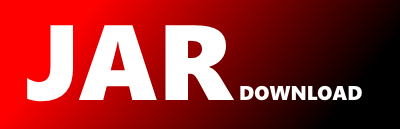
com.deliver8r.aws.io.snakeyaml.AWSResourcesSnakeYamlReader Maven / Gradle / Ivy
// =================== DO NOT EDIT THIS FILE ====================
// Generated by Modello 1.8.3,
// any modifications will be overwritten.
// ==============================================================
package com.deliver8r.aws.io.snakeyaml;
//---------------------------------/
//- Imported classes and packages -/
//---------------------------------/
import com.deliver8r.aws.AwsInstances;
import com.deliver8r.aws.Instance;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.io.Reader;
import java.text.DateFormat;
import java.util.HashSet;
import java.util.Set;
import org.yaml.snakeyaml.events.DocumentEndEvent;
import org.yaml.snakeyaml.events.DocumentStartEvent;
import org.yaml.snakeyaml.events.Event;
import org.yaml.snakeyaml.events.ImplicitTuple;
import org.yaml.snakeyaml.events.MappingEndEvent;
import org.yaml.snakeyaml.events.MappingStartEvent;
import org.yaml.snakeyaml.events.ScalarEvent;
import org.yaml.snakeyaml.events.SequenceEndEvent;
import org.yaml.snakeyaml.events.SequenceStartEvent;
import org.yaml.snakeyaml.events.StreamEndEvent;
import org.yaml.snakeyaml.events.StreamStartEvent;
import org.yaml.snakeyaml.parser.Parser;
import org.yaml.snakeyaml.parser.ParserException;
import org.yaml.snakeyaml.parser.ParserImpl;
import org.yaml.snakeyaml.reader.StreamReader;
/**
* Class AWSResourcesSnakeYamlReader.
*
* @version $Revision$ $Date$
*/
@SuppressWarnings( "all" )
public class AWSResourcesSnakeYamlReader
{
//-----------/
//- Methods -/
//-----------/
/**
* Method checkFieldWithDuplicate.
*
* @param event
* @param parsed
* @param alias
* @param tagName
* @throws IOException
* @return boolean
*/
private boolean checkFieldWithDuplicate( Event event, String tagName, String alias, Set parsed )
throws IOException
{
String currentName = ( (ScalarEvent) event ).getValue();
if ( !( currentName.equals( tagName ) || currentName.equals( alias ) ) )
{
return false;
}
if ( !parsed.add( tagName ) )
{
throw new ParserException( "Duplicated tag: '" + tagName + "'", event.getStartMark(), "", null );
}
return true;
} //-- boolean checkFieldWithDuplicate( Event, String, String, Set )
/**
* Method checkUnknownAttribute.
*
* @param parser
* @param strict
* @param tagName
* @param attribute
* @throws IOException
*/
private void checkUnknownAttribute( Parser parser, String attribute, String tagName, boolean strict )
throws IOException
{
// strictXmlAttributes = true for model: if strict == true, not only elements are checked but attributes too
if ( strict )
{
throw new ParserException( "", parser.peekEvent().getStartMark(), "Unknown attribute '" + attribute + "' for tag '" + tagName + "'", parser.peekEvent().getEndMark() );
}
} //-- void checkUnknownAttribute( Parser, String, String, boolean )
/**
* Method checkUnknownElement.
*
* @param event
* @param strict
* @param parser
* @throws IOException
*/
private void checkUnknownElement( Event event, Parser parser, boolean strict )
throws IOException
{
if ( strict )
{
throw new ParserException( "Unrecognised tag: '" + ( (ScalarEvent) event ).getValue() + "'", event.getStartMark(), "", null );
}
for ( int unrecognizedTagCount = 1; unrecognizedTagCount > 0; )
{
event = parser.getEvent();
if ( event.is( Event.ID.MappingStart ) )
{
unrecognizedTagCount++;
}
else if ( event.is( Event.ID.MappingEnd ) )
{
unrecognizedTagCount--;
}
}
} //-- void checkUnknownElement( Event, Parser, boolean )
/**
* Method getBooleanValue.
*
* @param s
* @return boolean
*/
private boolean getBooleanValue( String s )
{
if ( s != null )
{
return Boolean.valueOf( s ).booleanValue();
}
return false;
} //-- boolean getBooleanValue( String )
/**
* Method getByteValue.
*
* @param s
* @param strict
* @param event
* @param attribute
* @return byte
*/
private byte getByteValue( String s, String attribute, Event event, boolean strict )
{
if ( s != null )
{
try
{
return Byte.valueOf( s ).byteValue();
}
catch ( NumberFormatException nfe )
{
if ( strict )
{
throw new ParserException( "", event.getStartMark(), "Unable to parse element '" + attribute + "', must be a byte but was '" + s + "'", event.getEndMark() );
}
}
}
return 0;
} //-- byte getByteValue( String, String, Event, boolean )
/**
* Method getCharacterValue.
*
* @param s
* @return char
*/
private char getCharacterValue( String s )
{
if ( s != null )
{
return s.charAt( 0 );
}
return 0;
} //-- char getCharacterValue( String )
/**
* Method getDateValue.
*
* @param s
* @param event
* @param dateFormat
* @return Date
*/
private java.util.Date getDateValue( String s, String dateFormat, Event event )
{
if ( s != null )
{
String effectiveDateFormat = dateFormat;
if ( dateFormat == null )
{
effectiveDateFormat = "yyyy-MM-dd'T'HH:mm:ss.SSS";
}
if ( "long".equals( effectiveDateFormat ) )
{
try
{
return new java.util.Date( Long.parseLong( s ) );
}
catch ( NumberFormatException e )
{
throw new ParserException( "", event.getStartMark(), e.getMessage(), event.getEndMark() );
}
}
else
{
try
{
DateFormat dateParser = new java.text.SimpleDateFormat( effectiveDateFormat, java.util.Locale.US );
return dateParser.parse( s );
}
catch ( java.text.ParseException e )
{
throw new ParserException( "", event.getStartMark(), e.getMessage(), event.getEndMark() );
}
}
}
return null;
} //-- java.util.Date getDateValue( String, String, Event )
/**
* Method getDefaultValue.
*
* @param s
* @param v
* @return String
*/
private String getDefaultValue( String s, String v )
{
if ( s == null )
{
s = v;
}
return s;
} //-- String getDefaultValue( String, String )
/**
* Method getDoubleValue.
*
* @param s
* @param strict
* @param event
* @param attribute
* @return double
*/
private double getDoubleValue( String s, String attribute, Event event, boolean strict )
{
if ( s != null )
{
try
{
return Double.valueOf( s ).doubleValue();
}
catch ( NumberFormatException nfe )
{
if ( strict )
{
throw new ParserException( "", event.getStartMark(), "Unable to parse element '" + attribute + "', must be a floating point number but was '" + s + "'", event.getEndMark() );
}
}
}
return 0;
} //-- double getDoubleValue( String, String, Event, boolean )
/**
* Method getFloatValue.
*
* @param s
* @param strict
* @param event
* @param attribute
* @return float
*/
private float getFloatValue( String s, String attribute, Event event, boolean strict )
{
if ( s != null )
{
try
{
return Float.valueOf( s ).floatValue();
}
catch ( NumberFormatException nfe )
{
if ( strict )
{
throw new ParserException( "", event.getStartMark(), "Unable to parse element '" + attribute + "', must be a floating point number but was '" + s + "'", event.getEndMark() );
}
}
}
return 0;
} //-- float getFloatValue( String, String, Event, boolean )
/**
* Method getIntegerValue.
*
* @param s
* @param strict
* @param event
* @param attribute
* @return int
*/
private int getIntegerValue( String s, String attribute, Event event, boolean strict )
{
if ( s != null )
{
try
{
return Integer.valueOf( s ).intValue();
}
catch ( NumberFormatException nfe )
{
if ( strict )
{
throw new ParserException( "", event.getStartMark(), "Unable to parse element '" + attribute + "', must be an integer but was '" + s + "'", event.getEndMark() );
}
}
}
return 0;
} //-- int getIntegerValue( String, String, Event, boolean )
/**
* Method getLongValue.
*
* @param s
* @param strict
* @param event
* @param attribute
* @return long
*/
private long getLongValue( String s, String attribute, Event event, boolean strict )
{
if ( s != null )
{
try
{
return Long.valueOf( s ).longValue();
}
catch ( NumberFormatException nfe )
{
if ( strict )
{
throw new ParserException( "", event.getStartMark(), "Unable to parse element '" + attribute + "', must be a long integer but was '" + s + "'", event.getEndMark() );
}
}
}
return 0;
} //-- long getLongValue( String, String, Event, boolean )
/**
* Method getRequiredAttributeValue.
*
* @param s
* @param strict
* @param parser
* @param attribute
* @throws ParserException
* @return String
*/
private String getRequiredAttributeValue( String s, String attribute, Parser parser, boolean strict )
throws ParserException
{
if ( s == null )
{
if ( strict )
{
throw new ParserException( "Missing required value for attribute '" + attribute + "'", parser.peekEvent().getStartMark(), "", null );
}
}
return s;
} //-- String getRequiredAttributeValue( String, String, Parser, boolean )
/**
* Method getShortValue.
*
* @param s
* @param strict
* @param event
* @param attribute
* @return short
*/
private short getShortValue( String s, String attribute, Event event, boolean strict )
{
if ( s != null )
{
try
{
return Short.valueOf( s ).shortValue();
}
catch ( NumberFormatException nfe )
{
if ( strict )
{
throw new ParserException( "", event.getStartMark(), "Unable to parse element '" + attribute + "', must be a short integer but was '" + s + "'", event.getEndMark() );
}
}
}
return 0;
} //-- short getShortValue( String, String, Event, boolean )
/**
* Method getTrimmedValue.
*
* @param s
* @return String
*/
private String getTrimmedValue( String s )
{
if ( s != null )
{
s = s.trim();
}
return s;
} //-- String getTrimmedValue( String )
/**
* Method read.
*
* @param reader
* @param strict
* @throws IOException
* @return AwsInstances
*/
public AwsInstances read( Reader reader, boolean strict )
throws IOException
{
Parser parser = new ParserImpl( new StreamReader( reader ) );
return read( parser, strict );
} //-- AwsInstances read( Reader, boolean )
/**
* Method read.
*
* @param reader
* @throws IOException
* @return AwsInstances
*/
public AwsInstances read( Reader reader )
throws IOException
{
return read( reader, true );
} //-- AwsInstances read( Reader )
/**
* Method read.
*
* @param in
* @param strict
* @throws IOException
* @return AwsInstances
*/
public AwsInstances read( InputStream in, boolean strict )
throws IOException
{
return read( new InputStreamReader( in ), strict );
} //-- AwsInstances read( InputStream, boolean )
/**
* Method read.
*
* @param in
* @throws IOException
* @return AwsInstances
*/
public AwsInstances read( InputStream in )
throws IOException
{
return read( in, true );
} //-- AwsInstances read( InputStream )
/**
* Method parseAwsInstances.
*
* @param parser
* @param strict
* @throws IOException
* @return AwsInstances
*/
private AwsInstances parseAwsInstances( Parser parser, boolean strict )
throws IOException
{
Event event = parser.getEvent();
if ( !event.is( Event.ID.MappingStart ) )
{
throw new ParserException( "Expected 'AwsInstances' data to start with a Mapping", event.getStartMark(), "", null );
}
AwsInstances awsInstances = new AwsInstances();
Set parsed = new HashSet();
while ( !( event = parser.getEvent() ).is( Event.ID.MappingEnd ) )
{
if ( checkFieldWithDuplicate( event, "createDate", null, parsed ) )
{
awsInstances.setCreateDate( getLongValue( getTrimmedValue( getDefaultValue( ( (ScalarEvent) parser.getEvent() ).getValue(), "0" ) ), "createDate", parser.peekEvent(), strict ) );
}
else if ( checkFieldWithDuplicate( event, "instances", null, parsed ) )
{
if ( !parser.getEvent().is( Event.ID.SequenceStart ) )
{
throw new ParserException( "Expected 'instances' data to start with a Sequence", event.getStartMark(), "", null );
}
java.util.List instances = awsInstances.getInstances();
if ( instances == null )
{
instances = new java.util.ArrayList/**/();
awsInstances.setInstances( instances );
}
while ( !parser.peekEvent().is( Event.ID.SequenceEnd ) )
{
instances.add( parseInstance( parser, strict ) );
}
parser.getEvent();
}
else
{
checkUnknownElement( event, parser, strict );
}
}
return awsInstances;
} //-- AwsInstances parseAwsInstances( Parser, boolean )
/**
* Method parseInstance.
*
* @param parser
* @param strict
* @throws IOException
* @return Instance
*/
private Instance parseInstance( Parser parser, boolean strict )
throws IOException
{
Event event = parser.getEvent();
if ( !event.is( Event.ID.MappingStart ) )
{
throw new ParserException( "Expected 'Instance' data to start with a Mapping", event.getStartMark(), "", null );
}
Instance instance = new Instance();
Set parsed = new HashSet();
while ( !( event = parser.getEvent() ).is( Event.ID.MappingEnd ) )
{
if ( checkFieldWithDuplicate( event, "model", null, parsed ) )
{
instance.setModel( getTrimmedValue( ( (ScalarEvent) parser.getEvent() ).getValue() ) );
}
else if ( checkFieldWithDuplicate( event, "vCPU", null, parsed ) )
{
instance.setVCPU( getIntegerValue( getTrimmedValue( getDefaultValue( ( (ScalarEvent) parser.getEvent() ).getValue(), "0" ) ), "vCPU", parser.peekEvent(), strict ) );
}
else if ( checkFieldWithDuplicate( event, "gpus", null, parsed ) )
{
instance.setGpus( getIntegerValue( getTrimmedValue( getDefaultValue( ( (ScalarEvent) parser.getEvent() ).getValue(), "0" ) ), "gpus", parser.peekEvent(), strict ) );
}
else if ( checkFieldWithDuplicate( event, "ram", null, parsed ) )
{
instance.setRam( getDoubleValue( getTrimmedValue( getDefaultValue( ( (ScalarEvent) parser.getEvent() ).getValue(), "0.0" ) ), "ram", parser.peekEvent(), strict ) );
}
else if ( checkFieldWithDuplicate( event, "storageType", null, parsed ) )
{
instance.setStorageType( getTrimmedValue( ( (ScalarEvent) parser.getEvent() ).getValue() ) );
}
else if ( checkFieldWithDuplicate( event, "ssdCount", null, parsed ) )
{
instance.setSsdCount( getIntegerValue( getTrimmedValue( getDefaultValue( ( (ScalarEvent) parser.getEvent() ).getValue(), "0" ) ), "ssdCount", parser.peekEvent(), strict ) );
}
else if ( checkFieldWithDuplicate( event, "ssdSize", null, parsed ) )
{
instance.setSsdSize( getIntegerValue( getTrimmedValue( getDefaultValue( ( (ScalarEvent) parser.getEvent() ).getValue(), "0" ) ), "ssdSize", parser.peekEvent(), strict ) );
}
else if ( checkFieldWithDuplicate( event, "networkingPerformace", null, parsed ) )
{
instance.setNetworkingPerformace( getTrimmedValue( getDefaultValue( ( (ScalarEvent) parser.getEvent() ).getValue(), "HIGH" ) ) );
}
else if ( checkFieldWithDuplicate( event, "physicalProcessor", null, parsed ) )
{
instance.setPhysicalProcessor( getTrimmedValue( getDefaultValue( ( (ScalarEvent) parser.getEvent() ).getValue(), "INTEL_XEON_E5_2670_V2" ) ) );
}
else if ( checkFieldWithDuplicate( event, "clockSpeed", null, parsed ) )
{
instance.setClockSpeed( getDoubleValue( getTrimmedValue( getDefaultValue( ( (ScalarEvent) parser.getEvent() ).getValue(), "2.5" ) ), "clockSpeed", parser.peekEvent(), strict ) );
}
else if ( checkFieldWithDuplicate( event, "intelAVX", null, parsed ) )
{
instance.setIntelAVX( getBooleanValue( getTrimmedValue( getDefaultValue( ( (ScalarEvent) parser.getEvent() ).getValue(), "true" ) ) ) );
}
else if ( checkFieldWithDuplicate( event, "intelAVX2", null, parsed ) )
{
instance.setIntelAVX2( getBooleanValue( getTrimmedValue( getDefaultValue( ( (ScalarEvent) parser.getEvent() ).getValue(), "false" ) ) ) );
}
else if ( checkFieldWithDuplicate( event, "intelTurbo", null, parsed ) )
{
instance.setIntelTurbo( getBooleanValue( getTrimmedValue( getDefaultValue( ( (ScalarEvent) parser.getEvent() ).getValue(), "true" ) ) ) );
}
else if ( checkFieldWithDuplicate( event, "ebsOptimized", null, parsed ) )
{
instance.setEbsOptimized( getBooleanValue( getTrimmedValue( getDefaultValue( ( (ScalarEvent) parser.getEvent() ).getValue(), "true" ) ) ) );
}
else if ( checkFieldWithDuplicate( event, "dedicatedEBSThroughput", null, parsed ) )
{
instance.setDedicatedEBSThroughput( getIntegerValue( getTrimmedValue( getDefaultValue( ( (ScalarEvent) parser.getEvent() ).getValue(), "-1" ) ), "dedicatedEBSThroughput", parser.peekEvent(), strict ) );
}
else if ( checkFieldWithDuplicate( event, "enhancedNetworking", null, parsed ) )
{
instance.setEnhancedNetworking( getBooleanValue( getTrimmedValue( getDefaultValue( ( (ScalarEvent) parser.getEvent() ).getValue(), "true" ) ) ) );
}
else if ( checkFieldWithDuplicate( event, "burstable", null, parsed ) )
{
instance.setBurstable( getBooleanValue( getTrimmedValue( getDefaultValue( ( (ScalarEvent) parser.getEvent() ).getValue(), "false" ) ) ) );
}
else if ( checkFieldWithDuplicate( event, "cpuCreditsPerHour", null, parsed ) )
{
instance.setCpuCreditsPerHour( getIntegerValue( getTrimmedValue( getDefaultValue( ( (ScalarEvent) parser.getEvent() ).getValue(), "0" ) ), "cpuCreditsPerHour", parser.peekEvent(), strict ) );
}
else if ( checkFieldWithDuplicate( event, "clusterNetworking", null, parsed ) )
{
instance.setClusterNetworking( getBooleanValue( getTrimmedValue( getDefaultValue( ( (ScalarEvent) parser.getEvent() ).getValue(), "true" ) ) ) );
}
else
{
checkUnknownElement( event, parser, strict );
}
}
return instance;
} //-- Instance parseInstance( Parser, boolean )
/**
* Method read.
*
* @param parser
* @param strict
* @throws IOException
* @return AwsInstances
*/
private AwsInstances read( Parser parser, boolean strict )
throws IOException
{
Event event;
if ( !( event = parser.getEvent() ).is( Event.ID.StreamStart ) )
{
throw new ParserException( "Expected Stream Start event", event.getStartMark(), "", null );
}
if ( !( event = parser.getEvent() ).is( Event.ID.DocumentStart ) )
{
throw new ParserException( "Expected Document Start event", event.getStartMark(), "", null );
}
AwsInstances awsInstances = parseAwsInstances( parser, strict );
if ( !( event = parser.getEvent() ).is( Event.ID.DocumentEnd ) )
{
throw new ParserException( "Expected Document End event", event.getStartMark(), "", null );
}
if ( !( event = parser.getEvent() ).is( Event.ID.StreamEnd ) )
{
throw new ParserException( "Expected Stream End event", event.getStartMark(), "", null );
}
return awsInstances;
} //-- AwsInstances read( Parser, boolean )
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy