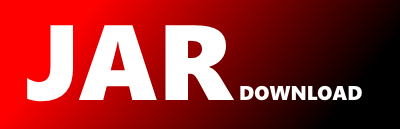
com.datastrato.gravitino.rel.expressions.literals.Literals Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of api Show documentation
Show all versions of api Show documentation
Gravitino is a high-performance, geo-distributed and federated metadata lake.
The newest version!
/*
* Copyright 2024 Datastrato Pvt Ltd.
* This software is licensed under the Apache License version 2.
*/
package com.datastrato.gravitino.rel.expressions.literals;
import com.datastrato.gravitino.rel.types.Decimal;
import com.datastrato.gravitino.rel.types.Type;
import com.datastrato.gravitino.rel.types.Types;
import java.time.LocalDate;
import java.time.LocalDateTime;
import java.time.LocalTime;
import java.util.Objects;
/** The helper class to create literals to pass into Gravitino. */
public class Literals {
/** Used to represent a null literal. */
public static final Literal NULL = new LiteralImpl<>(null, Types.NullType.get());
/**
* Creates a literal with the given value and data type.
*
* @param value the literal value
* @param dataType the data type of the literal
* @param the JVM type of value held by the literal
* @return a new {@link com.datastrato.gravitino.rel.expressions.Literal} instance
*/
public static LiteralImpl of(T value, Type dataType) {
return new LiteralImpl<>(value, dataType);
}
/**
* Creates a boolean type literal with the given value.
*
* @param value the boolean literal value
* @return a new {@link Literal} instance
*/
public static LiteralImpl booleanLiteral(Boolean value) {
return of(value, Types.BooleanType.get());
}
/**
* Creates a byte type literal with the given value.
*
* @param value the byte literal value
* @return a new {@link Literal} instance
*/
public static LiteralImpl byteLiteral(Byte value) {
return of(value, Types.ByteType.get());
}
/**
* Creates a short type literal with the given value.
*
* @param value the short literal value
* @return a new {@link Literal} instance
*/
public static LiteralImpl shortLiteral(Short value) {
return of(value, Types.ShortType.get());
}
/**
* Creates an integer type literal with the given value.
*
* @param value the integer literal value
* @return a new {@link Literal} instance
*/
public static LiteralImpl integerLiteral(Integer value) {
return of(value, Types.IntegerType.get());
}
/**
* Creates a long type literal with the given value.
*
* @param value the long literal value
* @return a new {@link Literal} instance
*/
public static LiteralImpl longLiteral(Long value) {
return of(value, Types.LongType.get());
}
/**
* Creates a float type literal with the given value.
*
* @param value the float literal value
* @return a new {@link Literal} instance
*/
public static LiteralImpl floatLiteral(Float value) {
return of(value, Types.FloatType.get());
}
/**
* Creates a double type literal with the given value.
*
* @param value the double literal value
* @return a new {@link Literal} instance
*/
public static LiteralImpl doubleLiteral(Double value) {
return of(value, Types.DoubleType.get());
}
/**
* Creates a decimal type literal with the given value.
*
* @param value the decimal literal value
* @return a new {@link Literal} instance
*/
public static LiteralImpl decimalLiteral(Decimal value) {
return of(value, Types.DecimalType.of(value.precision(), value.scale()));
}
/**
* Creates a date type literal with the given value.
*
* @param value the date literal value
* @return a new {@link Literal} instance
*/
public static LiteralImpl dateLiteral(LocalDate value) {
return of(value, Types.DateType.get());
}
/**
* Creates a time type literal with the given value.
*
* @param value the time literal value
* @return a new {@link Literal} instance
*/
public static LiteralImpl timeLiteral(LocalTime value) {
return of(value, Types.TimeType.get());
}
/**
* Creates a timestamp type literal with the given value.
*
* @param value the timestamp literal value
* @return a new {@link Literal} instance
*/
public static LiteralImpl timestampLiteral(LocalDateTime value) {
return of(value, Types.TimestampType.withoutTimeZone());
}
/**
* Creates a timestamp type literal with the given value.
*
* @param value the timestamp literal value, must be in the format "yyyy-MM-ddTHH:mm:ss"
* @return a new {@link Literal} instance
*/
public static LiteralImpl timestampLiteral(String value) {
return timestampLiteral(LocalDateTime.parse(value));
}
/**
* Creates a string type literal with the given value.
*
* @param value the string literal value
* @return a new {@link Literal} instance
*/
public static LiteralImpl stringLiteral(String value) {
return of(value, Types.StringType.get());
}
/**
* Creates a varchar type literal with the given value.
*
* @param value the string literal value
* @param length the length of the varchar
* @return a new {@link Literal} instance
*/
public static LiteralImpl varcharLiteral(int length, String value) {
return of(value, Types.VarCharType.of(length));
}
/**
* Creates a literal with the given type value.
*
* @param The JVM type of value held by the literal.
*/
public static final class LiteralImpl implements Literal {
private final T value;
private final Type dataType;
private LiteralImpl(T value, Type dataType) {
this.value = value;
this.dataType = dataType;
}
@Override
public T value() {
return value;
}
@Override
public Type dataType() {
return dataType;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
LiteralImpl> literal = (LiteralImpl>) o;
if (!Objects.equals(dataType, literal.dataType)) {
return false;
}
// Check both values for null before comparing to avoid NullPointerException
if (value == null || literal.value == null) {
return Objects.equals(value, literal.value);
}
// Now, it's safe to compare using toString() since neither value is null
return Objects.equals(value, literal.value)
|| value.toString().equals(literal.value.toString());
}
@Override
public int hashCode() {
return Objects.hash(dataType, value != null ? value.toString() : null);
}
@Override
public String toString() {
return "LiteralImpl{" + "value=" + value + ", dataType=" + dataType + '}';
}
}
private Literals() {}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy