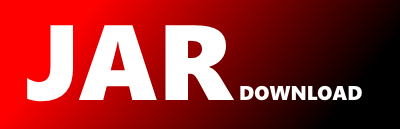
com.datastax.driver.mapping.Result Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cassandra-driver-mapping Show documentation
Show all versions of cassandra-driver-mapping Show documentation
Object mapper for the DataStax CQL Java Driver.
/*
* Copyright (C) 2012-2015 DataStax Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.datastax.driver.mapping;
import com.datastax.driver.core.*;
import com.google.common.base.Function;
import com.google.common.util.concurrent.Futures;
import com.google.common.util.concurrent.ListenableFuture;
import java.util.*;
/**
* A result set whose rows are mapped to an entity class.
*/
public class Result implements PagingIterable, T> {
private final ResultSet rs;
private final EntityMapper mapper;
private final boolean useAlias;
Result(ResultSet rs, EntityMapper mapper, boolean useAlias) {
this.rs = rs;
this.mapper = mapper;
this.useAlias = useAlias;
}
private T map(Row row) {
T entity = mapper.newEntity();
for (AliasedMappedProperty> c : mapper.allColumns) {
@SuppressWarnings("unchecked")
AliasedMappedProperty
© 2015 - 2024 Weber Informatics LLC | Privacy Policy