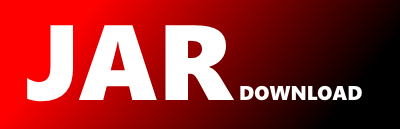
com.datastax.stargate.sdk.doc.CollectionClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of stargate-sdk Show documentation
Show all versions of stargate-sdk Show documentation
Connect to Stargate Data Gateway
/*
* Copyright DataStax, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.datastax.stargate.sdk.doc;
import static com.datastax.stargate.sdk.utils.JsonUtils.marshall;
import static com.datastax.stargate.sdk.utils.JsonUtils.unmarshallBean;
import static com.datastax.stargate.sdk.utils.JsonUtils.unmarshallType;
import java.io.UnsupportedEncodingException;
import java.net.HttpURLConnection;
import java.net.URLEncoder;
import java.nio.charset.StandardCharsets;
import java.util.ArrayList;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import java.util.concurrent.atomic.AtomicInteger;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import org.everit.json.schema.Schema;
import org.everit.json.schema.loader.SchemaLoader;
import org.json.JSONObject;
import com.datastax.stargate.sdk.StargateClientNode;
import com.datastax.stargate.sdk.StargateHttpClient;
import com.datastax.stargate.sdk.core.ApiResponse;
import com.datastax.stargate.sdk.core.ApiResponseHttp;
import com.datastax.stargate.sdk.doc.domain.CollectionDefinition;
import com.datastax.stargate.sdk.doc.domain.DocumentResultPage;
import com.datastax.stargate.sdk.doc.domain.SearchDocumentQuery;
import com.datastax.stargate.sdk.doc.domain.SearchDocumentQuery.SearchDocumentQueryBuilder;
import com.datastax.stargate.sdk.utils.Assert;
import com.datastax.stargate.sdk.utils.JsonUtils;
import com.datastax.stargate.sdk.utils.Utils;
import com.fasterxml.jackson.core.type.TypeReference;
/**
* Work on a dedicated collection without using the Pojo className.
*
* @author Cedrick LUNVEN (@clunven)
*/
public class CollectionClient {
/** Read document id. */
public static final String DOCUMENT_ID = "documentId";
/** Read document id. */
public static final String BATCH_ID_PATH = "id-path";
/** Get Topology of the nodes. */
private final StargateHttpClient stargateHttpClient;
/** Namespace. */
protected NamespaceClient namespaceClient;
/** Collection name. */
protected String collectionName;
/* Mapping type. */
private static TypeReference>>> RESPONSE_SEARCH =
new TypeReference>>>(){};
/**
* Full constructor.
*
* @param stargateHttpClient http client
* @param namespaceClient NamespaceClient
* @param collectionName String
*/
public CollectionClient(StargateHttpClient stargateHttpClient, NamespaceClient namespaceClient, String collectionName) {
this.namespaceClient = namespaceClient;
this.collectionName = collectionName;
this.stargateHttpClient = stargateHttpClient;
}
/**
* Get metadata of the collection. There is no dedicated resources we
* use the list and filter with what we need.
*
* @see Reference Documentation
*
* @return
* metadata of the collection if its exist or empty
*/
public Optional find() {
return namespaceClient.collections()
.filter(c -> collectionName.equalsIgnoreCase(c.getName()))
.findFirst();
}
/**
* Check if the collection exist.
*
* @return if the collection exists.
*/
public boolean exist() {
return namespaceClient.collectionNames()
.anyMatch(collectionName::equals);
}
/**
* Create the collection.
*
* @see Reference Documentation
*/
public void create() {
stargateHttpClient.POST(collectionResource,
"{\"name\":\"" + collectionName + "\"}");
}
/**
* Upgrade the collection.
* This is not relevant in ASTRA.
*/
public void upgradeSAI() {
upgrade(CollectionUpgradeType.SAI_INDEX_UPGRADE);
}
/**
* Update a collection to SAI.
*
* @param index
* collection SAI
*/
public void upgrade(CollectionUpgradeType index) {
stargateHttpClient.POST(collectionUpgradeResource,
"{\"upgradeType\":\"" + index.name() + "\"}");
}
/**
* Deleting the collection
*
* @see Reference Documentation
*/
public void delete() {
stargateHttpClient.DELETE(collectionResource);
}
/**
* Create a new document from any serializable object.
*
* @see Reference Documentation
*
* @param
* working bean type
* @param doc
* working bean instance
* @return
* created document id
*/
public String create(DOC doc) {
ApiResponseHttp res = stargateHttpClient.POST(collectionResource, marshall(doc));
try {
return (String) unmarshallBean(res.getBody(), Map.class)
.get(DOCUMENT_ID);
} catch (Exception e) {
throw new RuntimeException("Cannot marshall document id", e);
}
}
/**
* Use the resource batch to insert massively in the DB.
*
* @param records
* list of records
* @param idPath
* id path to enforced ids
* @param
* working document
* @return
* list of inserted ids
*/
@SuppressWarnings("unchecked")
public List batchInsert(List records, String idPath) {
Assert.notNull(records, "Records should be provided");
ApiResponseHttp res;
if (Utils.hasLength(idPath)) {
res = stargateHttpClient.POST(collectionBatchResource, marshall(records),
"?" + BATCH_ID_PATH + "=" + idPath);
} else {
res = stargateHttpClient.POST(collectionBatchResource, marshall(records));
}
Map doc = unmarshallBean(res.getBody(), Map.class);
if (doc.containsKey("documentIds")) {
return (List) doc.get("documentIds");
}
return new ArrayList<>();
}
/**
* Insert multiple record with a single resource.
*
* @param records
* list of records
* @param
* working document
* @return
* list of inserted ids
*/
public List batchInsert(List records) {
return batchInsert(records, null);
}
/**
* Count items in a collection, it can be slow as we iterate over pages limitating
* payload and marshalling as much as possible.
*
* @return
* number of record
*/
public int count() {
AtomicInteger count = new AtomicInteger(0);
// Invalid field provided for list of ids only
SearchDocumentQuery query = SearchDocumentQuery
.builder().select("field_not_exist")
.withPageSize(2).build();
ApiResponse
© 2015 - 2025 Weber Informatics LLC | Privacy Policy