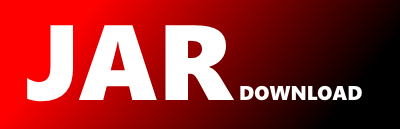
com.deepoove.poi.resolver.RunningRunParagraph Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of poi-tl Show documentation
Show all versions of poi-tl Show documentation
Generate word(docx) with template
/*
* Copyright 2014-2019 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.deepoove.poi.resolver;
import java.util.ArrayList;
import java.util.List;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
import javax.xml.namespace.QName;
import org.apache.commons.lang3.tuple.ImmutablePair;
import org.apache.commons.lang3.tuple.Pair;
import org.apache.poi.xwpf.usermodel.XWPFParagraph;
import org.apache.poi.xwpf.usermodel.XWPFRun;
import org.apache.xmlbeans.QNameSet;
import org.openxmlformats.schemas.wordprocessingml.x2006.main.CTBr;
import org.openxmlformats.schemas.wordprocessingml.x2006.main.CTEmpty;
import org.openxmlformats.schemas.wordprocessingml.x2006.main.CTR;
import org.openxmlformats.schemas.wordprocessingml.x2006.main.CTText;
import org.openxmlformats.schemas.wordprocessingml.x2006.main.impl.CTRImpl;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.deepoove.poi.util.StyleUtils;
public class RunningRunParagraph {
private static final Logger LOGGER = LoggerFactory.getLogger(RunningRunParagraph.class);
static QNameSet qname = QNameSet.forArray(new QName[] {
new QName("http://schemas.openxmlformats.org/wordprocessingml/2006/main", "br"),
new QName("http://schemas.openxmlformats.org/wordprocessingml/2006/main", "t"),
new QName("http://schemas.openxmlformats.org/wordprocessingml/2006/main", "cr") });
private XWPFParagraph paragraph;
private List runs;
List> pairs = new ArrayList>();
public RunningRunParagraph(XWPFParagraph paragraph, Pattern pattern) {
this.paragraph = paragraph;
this.runs = paragraph.getRuns();
if (null == runs || runs.isEmpty()) return;
Matcher matcher = pattern.matcher(paragraph.getText());
if (matcher.find()) {
refactorParagraph();
}
buildRunEdge(pattern);
}
public List refactorRun() {
if (pairs.isEmpty()) return null;
List templateRuns = new ArrayList();
int size = pairs.size();
Pair runEdgePair;
for (int n = size - 1; n >= 0; n--) {
runEdgePair = pairs.get(n);
RunEdge startEdge = runEdgePair.getLeft();
RunEdge endEdge = runEdgePair.getRight();
int startRunPos = startEdge.getRunPos();
int endRunPos = endEdge.getRunPos();
int startOffset = startEdge.getRunEdge();
int endOffset = endEdge.getRunEdge();
// String startText = runs.get(startRunPos).getText(0);
// String endText = runs.get(endRunPos).getText(0);
String startText = runs.get(startRunPos).text();
String endText = runs.get(endRunPos).text();
if (endOffset + 1 >= endText.length()) {
// end run 无需split,若不是start run,直接remove
if (startRunPos != endRunPos) paragraph.removeRun(endRunPos);
} else {
// split end run, set extra in a run
String extra = endText.substring(endOffset + 1, endText.length());
if (startRunPos == endRunPos) {
XWPFRun extraRun = paragraph.insertNewRun(endRunPos + 1);
StyleUtils.styleRun(extraRun, runs.get(endRunPos));
buildExtra(extra, extraRun);
} else {
XWPFRun extraRun = runs.get(endRunPos);
buildExtra(extra, extraRun);
}
}
// remove extra run
for (int m = endRunPos - 1; m > startRunPos; m--) {
paragraph.removeRun(m);
}
if (startOffset <= 0) {
// start run 无需split
XWPFRun templateRun = runs.get(startRunPos);
templateRun.setText(startEdge.getTag(), 0);
templateRuns.add(runs.get(startRunPos));
} else {
// split start run, set extra in a run
String extra = startText.substring(0, startOffset);
XWPFRun extraRun = runs.get(startRunPos);
buildExtra(extra, extraRun);// TODO
XWPFRun templateRun = paragraph.insertNewRun(startRunPos + 1);
StyleUtils.styleRun(templateRun, extraRun);
templateRun.setText(startEdge.getTag(), 0);
templateRuns.add(runs.get(startRunPos + 1));
}
}
return templateRuns;
}
private void buildExtra(String extra, XWPFRun extraRun) {
extraRun.setText(extra, 0);
}
private void refactorParagraph() {
for (int i = runs.size() - 1; i >= 0; i--) {
XWPFRun xwpfRun = runs.get(i);
CTR ctr = xwpfRun.getCTR();
CTRImpl ctrimpl = (CTRImpl) ctr;
int sizeOfBrArray = ctr.sizeOfBrArray();
int sizeOfCrArray = ctr.sizeOfCrArray();
if ((sizeOfBrArray + sizeOfCrArray) > 0) {
synchronized (ctrimpl.monitor()) {
// ctrimpl.check_orphaned();
ArrayList
© 2015 - 2025 Weber Informatics LLC | Privacy Policy