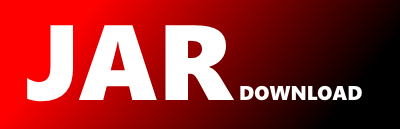
com.datumbox.framework.common.utilities.MapMethods Maven / Gradle / Ivy
/**
* Copyright (C) 2013-2016 Vasilis Vryniotis
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.datumbox.framework.common.utilities;
import com.datumbox.framework.common.dataobjects.AssociativeArray;
import com.datumbox.framework.common.dataobjects.TypeInference;
import java.util.*;
/**
* The MapMethods class contains a list of convenience methods for Maps and
AssociativeArrays.
*
* @author Vasilis Vryniotis
*/
public class MapMethods {
/**
* Selects the key-value entry with the largest value.
*
* @param keyValueMap
* @return
*/
public static Map.Entry
© 2015 - 2025 Weber Informatics LLC | Privacy Policy