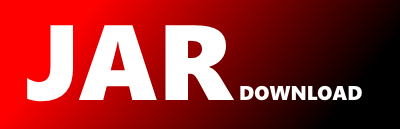
com.deliveredtechnologies.rulebook.runner.RuleAdapter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rulebook-core Show documentation
Show all versions of rulebook-core Show documentation
A simple and intuitive rules abstraction for Java
package com.deliveredtechnologies.rulebook.runner;
import com.deliveredtechnologies.rulebook.Decision;
import com.deliveredtechnologies.rulebook.Fact;
import com.deliveredtechnologies.rulebook.FactMap;
import com.deliveredtechnologies.rulebook.Result;
import com.deliveredtechnologies.rulebook.Rule;
import com.deliveredtechnologies.rulebook.RuleState;
import com.deliveredtechnologies.rulebook.StandardRule;
import com.deliveredtechnologies.rulebook.annotation.Given;
import com.deliveredtechnologies.rulebook.annotation.Then;
import com.deliveredtechnologies.rulebook.annotation.When;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.io.InvalidClassException;
import java.lang.reflect.Field;
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.Method;
import java.lang.reflect.ParameterizedType;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import java.util.Set;
import java.util.function.BiConsumer;
import java.util.function.BiFunction;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.function.Predicate;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import static com.deliveredtechnologies.rulebook.util.AnnotationUtils.getAnnotatedField;
import static com.deliveredtechnologies.rulebook.util.AnnotationUtils.getAnnotatedFields;
import static com.deliveredtechnologies.rulebook.util.AnnotationUtils.getAnnotatedMethods;
import static com.deliveredtechnologies.rulebook.util.AnnotationUtils.getAnnotation;
/**
* RuleAdapter accepts a POJO annotated Rule class and adapts it to an actual Rule class.
*/
@Deprecated
public class RuleAdapter implements Decision {
private static Logger LOGGER = LoggerFactory.getLogger(RuleAdapter.class);
private Object _rulePojo;
private Rule _rule;
private Result _result = new Result();
/**
* RuleAdapter accepts a {@link com.deliveredtechnologies.rulebook.annotation.Rule} annotated POJO
* and adapts it to a {@link Rule} or {@link com.deliveredtechnologies.rulebook.Decision}.
* @param rulePojo an annotated POJO to be adapted to a rule
* @param rule the {@link Rule} object delegated to for the adaptation
* @throws InvalidClassException if the POJO does not have the @Rule annotation
*/
@SuppressWarnings("unchecked")
public RuleAdapter(Object rulePojo, Rule rule) throws InvalidClassException {
if (getAnnotation(com.deliveredtechnologies.rulebook.annotation.Rule.class, rulePojo.getClass()) == null) {
throw new InvalidClassException(rulePojo.getClass() + " is not a Rule; missing @Rule annotation");
}
_rule = rule;
_rulePojo = rulePojo;
}
/**
* RuleAdapter accepts a {@link com.deliveredtechnologies.rulebook.annotation.Rule} annotated POJO
* and adapts it to a {@link Rule} or {@link com.deliveredtechnologies.rulebook.Decision}.
* This convenience constructor supplies a generic {@link StandardRule} to RuleAdapter(Object, Rule).
* @param rulePojo an annotated POJO to be adapted to a rule
* @throws InvalidClassException if the POJO does not have the @Rule annotation
*/
@SuppressWarnings("unchecked")
public RuleAdapter(Object rulePojo) throws InvalidClassException {
this(rulePojo, new StandardRule(Object.class));
}
@Override
@SuppressWarnings("unchecked")
public Decision given(Fact... facts) {
_rule.given(facts);
mapGivenFactsToProperties();
return this;
}
@Override
@SuppressWarnings("unchecked")
public Decision given(List list) {
_rule.given(list);
mapGivenFactsToProperties();
return this;
}
@Override
@SuppressWarnings("unchecked")
public Decision given(FactMap facts) {
_rule.given(facts);
mapGivenFactsToProperties();
return this;
}
@Override
@SuppressWarnings("unchecked")
public Decision given(String name, Object value) {
_rule.given(name, value);
mapGivenFactsToProperties();
return this;
}
@Override
public Decision givenUnTyped(FactMap facts) {
_rule.givenUnTyped(facts);
mapGivenFactsToProperties();
return this;
}
@Override
public Predicate getWhen() {
//Use what was set by then() first, if it's there
if (_rule.getWhen() != null) {
return _rule.getWhen();
}
//If nothing was explicitly set, then convert the method in the class
return Arrays.stream(_rulePojo.getClass().getMethods())
.filter(method -> method.getReturnType() == boolean.class || method.getReturnType() == Boolean.class)
.filter(method -> Arrays.stream(method.getDeclaredAnnotations()).anyMatch(When.class::isInstance))
.findFirst()
.map(method -> object -> {
try {
return (Boolean) method.invoke(_rulePojo);
} catch (InvocationTargetException | IllegalAccessException ex) {
LOGGER.error(
"Unable to validate condition due to an exception. Condition will be interpreted as false", ex);
return false;
}
})
//If the condition still can't be determined, then just had back one that returns false
.orElse(o -> false);
}
@Override
public List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy