOffset = pageOffset;
return this;
}
}
/**
* Get all powerpacks.
*
* See {@link #listPowerpacksWithHttpInfo}.
*
* @return ListPowerpacksResponse
* @throws ApiException if fails to make API call
*/
public ListPowerpacksResponse listPowerpacks() throws ApiException {
return listPowerpacksWithHttpInfo(new ListPowerpacksOptionalParameters()).getData();
}
/**
* Get all powerpacks.
*
*
See {@link #listPowerpacksWithHttpInfoAsync}.
*
* @return CompletableFuture<ListPowerpacksResponse>
*/
public CompletableFuture listPowerpacksAsync() {
return listPowerpacksWithHttpInfoAsync(new ListPowerpacksOptionalParameters())
.thenApply(
response -> {
return response.getData();
});
}
/**
* Get all powerpacks.
*
* See {@link #listPowerpacksWithHttpInfo}.
*
* @param parameters Optional parameters for the request.
* @return ListPowerpacksResponse
* @throws ApiException if fails to make API call
*/
public ListPowerpacksResponse listPowerpacks(ListPowerpacksOptionalParameters parameters)
throws ApiException {
return listPowerpacksWithHttpInfo(parameters).getData();
}
/**
* Get all powerpacks.
*
*
See {@link #listPowerpacksWithHttpInfoAsync}.
*
* @param parameters Optional parameters for the request.
* @return CompletableFuture<ListPowerpacksResponse>
*/
public CompletableFuture listPowerpacksAsync(
ListPowerpacksOptionalParameters parameters) {
return listPowerpacksWithHttpInfoAsync(parameters)
.thenApply(
response -> {
return response.getData();
});
}
/**
* Get all powerpacks.
*
* See {@link #listPowerpacksWithHttpInfo}.
*
* @return PaginationIterable<PowerpackData>
*/
public PaginationIterable listPowerpacksWithPagination() {
ListPowerpacksOptionalParameters parameters = new ListPowerpacksOptionalParameters();
return listPowerpacksWithPagination(parameters);
}
/**
* Get all powerpacks.
*
* See {@link #listPowerpacksWithHttpInfo}.
*
* @return ListPowerpacksResponse
*/
public PaginationIterable listPowerpacksWithPagination(
ListPowerpacksOptionalParameters parameters) {
String resultsPath = "getData";
String valueGetterPath = "";
String valueSetterPath = "pageOffset";
Boolean valueSetterParamOptional = true;
Long limit;
if (parameters.pageLimit == null) {
limit = 25l;
parameters.pageLimit(limit);
} else {
limit = parameters.pageLimit;
}
LinkedHashMap args = new LinkedHashMap();
args.put("optionalParams", parameters);
PaginationIterable iterator =
new PaginationIterable(
this,
"listPowerpacks",
resultsPath,
valueGetterPath,
valueSetterPath,
valueSetterParamOptional,
true,
limit,
args);
return iterator;
}
/**
* Get a list of all powerpacks.
*
* @param parameters Optional parameters for the request.
* @return ApiResponse<ListPowerpacksResponse>
* @throws ApiException if fails to make API call
* @http.response.details
*
* Response details
* Status Code Description Response Headers
* 200 OK -
* 429 Too many requests -
*
*/
public ApiResponse listPowerpacksWithHttpInfo(
ListPowerpacksOptionalParameters parameters) throws ApiException {
Object localVarPostBody = null;
Long pageLimit = parameters.pageLimit;
Long pageOffset = parameters.pageOffset;
// create path and map variables
String localVarPath = "/api/v2/powerpacks";
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPairs("", "page[limit]", pageLimit));
localVarQueryParams.addAll(apiClient.parameterToPairs("", "page[offset]", pageOffset));
Invocation.Builder builder =
apiClient.createBuilder(
"v2.PowerpackApi.listPowerpacks",
localVarPath,
localVarQueryParams,
localVarHeaderParams,
new HashMap(),
new String[] {"application/json"},
new String[] {"AuthZ", "apiKeyAuth", "appKeyAuth"});
return apiClient.invokeAPI(
"GET",
builder,
localVarHeaderParams,
new String[] {},
localVarPostBody,
new HashMap(),
false,
new GenericType() {});
}
/**
* Get all powerpacks.
*
* See {@link #listPowerpacksWithHttpInfo}.
*
* @param parameters Optional parameters for the request.
* @return CompletableFuture<ApiResponse<ListPowerpacksResponse>>
*/
public CompletableFuture> listPowerpacksWithHttpInfoAsync(
ListPowerpacksOptionalParameters parameters) {
Object localVarPostBody = null;
Long pageLimit = parameters.pageLimit;
Long pageOffset = parameters.pageOffset;
// create path and map variables
String localVarPath = "/api/v2/powerpacks";
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPairs("", "page[limit]", pageLimit));
localVarQueryParams.addAll(apiClient.parameterToPairs("", "page[offset]", pageOffset));
Invocation.Builder builder;
try {
builder =
apiClient.createBuilder(
"v2.PowerpackApi.listPowerpacks",
localVarPath,
localVarQueryParams,
localVarHeaderParams,
new HashMap(),
new String[] {"application/json"},
new String[] {"AuthZ", "apiKeyAuth", "appKeyAuth"});
} catch (ApiException ex) {
CompletableFuture> result = new CompletableFuture<>();
result.completeExceptionally(ex);
return result;
}
return apiClient.invokeAPIAsync(
"GET",
builder,
localVarHeaderParams,
new String[] {},
localVarPostBody,
new HashMap(),
false,
new GenericType() {});
}
/**
* Update a powerpack.
*
* See {@link #updatePowerpackWithHttpInfo}.
*
* @param powerpackId ID of the powerpack. (required)
* @param body Update a powerpack request body. (required)
* @return PowerpackResponse
* @throws ApiException if fails to make API call
*/
public PowerpackResponse updatePowerpack(String powerpackId, Powerpack body) throws ApiException {
return updatePowerpackWithHttpInfo(powerpackId, body).getData();
}
/**
* Update a powerpack.
*
*
See {@link #updatePowerpackWithHttpInfoAsync}.
*
* @param powerpackId ID of the powerpack. (required)
* @param body Update a powerpack request body. (required)
* @return CompletableFuture<PowerpackResponse>
*/
public CompletableFuture updatePowerpackAsync(
String powerpackId, Powerpack body) {
return updatePowerpackWithHttpInfoAsync(powerpackId, body)
.thenApply(
response -> {
return response.getData();
});
}
/**
* Update a powerpack.
*
* @param powerpackId ID of the powerpack. (required)
* @param body Update a powerpack request body. (required)
* @return ApiResponse<PowerpackResponse>
* @throws ApiException if fails to make API call
* @http.response.details
*
* Response details
* Status Code Description Response Headers
* 200 OK -
* 400 Bad Request -
* 404 Powerpack Not Found -
* 429 Too many requests -
*
*/
public ApiResponse updatePowerpackWithHttpInfo(
String powerpackId, Powerpack body) throws ApiException {
Object localVarPostBody = body;
// verify the required parameter 'powerpackId' is set
if (powerpackId == null) {
throw new ApiException(
400, "Missing the required parameter 'powerpackId' when calling updatePowerpack");
}
// verify the required parameter 'body' is set
if (body == null) {
throw new ApiException(
400, "Missing the required parameter 'body' when calling updatePowerpack");
}
// create path and map variables
String localVarPath =
"/api/v2/powerpacks/{powerpack_id}"
.replaceAll(
"\\{" + "powerpack_id" + "\\}", apiClient.escapeString(powerpackId.toString()));
Map localVarHeaderParams = new HashMap();
Invocation.Builder builder =
apiClient.createBuilder(
"v2.PowerpackApi.updatePowerpack",
localVarPath,
new ArrayList(),
localVarHeaderParams,
new HashMap(),
new String[] {"application/json"},
new String[] {"AuthZ", "apiKeyAuth", "appKeyAuth"});
return apiClient.invokeAPI(
"PATCH",
builder,
localVarHeaderParams,
new String[] {"application/json"},
localVarPostBody,
new HashMap(),
false,
new GenericType() {});
}
/**
* Update a powerpack.
*
* See {@link #updatePowerpackWithHttpInfo}.
*
* @param powerpackId ID of the powerpack. (required)
* @param body Update a powerpack request body. (required)
* @return CompletableFuture<ApiResponse<PowerpackResponse>>
*/
public CompletableFuture> updatePowerpackWithHttpInfoAsync(
String powerpackId, Powerpack body) {
Object localVarPostBody = body;
// verify the required parameter 'powerpackId' is set
if (powerpackId == null) {
CompletableFuture> result = new CompletableFuture<>();
result.completeExceptionally(
new ApiException(
400, "Missing the required parameter 'powerpackId' when calling updatePowerpack"));
return result;
}
// verify the required parameter 'body' is set
if (body == null) {
CompletableFuture> result = new CompletableFuture<>();
result.completeExceptionally(
new ApiException(
400, "Missing the required parameter 'body' when calling updatePowerpack"));
return result;
}
// create path and map variables
String localVarPath =
"/api/v2/powerpacks/{powerpack_id}"
.replaceAll(
"\\{" + "powerpack_id" + "\\}", apiClient.escapeString(powerpackId.toString()));
Map localVarHeaderParams = new HashMap();
Invocation.Builder builder;
try {
builder =
apiClient.createBuilder(
"v2.PowerpackApi.updatePowerpack",
localVarPath,
new ArrayList(),
localVarHeaderParams,
new HashMap(),
new String[] {"application/json"},
new String[] {"AuthZ", "apiKeyAuth", "appKeyAuth"});
} catch (ApiException ex) {
CompletableFuture> result = new CompletableFuture<>();
result.completeExceptionally(ex);
return result;
}
return apiClient.invokeAPIAsync(
"PATCH",
builder,
localVarHeaderParams,
new String[] {"application/json"},
localVarPostBody,
new HashMap(),
false,
new GenericType() {});
}
}