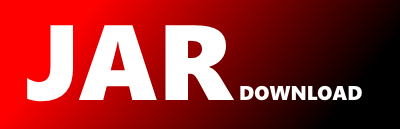
com.datastax.stargate.sdk.grpc.domain.ResultSetGrpc Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of stargate-sdk Show documentation
Show all versions of stargate-sdk Show documentation
Connect to Stargate Data Gateway
package com.datastax.stargate.sdk.grpc.domain;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.stream.Collectors;
import io.stargate.proto.QueryOuterClass.ColumnSpec;
import io.stargate.proto.QueryOuterClass.ResultSet;
import io.stargate.proto.QueryOuterClass.Row;
/**
* Helper to parse the grpoc output.
*
* @author Cedrick LUNVEN (@clunven)
*/
public class ResultSetGrpc {
/** Object returned by the grpc.*/
private final ResultSet rs;
/** Index columns names. */
private final List columnsNames = new ArrayList<>();
/** Access one column in particular. */
private final Map columnsSpecs = new HashMap<>();
/** Access one column in particular. */
private final Map columnsIndexes = new HashMap<>();
/**
* Constructor for the wrapper.
*
* @param rs
* resultset
*/
public ResultSetGrpc(ResultSet rs) {
this.rs = rs;
for (int i=0; i rs.getRowsCount()) {
throw new IllegalArgumentException("Resulset contains only " + rs.getRowsCount() + " row(s).");
}
return rs.getRowsList().get(idx);
}
/**
* Access Rows.
*
* @return
* list if items
*/
public List getRows() {
return rs.getRowsList()
.stream()
.map(r -> new RowGrpc(this, r))
.collect(Collectors.toList());
}
/**
* Accessor for internal object.
*
* @return
* internal object
*/
public ResultSet getResultSet() {
return rs;
}
/**
* Access column index based on offset.
*
* @param name
* column name
* @return
* column index
*/
public int getColumnIndex(String name) {
if (!columnsIndexes.containsKey(name)) {
throw new IllegalArgumentException("Column '" + name + "' is unknown, use " + columnsNames);
}
return columnsIndexes.get(name);
}
/**
* Return column name based on index.
* @param idx
* column index
* @return
* column name
*/
public String getColumnName(int idx) {
if (idx > columnsNames.size()) {
throw new IllegalArgumentException("Invalid index, only '"
+ columnsNames.size() + "' size available");
}
return columnsNames.get(idx);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy