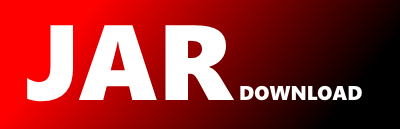
com.dell.cpsd.hal.data.provider.api.ElementDefinition Maven / Gradle / Ivy
package com.dell.cpsd.hal.data.provider.api;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import org.apache.commons.lang.builder.EqualsBuilder;
import org.apache.commons.lang.builder.HashCodeBuilder;
import org.apache.commons.lang.builder.ToStringBuilder;
/**
* ElementDefinition
*
*
*
*/
@JsonInclude(JsonInclude.Include.NON_NULL)
@JsonPropertyOrder({
"vendor",
"productFamily",
"product",
"modelFamily",
"model",
"code"
})
public class ElementDefinition {
@JsonProperty("vendor")
private String vendor;
@JsonProperty("productFamily")
private String productFamily;
@JsonProperty("product")
private String product;
@JsonProperty("modelFamily")
private String modelFamily;
@JsonProperty("model")
private String model;
@JsonProperty("code")
private String code;
/**
* No args constructor for use in serialization
*
*/
public ElementDefinition() {
}
/**
*
* @param productFamily
* @param product
* @param code
* @param modelFamily
* @param vendor
* @param model
*/
public ElementDefinition(String vendor, String productFamily, String product, String modelFamily, String model, String code) {
super();
this.vendor = vendor;
this.productFamily = productFamily;
this.product = product;
this.modelFamily = modelFamily;
this.model = model;
this.code = code;
}
/**
*
* @return
* The vendor
*/
@JsonProperty("vendor")
public String getVendor() {
return vendor;
}
/**
*
* @param vendor
* The vendor
*/
@JsonProperty("vendor")
public void setVendor(String vendor) {
this.vendor = vendor;
}
/**
*
* @return
* The productFamily
*/
@JsonProperty("productFamily")
public String getProductFamily() {
return productFamily;
}
/**
*
* @param productFamily
* The productFamily
*/
@JsonProperty("productFamily")
public void setProductFamily(String productFamily) {
this.productFamily = productFamily;
}
/**
*
* @return
* The product
*/
@JsonProperty("product")
public String getProduct() {
return product;
}
/**
*
* @param product
* The product
*/
@JsonProperty("product")
public void setProduct(String product) {
this.product = product;
}
/**
*
* @return
* The modelFamily
*/
@JsonProperty("modelFamily")
public String getModelFamily() {
return modelFamily;
}
/**
*
* @param modelFamily
* The modelFamily
*/
@JsonProperty("modelFamily")
public void setModelFamily(String modelFamily) {
this.modelFamily = modelFamily;
}
/**
*
* @return
* The model
*/
@JsonProperty("model")
public String getModel() {
return model;
}
/**
*
* @param model
* The model
*/
@JsonProperty("model")
public void setModel(String model) {
this.model = model;
}
/**
*
* @return
* The code
*/
@JsonProperty("code")
public String getCode() {
return code;
}
/**
*
* @param code
* The code
*/
@JsonProperty("code")
public void setCode(String code) {
this.code = code;
}
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this);
}
@Override
public int hashCode() {
return new HashCodeBuilder().append(vendor).append(productFamily).append(product).append(modelFamily).append(model).append(code).toHashCode();
}
@Override
public boolean equals(Object other) {
if (other == this) {
return true;
}
if ((other instanceof ElementDefinition) == false) {
return false;
}
ElementDefinition rhs = ((ElementDefinition) other);
return new EqualsBuilder().append(vendor, rhs.vendor).append(productFamily, rhs.productFamily).append(product, rhs.product).append(modelFamily, rhs.modelFamily).append(model, rhs.model).append(code, rhs.code).isEquals();
}
}