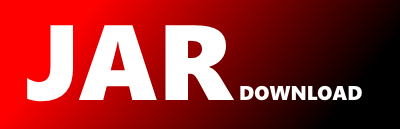
com.datumbox.framework.common.concurrency.ForkJoinStream Maven / Gradle / Ivy
/**
* Copyright (C) 2013-2016 Vasilis Vryniotis
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.datumbox.framework.common.concurrency;
import java.util.Comparator;
import java.util.Optional;
import java.util.concurrent.Callable;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collector;
import java.util.stream.DoubleStream;
import java.util.stream.Stream;
/**
* This class can be used to process a Stream in parallel using a custom ForkJoinPool.
*
* @author Vasilis Vryniotis
*/
public class ForkJoinStream {
private final ConcurrencyConfiguration concurrencyConfig;
/**
* Default constructor which receives the Concurrency Configuration object.
*
* @param concurrencyConfig
*/
public ForkJoinStream(ConcurrencyConfiguration concurrencyConfig) {
this.concurrencyConfig = concurrencyConfig;
}
/**
* Executes forEach on the provided stream. If the Stream is parallel, it is
* executed using the custom pool, else it is executed directly from the
* main thread.
*
* @param
* @param stream
* @param action
*/
public void forEach(Stream stream, Consumer super T> action) {
Runnable runnable = () -> stream.forEach(action);
ThreadMethods.forkJoinExecution(runnable, concurrencyConfig, stream.isParallel());
}
/**
* Executes map on the provided stream. If the Stream is parallel, it is
* executed using the custom pool, else it is executed directly from the
* main thread.
*
* @param
* @param
* @param stream
* @param mapper
* @return
*/
public Stream map(Stream stream, Function super T, ? extends R> mapper) {
Callable> callable = () -> stream.map(mapper);
return ThreadMethods.forkJoinExecution(callable, concurrencyConfig, stream.isParallel());
}
/**
* Executes collect on the provided stream using the provided collector.
* If the Stream is parallel, it is executed using the custom pool, else it
* is executed directly from the main thread.
*
* @param
* @param
* @param
* @param stream
* @param collector
* @return
*/
public R collect(Stream stream, Collector super T, A, R> collector) {
Callable callable = () -> stream.collect(collector);
return ThreadMethods.forkJoinExecution(callable, concurrencyConfig, stream.isParallel());
}
/**
* Executes min on the provided stream using the provided collector.
* If the Stream is parallel, it is executed using the custom pool, else it
* is executed directly from the main thread.
*
* @param
* @param stream
* @param comparator
* @return
*/
public Optional min(Stream stream, Comparator super T> comparator) {
Callable> callable = () -> stream.min(comparator);
return ThreadMethods.forkJoinExecution(callable, concurrencyConfig, stream.isParallel());
}
/**
* Executes max on the provided stream using the provided collector.
* If the Stream is parallel, it is executed using the custom pool, else it
* is executed directly from the main thread.
*
* @param
* @param stream
* @param comparator
* @return
*/
public Optional max(Stream stream, Comparator super T> comparator) {
Callable> callable = () -> stream.max(comparator);
return ThreadMethods.forkJoinExecution(callable, concurrencyConfig, stream.isParallel());
}
/**
* Executes sum on the provided DoubleStream using the provided collector.
* If the Stream is parallel, it is executed using the custom pool, else it
* is executed directly from the main thread.
*
* @param stream
* @return
*/
public double sum(DoubleStream stream) {
Callable callable = () -> stream.sum();
return ThreadMethods.forkJoinExecution(callable, concurrencyConfig, stream.isParallel());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy