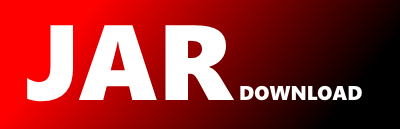
com.datastax.driver.mapping.MappedUDTCodec Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cassandra-driver-mapping Show documentation
Show all versions of cassandra-driver-mapping Show documentation
Object mapper for the DataStax CQL Java Driver.
/*
* Copyright (C) 2012-2015 DataStax Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.datastax.driver.mapping;
import java.nio.ByteBuffer;
import java.util.List;
import com.google.common.collect.Lists;
import com.datastax.driver.core.*;
import com.datastax.driver.core.exceptions.InvalidTypeException;
/**
* Serializes a class annotated with {@code @UDT} to the corresponding CQL user-defined type.
*/
class MappedUDTCodec extends TypeCodec {
private final UserType cqlUserType;
private final Class udtClass;
private final List> columnMappers;
private final CodecRegistry codecRegistry;
public MappedUDTCodec(UserType cqlUserType, Class udtClass, List> columnMappers, MappingManager mappingManager) {
super(cqlUserType, udtClass);
this.cqlUserType = cqlUserType;
this.udtClass = udtClass;
this.columnMappers = columnMappers;
this.codecRegistry = mappingManager.getSession().getCluster().getConfiguration().getCodecRegistry();
}
@Override
public ByteBuffer serialize(T sourceObject, ProtocolVersion protocolVersion) throws InvalidTypeException {
if (sourceObject == null)
return null;
int size = 0;
List serializedFields = Lists.newArrayList();
for (ColumnMapper cm : columnMappers) {
Object value = cm.getValue(sourceObject);
TypeCodec
© 2015 - 2025 Weber Informatics LLC | Privacy Policy