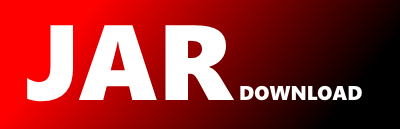
com.deliveredtechnologies.rulebook.lang.ThenRuleBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rulebook-core Show documentation
Show all versions of rulebook-core Show documentation
A simple and intuitive rules abstraction for Java
package com.deliveredtechnologies.rulebook.lang;
import com.deliveredtechnologies.rulebook.FactMap;
import com.deliveredtechnologies.rulebook.NameValueReferableTypeConvertibleMap;
import com.deliveredtechnologies.rulebook.Result;
import com.deliveredtechnologies.rulebook.RuleState;
import com.deliveredtechnologies.rulebook.model.Rule;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
/**
* Builds the portion of a Rule that is available in the languate on 'then'.
*/
public class ThenRuleBuilder implements TerminatingRuleBuilder {
Rule _rule;
ThenRuleBuilder(Rule rule, Consumer> action) {
_rule = rule;
_rule.addAction(action);
}
ThenRuleBuilder(Rule rule, BiConsumer, Result> action) {
_rule = rule;
_rule.addAction(action);
}
/**
* Adds a using constraint in the Rule that restricts the facts supplied to the subsequent 'then' action.
* @param factNames the fact names to be supplied to the subsequent 'then' action
* @return a builder the allows for the Rule to be built following the 'using' statement
*/
public UsingRuleBuilder using(String... factNames) {
return new UsingRuleBuilder(_rule, factNames);
}
/**
* Adds a then action into the Rule.
* @param action an action that the rule will execute based on the condition
* @return a builder that allows for the Rule to be built following the 'then' statement
*/
public ThenRuleBuilder then(Consumer> action) {
_rule.addAction(action);
return this;
}
/**
* Addds a then action into the Rule.
* @param action an action that the rule will execute based on the condition; accepts facts and the result
* @return a builder that allows for the Rule to be built following the 'then' statement
*/
public ThenRuleBuilder then(BiConsumer, Result> action) {
_rule.addAction(action);
return this;
}
/**
* Sends a signal that the rule chain should be broken.
* @return a TerminatingRuleBuilder that only allows for the Rule to be built following stop()
*/
public TerminatingRuleBuilder stop() {
_rule.setRuleState(RuleState.BREAK);
return this;
}
/**
* Builds the Rule.
* @return a Rule
*/
public Rule build() {
return _rule;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy