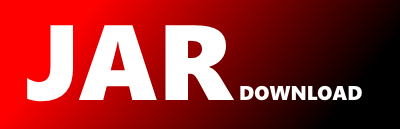
com.datastax.oss.dsbulk.generated.cql3.CqlBaseVisitor Maven / Gradle / Ivy
Show all versions of dsbulk-cql Show documentation
// Generated from com/datastax/oss/dsbulk/generated/cql3/Cql.g4 by ANTLR 4.9.3
package com.datastax.oss.dsbulk.generated.cql3;
import org.antlr.v4.runtime.tree.AbstractParseTreeVisitor;
/**
* This class provides an empty implementation of {@link CqlVisitor},
* which can be extended to create a visitor which only needs to handle a subset
* of the available methods.
*
* @param The return type of the visit operation. Use {@link Void} for
* operations with no return type.
*/
public class CqlBaseVisitor extends AbstractParseTreeVisitor implements CqlVisitor {
/**
* {@inheritDoc}
*
* The default implementation returns the result of calling
* {@link #visitChildren} on {@code ctx}.
*/
@Override public T visitCqlStatement(CqlParser.CqlStatementContext ctx) { return visitChildren(ctx); }
/**
* {@inheritDoc}
*
* The default implementation returns the result of calling
* {@link #visitChildren} on {@code ctx}.
*/
@Override public T visitSelectStatement(CqlParser.SelectStatementContext ctx) { return visitChildren(ctx); }
/**
* {@inheritDoc}
*
* The default implementation returns the result of calling
* {@link #visitChildren} on {@code ctx}.
*/
@Override public T visitSelectClause(CqlParser.SelectClauseContext ctx) { return visitChildren(ctx); }
/**
* {@inheritDoc}
*
* The default implementation returns the result of calling
* {@link #visitChildren} on {@code ctx}.
*/
@Override public T visitSelector(CqlParser.SelectorContext ctx) { return visitChildren(ctx); }
/**
* {@inheritDoc}
*
* The default implementation returns the result of calling
* {@link #visitChildren} on {@code ctx}.
*/
@Override public T visitUnaliasedSelector(CqlParser.UnaliasedSelectorContext ctx) { return visitChildren(ctx); }
/**
* {@inheritDoc}
*
* The default implementation returns the result of calling
* {@link #visitChildren} on {@code ctx}.
*/
@Override public T visitSelectionFunctionArgs(CqlParser.SelectionFunctionArgsContext ctx) { return visitChildren(ctx); }
/**
* {@inheritDoc}
*
* The default implementation returns the result of calling
* {@link #visitChildren} on {@code ctx}.
*/
@Override public T visitWhereClause(CqlParser.WhereClauseContext ctx) { return visitChildren(ctx); }
/**
* {@inheritDoc}
*
* The default implementation returns the result of calling
* {@link #visitChildren} on {@code ctx}.
*/
@Override public T visitRelationOrExpression(CqlParser.RelationOrExpressionContext ctx) { return visitChildren(ctx); }
/**
* {@inheritDoc}
*
* The default implementation returns the result of calling
* {@link #visitChildren} on {@code ctx}.
*/
@Override public T visitCustomIndexExpression(CqlParser.CustomIndexExpressionContext ctx) { return visitChildren(ctx); }
/**
* {@inheritDoc}
*
* The default implementation returns the result of calling
* {@link #visitChildren} on {@code ctx}.
*/
@Override public T visitOrderByClause(CqlParser.OrderByClauseContext ctx) { return visitChildren(ctx); }
/**
* {@inheritDoc}
*
* The default implementation returns the result of calling
* {@link #visitChildren} on {@code ctx}.
*/
@Override public T visitGroupByClause(CqlParser.GroupByClauseContext ctx) { return visitChildren(ctx); }
/**
* {@inheritDoc}
*
* The default implementation returns the result of calling
* {@link #visitChildren} on {@code ctx}.
*/
@Override public T visitPerPartitionLimitClause(CqlParser.PerPartitionLimitClauseContext ctx) { return visitChildren(ctx); }
/**
* {@inheritDoc}
*
* The default implementation returns the result of calling
* {@link #visitChildren} on {@code ctx}.
*/
@Override public T visitLimitClause(CqlParser.LimitClauseContext ctx) { return visitChildren(ctx); }
/**
* {@inheritDoc}
*
* The default implementation returns the result of calling
* {@link #visitChildren} on {@code ctx}.
*/
@Override public T visitInsertStatement(CqlParser.InsertStatementContext ctx) { return visitChildren(ctx); }
/**
* {@inheritDoc}
*
* The default implementation returns the result of calling
* {@link #visitChildren} on {@code ctx}.
*/
@Override public T visitNormalInsertStatement(CqlParser.NormalInsertStatementContext ctx) { return visitChildren(ctx); }
/**
* {@inheritDoc}
*
* The default implementation returns the result of calling
* {@link #visitChildren} on {@code ctx}.
*/
@Override public T visitJsonInsertStatement(CqlParser.JsonInsertStatementContext ctx) { return visitChildren(ctx); }
/**
* {@inheritDoc}
*
* The default implementation returns the result of calling
* {@link #visitChildren} on {@code ctx}.
*/
@Override public T visitJsonValue(CqlParser.JsonValueContext ctx) { return visitChildren(ctx); }
/**
* {@inheritDoc}
*
* The default implementation returns the result of calling
* {@link #visitChildren} on {@code ctx}.
*/
@Override public T visitUsingClause(CqlParser.UsingClauseContext ctx) { return visitChildren(ctx); }
/**
* {@inheritDoc}
*
* The default implementation returns the result of calling
* {@link #visitChildren} on {@code ctx}.
*/
@Override public T visitUsingClauseObjective(CqlParser.UsingClauseObjectiveContext ctx) { return visitChildren(ctx); }
/**
* {@inheritDoc}
*
* The default implementation returns the result of calling
* {@link #visitChildren} on {@code ctx}.
*/
@Override public T visitUpdateStatement(CqlParser.UpdateStatementContext ctx) { return visitChildren(ctx); }
/**
* {@inheritDoc}
*
* The default implementation returns the result of calling
* {@link #visitChildren} on {@code ctx}.
*/
@Override public T visitUpdateConditions(CqlParser.UpdateConditionsContext ctx) { return visitChildren(ctx); }
/**
* {@inheritDoc}
*
* The default implementation returns the result of calling
* {@link #visitChildren} on {@code ctx}.
*/
@Override public T visitDeleteStatement(CqlParser.DeleteStatementContext ctx) { return visitChildren(ctx); }
/**
* {@inheritDoc}
*
* The default implementation returns the result of calling
* {@link #visitChildren} on {@code ctx}.
*/
@Override public T visitDeleteSelection(CqlParser.DeleteSelectionContext ctx) { return visitChildren(ctx); }
/**
* {@inheritDoc}
*
* The default implementation returns the result of calling
* {@link #visitChildren} on {@code ctx}.
*/
@Override public T visitDeleteOp(CqlParser.DeleteOpContext ctx) { return visitChildren(ctx); }
/**
* {@inheritDoc}
*
* The default implementation returns the result of calling
* {@link #visitChildren} on {@code ctx}.
*/
@Override public T visitUsingClauseDelete(CqlParser.UsingClauseDeleteContext ctx) { return visitChildren(ctx); }
/**
* {@inheritDoc}
*
* The default implementation returns the result of calling
* {@link #visitChildren} on {@code ctx}.
*/
@Override public T visitBatchStatement(CqlParser.BatchStatementContext ctx) { return visitChildren(ctx); }
/**
* {@inheritDoc}
*
* The default implementation returns the result of calling
* {@link #visitChildren} on {@code ctx}.
*/
@Override public T visitBatchStatementObjective(CqlParser.BatchStatementObjectiveContext ctx) { return visitChildren(ctx); }
/**
* {@inheritDoc}
*
* The default implementation returns the result of calling
* {@link #visitChildren} on {@code ctx}.
*/
@Override public T visitCident(CqlParser.CidentContext ctx) { return visitChildren(ctx); }
/**
* {@inheritDoc}
*
* The default implementation returns the result of calling
* {@link #visitChildren} on {@code ctx}.
*/
@Override public T visitFident(CqlParser.FidentContext ctx) { return visitChildren(ctx); }
/**
* {@inheritDoc}
*
* The default implementation returns the result of calling
* {@link #visitChildren} on {@code ctx}.
*/
@Override public T visitNoncolIdent(CqlParser.NoncolIdentContext ctx) { return visitChildren(ctx); }
/**
* {@inheritDoc}
*
* The default implementation returns the result of calling
* {@link #visitChildren} on {@code ctx}.
*/
@Override public T visitKeyspaceName(CqlParser.KeyspaceNameContext ctx) { return visitChildren(ctx); }
/**
* {@inheritDoc}
*
* The default implementation returns the result of calling
* {@link #visitChildren} on {@code ctx}.
*/
@Override public T visitColumnFamilyName(CqlParser.ColumnFamilyNameContext ctx) { return visitChildren(ctx); }
/**
* {@inheritDoc}
*
* The default implementation returns the result of calling
* {@link #visitChildren} on {@code ctx}.
*/
@Override public T visitUserTypeName(CqlParser.UserTypeNameContext ctx) { return visitChildren(ctx); }
/**
* {@inheritDoc}
*
* The default implementation returns the result of calling
* {@link #visitChildren} on {@code ctx}.
*/
@Override public T visitKsName(CqlParser.KsNameContext ctx) { return visitChildren(ctx); }
/**
* {@inheritDoc}
*
* The default implementation returns the result of calling
* {@link #visitChildren} on {@code ctx}.
*/
@Override public T visitCfName(CqlParser.CfNameContext ctx) { return visitChildren(ctx); }
/**
* {@inheritDoc}
*
* The default implementation returns the result of calling
* {@link #visitChildren} on {@code ctx}.
*/
@Override public T visitIdxName(CqlParser.IdxNameContext ctx) { return visitChildren(ctx); }
/**
* {@inheritDoc}
*
* The default implementation returns the result of calling
* {@link #visitChildren} on {@code ctx}.
*/
@Override public T visitConstant(CqlParser.ConstantContext ctx) { return visitChildren(ctx); }
/**
* {@inheritDoc}
*
* The default implementation returns the result of calling
* {@link #visitChildren} on {@code ctx}.
*/
@Override public T visitSetOrMapLiteral(CqlParser.SetOrMapLiteralContext ctx) { return visitChildren(ctx); }
/**
* {@inheritDoc}
*
* The default implementation returns the result of calling
* {@link #visitChildren} on {@code ctx}.
*/
@Override public T visitCollectionLiteral(CqlParser.CollectionLiteralContext ctx) { return visitChildren(ctx); }
/**
* {@inheritDoc}
*
* The default implementation returns the result of calling
* {@link #visitChildren} on {@code ctx}.
*/
@Override public T visitUsertypeLiteral(CqlParser.UsertypeLiteralContext ctx) { return visitChildren(ctx); }
/**
* {@inheritDoc}
*
* The default implementation returns the result of calling
* {@link #visitChildren} on {@code ctx}.
*/
@Override public T visitTupleLiteral(CqlParser.TupleLiteralContext ctx) { return visitChildren(ctx); }
/**
* {@inheritDoc}
*
* The default implementation returns the result of calling
* {@link #visitChildren} on {@code ctx}.
*/
@Override public T visitValue(CqlParser.ValueContext ctx) { return visitChildren(ctx); }
/**
* {@inheritDoc}
*
* The default implementation returns the result of calling
* {@link #visitChildren} on {@code ctx}.
*/
@Override public T visitIntValue(CqlParser.IntValueContext ctx) { return visitChildren(ctx); }
/**
* {@inheritDoc}
*
* The default implementation returns the result of calling
* {@link #visitChildren} on {@code ctx}.
*/
@Override public T visitFunctionName(CqlParser.FunctionNameContext ctx) { return visitChildren(ctx); }
/**
* {@inheritDoc}
*
* The default implementation returns the result of calling
* {@link #visitChildren} on {@code ctx}.
*/
@Override public T visitAllowedFunctionName(CqlParser.AllowedFunctionNameContext ctx) { return visitChildren(ctx); }
/**
* {@inheritDoc}
*
* The default implementation returns the result of calling
* {@link #visitChildren} on {@code ctx}.
*/
@Override public T visitFunction(CqlParser.FunctionContext ctx) { return visitChildren(ctx); }
/**
* {@inheritDoc}
*
* The default implementation returns the result of calling
* {@link #visitChildren} on {@code ctx}.
*/
@Override public T visitFunctionArgs(CqlParser.FunctionArgsContext ctx) { return visitChildren(ctx); }
/**
* {@inheritDoc}
*
* The default implementation returns the result of calling
* {@link #visitChildren} on {@code ctx}.
*/
@Override public T visitTerm(CqlParser.TermContext ctx) { return visitChildren(ctx); }
/**
* {@inheritDoc}
*
* The default implementation returns the result of calling
* {@link #visitChildren} on {@code ctx}.
*/
@Override public T visitColumnOperation(CqlParser.ColumnOperationContext ctx) { return visitChildren(ctx); }
/**
* {@inheritDoc}
*
* The default implementation returns the result of calling
* {@link #visitChildren} on {@code ctx}.
*/
@Override public T visitColumnOperationDifferentiator(CqlParser.ColumnOperationDifferentiatorContext ctx) { return visitChildren(ctx); }
/**
* {@inheritDoc}
*
* The default implementation returns the result of calling
* {@link #visitChildren} on {@code ctx}.
*/
@Override public T visitNormalColumnOperation(CqlParser.NormalColumnOperationContext ctx) { return visitChildren(ctx); }
/**
* {@inheritDoc}
*
* The default implementation returns the result of calling
* {@link #visitChildren} on {@code ctx}.
*/
@Override public T visitShorthandColumnOperation(CqlParser.ShorthandColumnOperationContext ctx) { return visitChildren(ctx); }
/**
* {@inheritDoc}
*
* The default implementation returns the result of calling
* {@link #visitChildren} on {@code ctx}.
*/
@Override public T visitCollectionColumnOperation(CqlParser.CollectionColumnOperationContext ctx) { return visitChildren(ctx); }
/**
* {@inheritDoc}
*
* The default implementation returns the result of calling
* {@link #visitChildren} on {@code ctx}.
*/
@Override public T visitUdtColumnOperation(CqlParser.UdtColumnOperationContext ctx) { return visitChildren(ctx); }
/**
* {@inheritDoc}
*
* The default implementation returns the result of calling
* {@link #visitChildren} on {@code ctx}.
*/
@Override public T visitColumnCondition(CqlParser.ColumnConditionContext ctx) { return visitChildren(ctx); }
/**
* {@inheritDoc}
*
* The default implementation returns the result of calling
* {@link #visitChildren} on {@code ctx}.
*/
@Override public T visitRelationType(CqlParser.RelationTypeContext ctx) { return visitChildren(ctx); }
/**
* {@inheritDoc}
*
* The default implementation returns the result of calling
* {@link #visitChildren} on {@code ctx}.
*/
@Override public T visitRelation(CqlParser.RelationContext ctx) { return visitChildren(ctx); }
/**
* {@inheritDoc}
*
* The default implementation returns the result of calling
* {@link #visitChildren} on {@code ctx}.
*/
@Override public T visitInMarker(CqlParser.InMarkerContext ctx) { return visitChildren(ctx); }
/**
* {@inheritDoc}
*
* The default implementation returns the result of calling
* {@link #visitChildren} on {@code ctx}.
*/
@Override public T visitTupleOfIdentifiers(CqlParser.TupleOfIdentifiersContext ctx) { return visitChildren(ctx); }
/**
* {@inheritDoc}
*
* The default implementation returns the result of calling
* {@link #visitChildren} on {@code ctx}.
*/
@Override public T visitSingleColumnInValues(CqlParser.SingleColumnInValuesContext ctx) { return visitChildren(ctx); }
/**
* {@inheritDoc}
*
* The default implementation returns the result of calling
* {@link #visitChildren} on {@code ctx}.
*/
@Override public T visitTupleOfTupleLiterals(CqlParser.TupleOfTupleLiteralsContext ctx) { return visitChildren(ctx); }
/**
* {@inheritDoc}
*
* The default implementation returns the result of calling
* {@link #visitChildren} on {@code ctx}.
*/
@Override public T visitMarkerForTuple(CqlParser.MarkerForTupleContext ctx) { return visitChildren(ctx); }
/**
* {@inheritDoc}
*
* The default implementation returns the result of calling
* {@link #visitChildren} on {@code ctx}.
*/
@Override public T visitTupleOfMarkersForTuples(CqlParser.TupleOfMarkersForTuplesContext ctx) { return visitChildren(ctx); }
/**
* {@inheritDoc}
*
* The default implementation returns the result of calling
* {@link #visitChildren} on {@code ctx}.
*/
@Override public T visitInMarkerForTuple(CqlParser.InMarkerForTupleContext ctx) { return visitChildren(ctx); }
/**
* {@inheritDoc}
*
* The default implementation returns the result of calling
* {@link #visitChildren} on {@code ctx}.
*/
@Override public T visitComparatorType(CqlParser.ComparatorTypeContext ctx) { return visitChildren(ctx); }
/**
* {@inheritDoc}
*
* The default implementation returns the result of calling
* {@link #visitChildren} on {@code ctx}.
*/
@Override public T visitNativeType(CqlParser.NativeTypeContext ctx) { return visitChildren(ctx); }
/**
* {@inheritDoc}
*
* The default implementation returns the result of calling
* {@link #visitChildren} on {@code ctx}.
*/
@Override public T visitCollectionType(CqlParser.CollectionTypeContext ctx) { return visitChildren(ctx); }
/**
* {@inheritDoc}
*
* The default implementation returns the result of calling
* {@link #visitChildren} on {@code ctx}.
*/
@Override public T visitTupleType(CqlParser.TupleTypeContext ctx) { return visitChildren(ctx); }
/**
* {@inheritDoc}
*
* The default implementation returns the result of calling
* {@link #visitChildren} on {@code ctx}.
*/
@Override public T visitNonTypeIdent(CqlParser.NonTypeIdentContext ctx) { return visitChildren(ctx); }
/**
* {@inheritDoc}
*
* The default implementation returns the result of calling
* {@link #visitChildren} on {@code ctx}.
*/
@Override public T visitUnreservedKeyword(CqlParser.UnreservedKeywordContext ctx) { return visitChildren(ctx); }
/**
* {@inheritDoc}
*
* The default implementation returns the result of calling
* {@link #visitChildren} on {@code ctx}.
*/
@Override public T visitUnreservedFunctionKeyword(CqlParser.UnreservedFunctionKeywordContext ctx) { return visitChildren(ctx); }
/**
* {@inheritDoc}
*
* The default implementation returns the result of calling
* {@link #visitChildren} on {@code ctx}.
*/
@Override public T visitBasicUnreservedKeyword(CqlParser.BasicUnreservedKeywordContext ctx) { return visitChildren(ctx); }
}