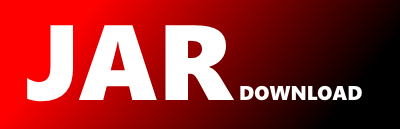
com.datastax.stargate.sdk.doc.StargateDocumentRepository Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of stargate-sdk Show documentation
Show all versions of stargate-sdk Show documentation
Connect to Stargate Data Gateway
package com.datastax.stargate.sdk.doc;
import java.util.Optional;
import java.util.stream.Stream;
import com.datastax.stargate.sdk.StargateClient;
import com.datastax.stargate.sdk.doc.domain.DocumentResultPage;
import com.datastax.stargate.sdk.doc.domain.SearchDocumentQuery;
/**
* Super class to help you working with the document API.
*
* @author Cedrick LUNVEN (@clunven)
*
* @param
* current document bean.
*/
public class StargateDocumentRepository {
/** Reference to underlying collection client. **/
private final CollectionClient collectionClient;
/** Keep ref to the generic. */
private final Class docClass;
/**
* Default constructor.
*
* @param col
* collection client parent
* @param clazz
* working bean class
*/
public StargateDocumentRepository(CollectionClient col, Class clazz) {
this.collectionClient = col;
this.docClass = clazz;
}
/**
* Constructor from {@link StargateClient}.
*
* @param nc
* reference to the StargateClient
* @param clazz
* working namespace identifier
*/
public StargateDocumentRepository(NamespaceClient nc, Class clazz) {
this.docClass = clazz;
this.collectionClient = new CollectionClient(nc.stargateHttpClient, nc, getCollectionName(clazz));
}
/**
* Count items in the collection.
*
* @return
* number of records.
*/
public int count() {
return collectionClient.count();
}
/**
* Delete a document from its iid.
*
* @param docId
* document identifier
*/
public void delete(String docId) {
collectionClient.document(docId).delete();;
}
/**
* Check existence of a document from its id.
*
* @param docId
* document identifier
* @return
* existence status
*/
public boolean exists(String docId) {
return collectionClient.document(docId).exist();
}
/**
* Create a new document an generating identifier.
*
* @param p
* working document
* @return
* an unique identifier for the document
*/
public String insert(DOC p) {
return collectionClient.create(p);
}
/**
* Create if not exist with defined ID.
*
* @param docId
* expected document id
* @param doc
* document
*/
public void insert(String docId, DOC doc) {
collectionClient.document(docId).upsert(doc);
}
/**
* Create if not exist with defined ID.
*
* @param docId
* expected document id
* @param doc
* document
*/
public void save(String docId, DOC doc) {
collectionClient.document(docId).upsert(doc);
}
/**
* Evaluation on which collection we are working.
*
* @return
* collection identifier
*/
public String getCollectionName() {
return collectionClient.getCollectionName();
}
/**
* Find a person from ids unique identifier.
*
* @param docId
* document Id
* @return
* the object only if present
*/
public Optional find(String docId) {
return collectionClient.document(docId).find(docClass);
}
/**
* Search document based on a search query
*
* @param query
* current query
* @return
* all the element matching
*/
public Stream> search(SearchDocumentQuery query) {
return collectionClient.findAll(query, docClass);
}
/**
* Search document with attributes.
*
* @param query
* current query
* @return
* result page
*/
public DocumentResultPage searchPage(SearchDocumentQuery query) {
return collectionClient.findPage(query, docClass);
}
/**
* Retrieve all documents from the collection.
*
* @return
* every document of the collection
*/
public Stream> findAll() {
return collectionClient.findAll(docClass);
}
/**
* Find first page.
*
* @return
* first page of the collection
*/
public DocumentResultPage findFirstPage() {
return collectionClient.findFirstPage(docClass);
}
/**
* Find first page.
*
* @param pageSize
* size of page
* @return
* first page of the collection
*/
public DocumentResultPage findFirstPage(int pageSize){
return collectionClient.findFirstPage(docClass, pageSize);
}
/**
* Find page.
*
* @param pageSize
* size of page
* @param pageingState
* cursor to retrieve pages
* @return
* first page of the collection
*/
public DocumentResultPage findPage(int pageSize, String pageingState){
return collectionClient.findPage(docClass, pageSize, pageingState);
}
/**
* Read Collection Name.
*
* @param myClass
* my current class
* @return
* name of the collection
*/
private String getCollectionName(Class myClass) {
Collection ann = myClass.getAnnotation(Collection.class);
if (null != ann && ann.value() !=null && !ann.value().equals("")) {
return ann.value();
} else {
return myClass.getSimpleName().toLowerCase();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy