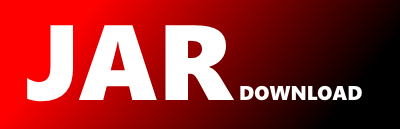
com.deliveredtechnologies.terraform.helpers.Http.groovy Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of tf-test-groovy Show documentation
Show all versions of tf-test-groovy Show documentation
Terraform Build Tools: The parent POM project for Java and Terraform Tools
package com.deliveredtechnologies.terraform.helpers
import net.jodah.failsafe.Failsafe
import net.jodah.failsafe.RetryPolicy
import net.jodah.failsafe.function.CheckedSupplier
import org.slf4j.Logger
import org.slf4j.LoggerFactory
import java.time.Duration
import java.util.function.Consumer
class Http {
private final Logger log = LoggerFactory.getLogger(getClass())
private Http(){
throw new IllegalStateException("Static utility class")
}
static Map httpGetWithCustomValidation(String url, Consumer statusCode, Consumer response, Proxy proxy = Proxy.NO_PROXY) {
def connection = new URL(url).openConnection(proxy)
def body = connection.content.text
int responseCode = connection.responseCode
statusCode.accept(responseCode)
response.accept(body)
[statusCode: statusCode, body: body]
}
static Map httpGetRetryWithCustomValidation(String url, Duration delay, int maxRetries, Consumer statusCode, Consumer response, Proxy proxy = Proxy.NO_PROXY) {
RetryPolicy
© 2015 - 2025 Weber Informatics LLC | Privacy Policy