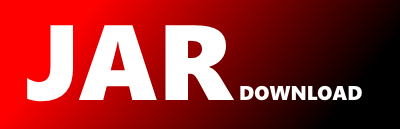
com.deliver8r.aws.io.snakeyaml.AWSResourcesSnakeYamlWriter Maven / Gradle / Ivy
// =================== DO NOT EDIT THIS FILE ====================
// Generated by Modello 1.8.3,
// any modifications will be overwritten.
// ==============================================================
package com.deliver8r.aws.io.snakeyaml;
//---------------------------------/
//- Imported classes and packages -/
//---------------------------------/
import com.deliver8r.aws.AwsInstances;
import com.deliver8r.aws.Instance;
import java.io.IOException;
import java.io.OutputStream;
import java.io.OutputStreamWriter;
import java.io.Writer;
import org.yaml.snakeyaml.DumperOptions;
import org.yaml.snakeyaml.DumperOptions.Version;
import org.yaml.snakeyaml.emitter.Emitable;
import org.yaml.snakeyaml.emitter.Emitter;
import org.yaml.snakeyaml.events.DocumentEndEvent;
import org.yaml.snakeyaml.events.DocumentStartEvent;
import org.yaml.snakeyaml.events.ImplicitTuple;
import org.yaml.snakeyaml.events.MappingEndEvent;
import org.yaml.snakeyaml.events.MappingStartEvent;
import org.yaml.snakeyaml.events.ScalarEvent;
import org.yaml.snakeyaml.events.SequenceEndEvent;
import org.yaml.snakeyaml.events.SequenceStartEvent;
import org.yaml.snakeyaml.events.StreamEndEvent;
import org.yaml.snakeyaml.events.StreamStartEvent;
/**
* Class AWSResourcesSnakeYamlWriter.
*
* @version $Revision$ $Date$
*/
public class AWSResourcesSnakeYamlWriter
{
//--------------------------/
//- Class/Member Variables -/
//--------------------------/
/**
* Field dumperOptions.
*/
private final DumperOptions dumperOptions = new DumperOptions();
//----------------/
//- Constructors -/
//----------------/
public AWSResourcesSnakeYamlWriter()
{
dumperOptions.setAllowUnicode( true );
dumperOptions.setPrettyFlow( true );
dumperOptions.setVersion( Version.V1_1 );
} //-- com.deliver8r.aws.io.snakeyaml.AWSResourcesSnakeYamlWriter()
//-----------/
//- Methods -/
//-----------/
/**
* Method write.
*
* @param writer
* @param awsInstances
* @throws IOException
*/
public void write( Writer writer, AwsInstances awsInstances )
throws IOException
{
Emitable generator = new Emitter( writer, dumperOptions );
generator.emit( new StreamStartEvent( null, null ) );
generator.emit( new DocumentStartEvent( null, null, dumperOptions.isExplicitStart(), dumperOptions.getVersion(), dumperOptions.getTags() ) );
writeAwsInstances( awsInstances, generator );
generator.emit( new DocumentEndEvent( null, null, dumperOptions.isExplicitEnd() ) );
generator.emit( new StreamEndEvent( null, null ) );
} //-- void write( Writer, AwsInstances )
/**
* Method write.
*
* @param stream
* @param awsInstances
* @throws IOException
*/
public void write( OutputStream stream, AwsInstances awsInstances )
throws IOException
{
write( new OutputStreamWriter( stream, awsInstances.getModelEncoding() ), awsInstances );
} //-- void write( OutputStream, AwsInstances )
/**
* Method writeAwsInstances.
*
* @param awsInstances
* @param generator
* @throws IOException
*/
private void writeAwsInstances( AwsInstances awsInstances, Emitable generator )
throws IOException
{
generator.emit( new MappingStartEvent( null, null, true, null, null, false ) );
if ( awsInstances.getCreateDate() != 0L )
{
generator.emit( new ScalarEvent( null, null, new ImplicitTuple( true, true ), "createDate", null, null, ' ' ) );
generator.emit( new ScalarEvent( null, null, new ImplicitTuple( true, true ), String.valueOf( awsInstances.getCreateDate() ), null, null, ' ' ) );
}
if ( ( awsInstances.getInstances() != null ) && ( awsInstances.getInstances().size() > 0 ) )
{
generator.emit( new ScalarEvent( null, null, new ImplicitTuple( true, true ), "instances", null, null, ' ' ) );
generator.emit( new SequenceStartEvent( null, null, true, null, null, false ) );
for ( Instance o : awsInstances.getInstances() )
{
writeInstance( o, generator );
}
generator.emit( new SequenceEndEvent( null, null ) );
}
generator.emit( new MappingEndEvent( null, null ) );
} //-- void writeAwsInstances( AwsInstances, Emitable )
/**
* Method writeInstance.
*
* @param instance
* @param generator
* @throws IOException
*/
private void writeInstance( Instance instance, Emitable generator )
throws IOException
{
generator.emit( new MappingStartEvent( null, null, true, null, null, false ) );
if ( instance.getModel() != null )
{
generator.emit( new ScalarEvent( null, null, new ImplicitTuple( true, true ), "model", null, null, ' ' ) );
generator.emit( new ScalarEvent( null, null, new ImplicitTuple( true, true ), instance.getModel(), null, null, ' ' ) );
}
if ( instance.getVCPU() != 0 )
{
generator.emit( new ScalarEvent( null, null, new ImplicitTuple( true, true ), "vCPU", null, null, ' ' ) );
generator.emit( new ScalarEvent( null, null, new ImplicitTuple( true, true ), String.valueOf( instance.getVCPU() ), null, null, ' ' ) );
}
if ( instance.getGpus() != 0 )
{
generator.emit( new ScalarEvent( null, null, new ImplicitTuple( true, true ), "gpus", null, null, ' ' ) );
generator.emit( new ScalarEvent( null, null, new ImplicitTuple( true, true ), String.valueOf( instance.getGpus() ), null, null, ' ' ) );
}
if ( instance.getRam() != 0.0 )
{
generator.emit( new ScalarEvent( null, null, new ImplicitTuple( true, true ), "ram", null, null, ' ' ) );
generator.emit( new ScalarEvent( null, null, new ImplicitTuple( true, true ), String.valueOf( instance.getRam() ), null, null, ' ' ) );
}
if ( instance.getStorageType() != null )
{
generator.emit( new ScalarEvent( null, null, new ImplicitTuple( true, true ), "storageType", null, null, ' ' ) );
generator.emit( new ScalarEvent( null, null, new ImplicitTuple( true, true ), instance.getStorageType(), null, null, ' ' ) );
}
if ( instance.getSsdCount() != 0 )
{
generator.emit( new ScalarEvent( null, null, new ImplicitTuple( true, true ), "ssdCount", null, null, ' ' ) );
generator.emit( new ScalarEvent( null, null, new ImplicitTuple( true, true ), String.valueOf( instance.getSsdCount() ), null, null, ' ' ) );
}
if ( instance.getSsdSize() != 0 )
{
generator.emit( new ScalarEvent( null, null, new ImplicitTuple( true, true ), "ssdSize", null, null, ' ' ) );
generator.emit( new ScalarEvent( null, null, new ImplicitTuple( true, true ), String.valueOf( instance.getSsdSize() ), null, null, ' ' ) );
}
if ( ( instance.getNetworkingPerformace() != null ) && !instance.getNetworkingPerformace().equals( "HIGH" ) )
{
generator.emit( new ScalarEvent( null, null, new ImplicitTuple( true, true ), "networkingPerformace", null, null, ' ' ) );
generator.emit( new ScalarEvent( null, null, new ImplicitTuple( true, true ), instance.getNetworkingPerformace(), null, null, ' ' ) );
}
if ( ( instance.getPhysicalProcessor() != null ) && !instance.getPhysicalProcessor().equals( "INTEL_XEON_E5_2670_V2" ) )
{
generator.emit( new ScalarEvent( null, null, new ImplicitTuple( true, true ), "physicalProcessor", null, null, ' ' ) );
generator.emit( new ScalarEvent( null, null, new ImplicitTuple( true, true ), instance.getPhysicalProcessor(), null, null, ' ' ) );
}
if ( instance.getClockSpeed() != 2.5 )
{
generator.emit( new ScalarEvent( null, null, new ImplicitTuple( true, true ), "clockSpeed", null, null, ' ' ) );
generator.emit( new ScalarEvent( null, null, new ImplicitTuple( true, true ), String.valueOf( instance.getClockSpeed() ), null, null, ' ' ) );
}
if ( instance.isIntelAVX() != true )
{
generator.emit( new ScalarEvent( null, null, new ImplicitTuple( true, true ), "intelAVX", null, null, ' ' ) );
generator.emit( new ScalarEvent( null, null, new ImplicitTuple( true, true ), String.valueOf( instance.isIntelAVX() ), null, null, ' ' ) );
}
if ( instance.isIntelAVX2() != false )
{
generator.emit( new ScalarEvent( null, null, new ImplicitTuple( true, true ), "intelAVX2", null, null, ' ' ) );
generator.emit( new ScalarEvent( null, null, new ImplicitTuple( true, true ), String.valueOf( instance.isIntelAVX2() ), null, null, ' ' ) );
}
if ( instance.isIntelTurbo() != true )
{
generator.emit( new ScalarEvent( null, null, new ImplicitTuple( true, true ), "intelTurbo", null, null, ' ' ) );
generator.emit( new ScalarEvent( null, null, new ImplicitTuple( true, true ), String.valueOf( instance.isIntelTurbo() ), null, null, ' ' ) );
}
if ( instance.isEbsOptimized() != true )
{
generator.emit( new ScalarEvent( null, null, new ImplicitTuple( true, true ), "ebsOptimized", null, null, ' ' ) );
generator.emit( new ScalarEvent( null, null, new ImplicitTuple( true, true ), String.valueOf( instance.isEbsOptimized() ), null, null, ' ' ) );
}
if ( instance.getDedicatedEBSThroughput() != -1 )
{
generator.emit( new ScalarEvent( null, null, new ImplicitTuple( true, true ), "dedicatedEBSThroughput", null, null, ' ' ) );
generator.emit( new ScalarEvent( null, null, new ImplicitTuple( true, true ), String.valueOf( instance.getDedicatedEBSThroughput() ), null, null, ' ' ) );
}
if ( instance.isEnhancedNetworking() != true )
{
generator.emit( new ScalarEvent( null, null, new ImplicitTuple( true, true ), "enhancedNetworking", null, null, ' ' ) );
generator.emit( new ScalarEvent( null, null, new ImplicitTuple( true, true ), String.valueOf( instance.isEnhancedNetworking() ), null, null, ' ' ) );
}
if ( instance.isBurstable() != false )
{
generator.emit( new ScalarEvent( null, null, new ImplicitTuple( true, true ), "burstable", null, null, ' ' ) );
generator.emit( new ScalarEvent( null, null, new ImplicitTuple( true, true ), String.valueOf( instance.isBurstable() ), null, null, ' ' ) );
}
if ( instance.getCpuCreditsPerHour() != 0 )
{
generator.emit( new ScalarEvent( null, null, new ImplicitTuple( true, true ), "cpuCreditsPerHour", null, null, ' ' ) );
generator.emit( new ScalarEvent( null, null, new ImplicitTuple( true, true ), String.valueOf( instance.getCpuCreditsPerHour() ), null, null, ' ' ) );
}
if ( instance.isClusterNetworking() != true )
{
generator.emit( new ScalarEvent( null, null, new ImplicitTuple( true, true ), "clusterNetworking", null, null, ' ' ) );
generator.emit( new ScalarEvent( null, null, new ImplicitTuple( true, true ), String.valueOf( instance.isClusterNetworking() ), null, null, ' ' ) );
}
generator.emit( new MappingEndEvent( null, null ) );
} //-- void writeInstance( Instance, Emitable )
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy