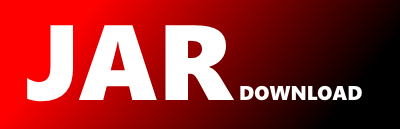
com.deepoove.swagger.diff.compare.MapKeyDiff Maven / Gradle / Ivy
package com.deepoove.swagger.diff.compare;
import java.util.ArrayList;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import java.util.Map.Entry;
public class MapKeyDiff {
private Map increased;
private Map missing;
private List sharedKey;
private MapKeyDiff() {
this.sharedKey = new ArrayList();
}
public static MapKeyDiff diff(Map mapLeft,
Map mapRight) {
MapKeyDiff instance = new MapKeyDiff();
if (null == mapLeft && null == mapRight) return instance;
if (null == mapLeft) {
instance.increased = mapRight;
return instance;
}
if (null == mapRight) {
instance.missing = mapLeft;
return instance;
}
instance.increased = new LinkedHashMap(mapRight);
instance.missing = new LinkedHashMap();
for (Entry entry : mapLeft.entrySet()) {
K leftKey = entry.getKey();
V leftValue = entry.getValue();
if (mapRight.containsKey(leftKey)) {
instance.increased.remove(leftKey);
instance.sharedKey.add(leftKey);
} else {
instance.missing.put(leftKey, leftValue);
}
}
return instance;
}
public Map getIncreased() {
return increased;
}
public Map getMissing() {
return missing;
}
public List getSharedKey() {
return sharedKey;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy