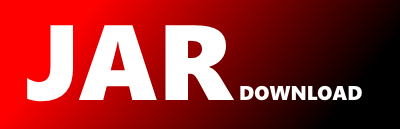
com.deliveredtechnologies.rulebook.lang.RuleBookRuleBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rulebook-core Show documentation
Show all versions of rulebook-core Show documentation
A simple and intuitive rules abstraction for Java
package com.deliveredtechnologies.rulebook.lang;
import com.deliveredtechnologies.rulebook.model.GoldenRule;
import com.deliveredtechnologies.rulebook.model.Rule;
import com.deliveredtechnologies.rulebook.model.RuleBook;
/**
* Chains building of a Rule onto a RuleBookBuilder.
* @param the type of the Result
*/
public class RuleBookRuleBuilder {
private RuleBook _ruleBook;
private Class extends Rule> _ruleClass = GoldenRule.class;
RuleBookRuleBuilder(RuleBook ruleBook) {
_ruleBook = ruleBook;
}
/**
* Specifies the Rule type.
* @param ruleType the type (class) of Rule to build
* @return RuleBookRuleBuilder using the Rule type specified
*/
public RuleBookRuleBuilder withRuleType(Class extends Rule> ruleType) {
_ruleClass = ruleType;
return this;
}
public RuleBookAuditableRuleBuilder withName(String ruleName) {
return new RuleBookAuditableRuleBuilder<>(_ruleBook, _ruleClass, ruleName);
}
/**
* Specifies the fact type
* @param factType the type of object that facts are restricted to in the Rule.
* @param the generic fact type
* @return RuleBookRuleWithFactTypeBuilder for building RuleBook Rules with a specific fact type
*/
@SuppressWarnings("unchecked")
public RuleBookRuleWithFactTypeBuilder withFactType(Class factType) {
Rule rule = (Rule)RuleBuilder.create(_ruleClass).withFactType(factType).build();
_ruleBook.addRule(rule);
return new RuleBookRuleWithFactTypeBuilder<>(rule);
}
/**
* Specifies no fact type.
* A generic Object type is used for the Rule's fact type.
* @return RuleBookRuleWithFactTypeBuilder for building RuleBook Rules with a specific fact type
*/
public RuleBookRuleWithFactTypeBuilder
© 2015 - 2025 Weber Informatics LLC | Privacy Policy