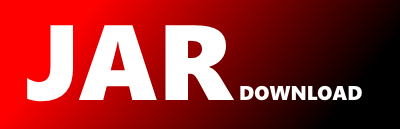
com.datumbox.framework.common.utilities.PHPMethods Maven / Gradle / Ivy
/**
* Copyright (C) 2013-2016 Vasilis Vryniotis
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.datumbox.framework.common.utilities;
import org.apache.commons.lang3.builder.ToStringBuilder;
import java.util.*;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
/**
* This class contains a number of convenience methods which have an API similar
* to PHP functions and their are implemented in Java.
*
* @author Vasilis Vryniotis
*/
public class PHPMethods {
private static final Pattern LTRIM = Pattern.compile("^\\s+");
private static final Pattern RTRIM = Pattern.compile("\\s+$");
/**
* Trims spaces on the left.
*
* @param s
* @return
*/
public static String ltrim(String s) {
return LTRIM.matcher(s).replaceAll("");
}
/**
* Trims spaces on the right.
*
* @param s
* @return
*/
public static String rtrim(String s) {
return RTRIM.matcher(s).replaceAll("");
}
/**
* Count the number of substring occurrences.
*
* @param string
* @param substring
* @return
*/
public static int substr_count(final String string, final String substring) {
if(substring.length()==1) {
return substr_count(string, substring.charAt(0));
}
int count = 0;
int idx = 0;
while ((idx = string.indexOf(substring, idx)) != -1) {
++idx;
++count;
}
return count;
}
/**
* Count the number of times a character appears in the string.
*
* @param string
* @param character
* @return
*/
public static int substr_count(final String string, final char character) {
int count = 0;
int n = string.length();
for(int i=0;i
* @param
* @param map
* @return
*/
public static Map array_flip(Map map) {
Map flipped = new HashMap<>();
for(Map.Entry entry : map.entrySet()) {
flipped.put(entry.getValue(), entry.getKey());
}
return flipped;
}
/**
* Shuffles the values of any array in place.
*
* @param
* @param array
*/
public static void shuffle(T[] array) {
//Implementing Fisher-Yates shuffle
T tmp;
Random rnd = RandomGenerator.getThreadLocalRandom();
for (int i = array.length - 1; i > 0; --i) {
int index = rnd.nextInt(i + 1);
tmp = array[index];
array[index] = array[i];
array[i] = tmp;
}
}
/**
* Returns the contexts of an Objects in a human readable format.
*
* @param
* @param object
* @return
*/
public static String var_export(T object) {
return ToStringBuilder.reflectionToString(object);
}
/**
* Sorts an array in ascending order and returns an array with indexes of
* the original order.
*
* @param
* @param array
* @return
*/
public static > Integer[] asort(T[] array) {
return _asort(array, false);
}
/**
* Sorts an array in descending order and returns an array with indexes of
* the original order.
*
* @param
* @param array
* @return
*/
public static > Integer[] arsort(T[] array) {
return _asort(array, true);
}
private static > Integer[] _asort(T[] array, boolean reverse) {
//create an array with the indexes
Integer[] indexes = new Integer[array.length];
for (int i = 0; i < array.length; ++i) {
indexes[i] = i;
}
//sort the indexes first
Comparator c = (Integer index1, Integer index2) -> array[index1].compareTo(array[index2]);
c = reverse?Collections.reverseOrder(c):c;
Arrays.sort(indexes, c);
//rearrenage the array based on the order of indexes
arrangeByIndex(array, indexes);
return indexes;
}
/**
* Rearranges the array based on the order of the provided indexes.
*
* @param
* @param array
* @param indexes
*/
public static void arrangeByIndex(T[] array, Integer[] indexes) {
if(array.length != indexes.length) {
throw new IllegalArgumentException("The length of the two arrays must match.");
}
//sort the array based on the indexes
for(int i=0;i
© 2015 - 2025 Weber Informatics LLC | Privacy Policy