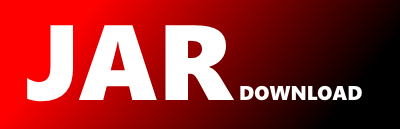
com.datastax.oss.dsbulk.generated.cql3.CqlVisitor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of dsbulk-cql Show documentation
Show all versions of dsbulk-cql Show documentation
CQL parser for the DataStax Bulk Loader.
The newest version!
// Generated from com/datastax/oss/dsbulk/generated/cql3/Cql.g4 by ANTLR 4.9.3
package com.datastax.oss.dsbulk.generated.cql3;
import org.antlr.v4.runtime.tree.ParseTreeVisitor;
/**
* This interface defines a complete generic visitor for a parse tree produced
* by {@link CqlParser}.
*
* @param The return type of the visit operation. Use {@link Void} for
* operations with no return type.
*/
public interface CqlVisitor extends ParseTreeVisitor {
/**
* Visit a parse tree produced by {@link CqlParser#cqlStatement}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitCqlStatement(CqlParser.CqlStatementContext ctx);
/**
* Visit a parse tree produced by {@link CqlParser#selectStatement}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitSelectStatement(CqlParser.SelectStatementContext ctx);
/**
* Visit a parse tree produced by {@link CqlParser#selectClause}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitSelectClause(CqlParser.SelectClauseContext ctx);
/**
* Visit a parse tree produced by {@link CqlParser#selector}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitSelector(CqlParser.SelectorContext ctx);
/**
* Visit a parse tree produced by {@link CqlParser#unaliasedSelector}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitUnaliasedSelector(CqlParser.UnaliasedSelectorContext ctx);
/**
* Visit a parse tree produced by {@link CqlParser#selectionFunctionArgs}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitSelectionFunctionArgs(CqlParser.SelectionFunctionArgsContext ctx);
/**
* Visit a parse tree produced by {@link CqlParser#whereClause}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitWhereClause(CqlParser.WhereClauseContext ctx);
/**
* Visit a parse tree produced by {@link CqlParser#relationOrExpression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitRelationOrExpression(CqlParser.RelationOrExpressionContext ctx);
/**
* Visit a parse tree produced by {@link CqlParser#customIndexExpression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitCustomIndexExpression(CqlParser.CustomIndexExpressionContext ctx);
/**
* Visit a parse tree produced by {@link CqlParser#orderByClause}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitOrderByClause(CqlParser.OrderByClauseContext ctx);
/**
* Visit a parse tree produced by {@link CqlParser#groupByClause}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitGroupByClause(CqlParser.GroupByClauseContext ctx);
/**
* Visit a parse tree produced by {@link CqlParser#perPartitionLimitClause}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitPerPartitionLimitClause(CqlParser.PerPartitionLimitClauseContext ctx);
/**
* Visit a parse tree produced by {@link CqlParser#limitClause}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitLimitClause(CqlParser.LimitClauseContext ctx);
/**
* Visit a parse tree produced by {@link CqlParser#insertStatement}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitInsertStatement(CqlParser.InsertStatementContext ctx);
/**
* Visit a parse tree produced by {@link CqlParser#normalInsertStatement}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitNormalInsertStatement(CqlParser.NormalInsertStatementContext ctx);
/**
* Visit a parse tree produced by {@link CqlParser#jsonInsertStatement}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitJsonInsertStatement(CqlParser.JsonInsertStatementContext ctx);
/**
* Visit a parse tree produced by {@link CqlParser#jsonValue}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitJsonValue(CqlParser.JsonValueContext ctx);
/**
* Visit a parse tree produced by {@link CqlParser#usingClause}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitUsingClause(CqlParser.UsingClauseContext ctx);
/**
* Visit a parse tree produced by {@link CqlParser#usingClauseObjective}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitUsingClauseObjective(CqlParser.UsingClauseObjectiveContext ctx);
/**
* Visit a parse tree produced by {@link CqlParser#updateStatement}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitUpdateStatement(CqlParser.UpdateStatementContext ctx);
/**
* Visit a parse tree produced by {@link CqlParser#updateConditions}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitUpdateConditions(CqlParser.UpdateConditionsContext ctx);
/**
* Visit a parse tree produced by {@link CqlParser#deleteStatement}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitDeleteStatement(CqlParser.DeleteStatementContext ctx);
/**
* Visit a parse tree produced by {@link CqlParser#deleteSelection}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitDeleteSelection(CqlParser.DeleteSelectionContext ctx);
/**
* Visit a parse tree produced by {@link CqlParser#deleteOp}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitDeleteOp(CqlParser.DeleteOpContext ctx);
/**
* Visit a parse tree produced by {@link CqlParser#usingClauseDelete}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitUsingClauseDelete(CqlParser.UsingClauseDeleteContext ctx);
/**
* Visit a parse tree produced by {@link CqlParser#batchStatement}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitBatchStatement(CqlParser.BatchStatementContext ctx);
/**
* Visit a parse tree produced by {@link CqlParser#batchStatementObjective}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitBatchStatementObjective(CqlParser.BatchStatementObjectiveContext ctx);
/**
* Visit a parse tree produced by {@link CqlParser#cident}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitCident(CqlParser.CidentContext ctx);
/**
* Visit a parse tree produced by {@link CqlParser#fident}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitFident(CqlParser.FidentContext ctx);
/**
* Visit a parse tree produced by {@link CqlParser#noncolIdent}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitNoncolIdent(CqlParser.NoncolIdentContext ctx);
/**
* Visit a parse tree produced by {@link CqlParser#keyspaceName}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitKeyspaceName(CqlParser.KeyspaceNameContext ctx);
/**
* Visit a parse tree produced by {@link CqlParser#columnFamilyName}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitColumnFamilyName(CqlParser.ColumnFamilyNameContext ctx);
/**
* Visit a parse tree produced by {@link CqlParser#userTypeName}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitUserTypeName(CqlParser.UserTypeNameContext ctx);
/**
* Visit a parse tree produced by {@link CqlParser#ksName}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitKsName(CqlParser.KsNameContext ctx);
/**
* Visit a parse tree produced by {@link CqlParser#cfName}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitCfName(CqlParser.CfNameContext ctx);
/**
* Visit a parse tree produced by {@link CqlParser#idxName}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitIdxName(CqlParser.IdxNameContext ctx);
/**
* Visit a parse tree produced by {@link CqlParser#constant}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitConstant(CqlParser.ConstantContext ctx);
/**
* Visit a parse tree produced by {@link CqlParser#setOrMapLiteral}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitSetOrMapLiteral(CqlParser.SetOrMapLiteralContext ctx);
/**
* Visit a parse tree produced by {@link CqlParser#collectionLiteral}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitCollectionLiteral(CqlParser.CollectionLiteralContext ctx);
/**
* Visit a parse tree produced by {@link CqlParser#usertypeLiteral}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitUsertypeLiteral(CqlParser.UsertypeLiteralContext ctx);
/**
* Visit a parse tree produced by {@link CqlParser#tupleLiteral}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitTupleLiteral(CqlParser.TupleLiteralContext ctx);
/**
* Visit a parse tree produced by {@link CqlParser#value}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitValue(CqlParser.ValueContext ctx);
/**
* Visit a parse tree produced by {@link CqlParser#intValue}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitIntValue(CqlParser.IntValueContext ctx);
/**
* Visit a parse tree produced by {@link CqlParser#functionName}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitFunctionName(CqlParser.FunctionNameContext ctx);
/**
* Visit a parse tree produced by {@link CqlParser#allowedFunctionName}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitAllowedFunctionName(CqlParser.AllowedFunctionNameContext ctx);
/**
* Visit a parse tree produced by {@link CqlParser#function}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitFunction(CqlParser.FunctionContext ctx);
/**
* Visit a parse tree produced by {@link CqlParser#functionArgs}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitFunctionArgs(CqlParser.FunctionArgsContext ctx);
/**
* Visit a parse tree produced by {@link CqlParser#term}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitTerm(CqlParser.TermContext ctx);
/**
* Visit a parse tree produced by {@link CqlParser#columnOperation}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitColumnOperation(CqlParser.ColumnOperationContext ctx);
/**
* Visit a parse tree produced by {@link CqlParser#columnOperationDifferentiator}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitColumnOperationDifferentiator(CqlParser.ColumnOperationDifferentiatorContext ctx);
/**
* Visit a parse tree produced by {@link CqlParser#normalColumnOperation}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitNormalColumnOperation(CqlParser.NormalColumnOperationContext ctx);
/**
* Visit a parse tree produced by {@link CqlParser#shorthandColumnOperation}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitShorthandColumnOperation(CqlParser.ShorthandColumnOperationContext ctx);
/**
* Visit a parse tree produced by {@link CqlParser#collectionColumnOperation}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitCollectionColumnOperation(CqlParser.CollectionColumnOperationContext ctx);
/**
* Visit a parse tree produced by {@link CqlParser#udtColumnOperation}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitUdtColumnOperation(CqlParser.UdtColumnOperationContext ctx);
/**
* Visit a parse tree produced by {@link CqlParser#columnCondition}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitColumnCondition(CqlParser.ColumnConditionContext ctx);
/**
* Visit a parse tree produced by {@link CqlParser#relationType}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitRelationType(CqlParser.RelationTypeContext ctx);
/**
* Visit a parse tree produced by {@link CqlParser#relation}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitRelation(CqlParser.RelationContext ctx);
/**
* Visit a parse tree produced by {@link CqlParser#inMarker}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitInMarker(CqlParser.InMarkerContext ctx);
/**
* Visit a parse tree produced by {@link CqlParser#tupleOfIdentifiers}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitTupleOfIdentifiers(CqlParser.TupleOfIdentifiersContext ctx);
/**
* Visit a parse tree produced by {@link CqlParser#singleColumnInValues}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitSingleColumnInValues(CqlParser.SingleColumnInValuesContext ctx);
/**
* Visit a parse tree produced by {@link CqlParser#tupleOfTupleLiterals}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitTupleOfTupleLiterals(CqlParser.TupleOfTupleLiteralsContext ctx);
/**
* Visit a parse tree produced by {@link CqlParser#markerForTuple}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitMarkerForTuple(CqlParser.MarkerForTupleContext ctx);
/**
* Visit a parse tree produced by {@link CqlParser#tupleOfMarkersForTuples}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitTupleOfMarkersForTuples(CqlParser.TupleOfMarkersForTuplesContext ctx);
/**
* Visit a parse tree produced by {@link CqlParser#inMarkerForTuple}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitInMarkerForTuple(CqlParser.InMarkerForTupleContext ctx);
/**
* Visit a parse tree produced by {@link CqlParser#comparatorType}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitComparatorType(CqlParser.ComparatorTypeContext ctx);
/**
* Visit a parse tree produced by {@link CqlParser#nativeType}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitNativeType(CqlParser.NativeTypeContext ctx);
/**
* Visit a parse tree produced by {@link CqlParser#collectionType}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitCollectionType(CqlParser.CollectionTypeContext ctx);
/**
* Visit a parse tree produced by {@link CqlParser#tupleType}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitTupleType(CqlParser.TupleTypeContext ctx);
/**
* Visit a parse tree produced by {@link CqlParser#nonTypeIdent}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitNonTypeIdent(CqlParser.NonTypeIdentContext ctx);
/**
* Visit a parse tree produced by {@link CqlParser#unreservedKeyword}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitUnreservedKeyword(CqlParser.UnreservedKeywordContext ctx);
/**
* Visit a parse tree produced by {@link CqlParser#unreservedFunctionKeyword}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitUnreservedFunctionKeyword(CqlParser.UnreservedFunctionKeywordContext ctx);
/**
* Visit a parse tree produced by {@link CqlParser#basicUnreservedKeyword}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitBasicUnreservedKeyword(CqlParser.BasicUnreservedKeywordContext ctx);
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy