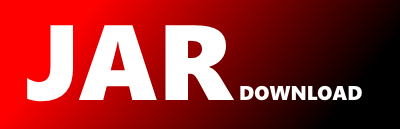
com.datastrato.gravitino.EntityStore Maven / Gradle / Ivy
Show all versions of core Show documentation
/*
* Copyright 2023 Datastrato Pvt Ltd.
* This software is licensed under the Apache License version 2.
*/
package com.datastrato.gravitino;
import com.datastrato.gravitino.Entity.EntityType;
import com.datastrato.gravitino.exceptions.AlreadyExistsException;
import com.datastrato.gravitino.exceptions.NoSuchEntityException;
import com.datastrato.gravitino.utils.Executable;
import java.io.Closeable;
import java.io.IOException;
import java.util.List;
import java.util.function.Function;
public interface EntityStore extends Closeable {
/**
* Initialize the entity store.
*
* Note. This method will be called after the EntityStore object is created, and before any
* other methods are called.
*
* @param config the configuration for the entity store
* @throws RuntimeException if the initialization fails
*/
void initialize(Config config) throws RuntimeException;
/**
* Set the {@link EntitySerDe} for the entity store. {@link EntitySerDe} will be used to serialize
* and deserialize the entities to the target format, and vice versa.
*
* @param entitySerDe the entity serde to set
*/
void setSerDe(EntitySerDe entitySerDe);
/**
* List all the entities with the specified {@link Namespace}, and deserialize them into the
* specified {@link Entity} object.
*
*
Note. Depends on the isolation levels provided by the underlying storage, the returned list
* may not be consistent.
*
* @param namespace the namespace of the entities
* @param class of the entity
* @param type the detailed type of the entity
* @param entityType the general type of the entity
* @throws IOException if the list operation fails
* @return the list of entities
*/
List list(
Namespace namespace, Class type, EntityType entityType) throws IOException;
/**
* Check if the entity with the specified {@link NameIdentifier} exists.
*
* @param ident the name identifier of the entity
* @param entityType the general type of the entity,
* @return true if the entity exists, false otherwise
* @throws IOException if the check operation fails
*/
boolean exists(NameIdentifier ident, EntityType entityType) throws IOException;
/**
* Store the entity into the underlying storage. If the entity already exists, it will overwrite
* the existing entity.
*
* @param e the entity to store
* @param the type of the entity
* @throws IOException if the store operation fails
*/
default void put(E e) throws IOException {
put(e, false);
}
/**
* Store the entity into the underlying storage. According to the {@code overwritten} flag, it
* will overwrite the existing entity or throw an {@link EntityAlreadyExistsException}.
*
* Note. The implementation should be transactional, and should be able to handle concurrent
* store of entities.
*
* @param e the entity to store
* @param overwritten whether to overwrite the existing entity
* @param the type of the entity
* @throws IOException if the store operation fails
* @throws EntityAlreadyExistsException if the entity already exists and the overwritten flag is
* set to false
*/
void put(E e, boolean overwritten)
throws IOException, EntityAlreadyExistsException;
/**
* Update the entity into the underlying storage.
*
* Note: the {@link NameIdentifier} of the updated entity may be changed. Based on the design
* of the storage key, the implementation may have two implementations.
*
*
1) if the storage key is name relevant, it needs to delete the old entity and store the new
* entity with the new key. 2) or if the storage key is name irrelevant, it can just store the new
* entity with the current key.
*
*
Note: the whole update operation should be in one transaction.
*
* @param ident the name identifier of the entity
* @param type the detailed type of the entity
* @param updater the updater function to update the entity
* @param the class of the entity
* @param entityType the general type of the entity
* @return E the updated entity
* @throws IOException if the store operation fails
* @throws NoSuchEntityException if the entity does not exist
* @throws AlreadyExistsException if the updated entity already existed.
*/
E update(
NameIdentifier ident, Class type, EntityType entityType, Function updater)
throws IOException, NoSuchEntityException, AlreadyExistsException;
/**
* Get the entity from the underlying storage.
*
* Note. The implementation should be thread-safe, and should be able to handle concurrent
* retrieve of entities.
*
* @param ident the unique identifier of the entity
* @param entityType the general type of the entity
* @param e the entity class instance
* @param the class of entity
* @return the entity retrieved from the underlying storage
* @throws NoSuchEntityException if the entity does not exist
* @throws IOException if the retrieve operation fails
*/
E get(NameIdentifier ident, EntityType entityType, Class e)
throws NoSuchEntityException, IOException;
/**
* Delete the entity from the underlying storage by the specified {@link NameIdentifier}.
*
* @param ident the name identifier of the entity
* @param entityType the type of the entity to be deleted
* @return true if the entity exists and is deleted successfully, false otherwise
* @throws IOException if the delete operation fails
*/
default boolean delete(NameIdentifier ident, EntityType entityType) throws IOException {
return delete(ident, entityType, false);
}
/**
* Delete the entity from the underlying storage by the specified {@link NameIdentifier}.
*
* @param ident the name identifier of the entity
* @param entityType the type of the entity to be deleted
* @param cascade support cascade delete or not
* @return true if the entity exists and is deleted successfully, false otherwise
* @throws IOException if the delete operation fails
*/
boolean delete(NameIdentifier ident, EntityType entityType, boolean cascade) throws IOException;
/**
* Execute the specified {@link Executable} in a transaction.
*
* @param executable the executable to run
* @param the type of the return value
* @param the type of the exception
* @return the return value of the executable
* @throws IOException if the execution fails
* @throws E if the execution fails
*/
R executeInTransaction(Executable executable)
throws E, IOException;
}