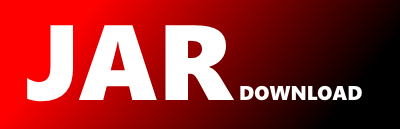
com.deliveredtechnologies.rulebook.lang.RuleBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rulebook-core Show documentation
Show all versions of rulebook-core Show documentation
A simple and intuitive rules abstraction for Java
package com.deliveredtechnologies.rulebook.lang;
import com.deliveredtechnologies.rulebook.Result;
import com.deliveredtechnologies.rulebook.Fact;
import com.deliveredtechnologies.rulebook.NameValueReferable;
import com.deliveredtechnologies.rulebook.NameValueReferableMap;
import com.deliveredtechnologies.rulebook.NameValueReferableTypeConvertibleMap;
import com.deliveredtechnologies.rulebook.model.AuditableRule;
import com.deliveredtechnologies.rulebook.model.GoldenRule;
import com.deliveredtechnologies.rulebook.model.Rule;
import com.deliveredtechnologies.rulebook.model.RuleChainActionType;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.lang.reflect.Constructor;
import java.lang.reflect.InvocationTargetException;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Predicate;
import static com.deliveredtechnologies.rulebook.model.RuleChainActionType.CONTINUE_ON_FAILURE;
import static com.deliveredtechnologies.rulebook.model.RuleChainActionType.STOP_ON_FAILURE;
/**
* The initial builder used to build a Rule.
* @param the type of facts used in the Rule
* @param the Result type used in the Rule
*/
public class RuleBuilder implements TerminatingRuleBuilder {
private static Logger LOGGER = LoggerFactory.getLogger(RuleBuilder.class);
private Class extends Rule> _ruleClass;
private Class _factType;
private Class _resultType;
private RuleChainActionType _actionType = CONTINUE_ON_FAILURE;
private Optional _name = Optional.empty();
/**
* Returns a new RuleBuilder for the specified Rule class.
* @param ruleClass the class of Rule to build
* @param actionType if STOP_ON_FAILURE, stops the rule chain if RuleState is BREAK only if the rule fails
* (for supported rule classes); default is CONTINUE_ON_FAILURE
* @return a new RuleBuilder
*/
public static RuleBuilder
© 2015 - 2025 Weber Informatics LLC | Privacy Policy