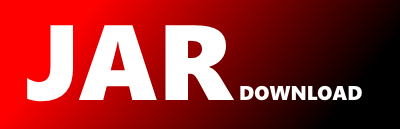
com.dell.cpsd.hal.data.provider.api.ElementEndpointValidationResult Maven / Gradle / Ivy
package com.dell.cpsd.hal.data.provider.api;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyDescription;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.annotation.JsonValue;
import org.apache.commons.lang.builder.EqualsBuilder;
import org.apache.commons.lang.builder.HashCodeBuilder;
import org.apache.commons.lang.builder.ToStringBuilder;
/**
* ElementEndpointValidationResult
*
*
*
*/
@JsonInclude(JsonInclude.Include.NON_NULL)
@JsonPropertyOrder({
"uuid",
"protocol",
"address",
"type",
"status",
"failureReasons"
})
public class ElementEndpointValidationResult {
/**
* Endpoint's unique identifier.
*
*/
@JsonProperty("uuid")
@JsonPropertyDescription("")
private String uuid;
/**
* Expected all-uppercase: SSH, CIM, XMLAPI...
*
*/
@JsonProperty("protocol")
@JsonPropertyDescription("")
private String protocol;
/**
* Address shouldn't have protocol prefix, as there is a separate field for that. IPv4 and IPv6 accepted.
*
*/
@JsonProperty("address")
@JsonPropertyDescription("")
private String address;
/**
* Optional field for different end point types like: ECOM Server, SPA, SPB.
*
*/
@JsonProperty("type")
@JsonPropertyDescription("")
private String type;
/**
*
* (Required)
*
*/
@JsonProperty("status")
private ElementEndpointValidationResult.Status status;
@JsonProperty("failureReasons")
private List failureReasons = new ArrayList();
/**
* No args constructor for use in serialization
*
*/
public ElementEndpointValidationResult() {
}
/**
*
* @param protocol
* @param address
* @param failureReasons
* @param type
* @param uuid
* @param status
*/
public ElementEndpointValidationResult(String uuid, String protocol, String address, String type, ElementEndpointValidationResult.Status status, List failureReasons) {
super();
this.uuid = uuid;
this.protocol = protocol;
this.address = address;
this.type = type;
this.status = status;
this.failureReasons = failureReasons;
}
/**
* Endpoint's unique identifier.
*
* @return
* The uuid
*/
@JsonProperty("uuid")
public String getUuid() {
return uuid;
}
/**
* Endpoint's unique identifier.
*
* @param uuid
* The uuid
*/
@JsonProperty("uuid")
public void setUuid(String uuid) {
this.uuid = uuid;
}
/**
* Expected all-uppercase: SSH, CIM, XMLAPI...
*
* @return
* The protocol
*/
@JsonProperty("protocol")
public String getProtocol() {
return protocol;
}
/**
* Expected all-uppercase: SSH, CIM, XMLAPI...
*
* @param protocol
* The protocol
*/
@JsonProperty("protocol")
public void setProtocol(String protocol) {
this.protocol = protocol;
}
/**
* Address shouldn't have protocol prefix, as there is a separate field for that. IPv4 and IPv6 accepted.
*
* @return
* The address
*/
@JsonProperty("address")
public String getAddress() {
return address;
}
/**
* Address shouldn't have protocol prefix, as there is a separate field for that. IPv4 and IPv6 accepted.
*
* @param address
* The address
*/
@JsonProperty("address")
public void setAddress(String address) {
this.address = address;
}
/**
* Optional field for different end point types like: ECOM Server, SPA, SPB.
*
* @return
* The type
*/
@JsonProperty("type")
public String getType() {
return type;
}
/**
* Optional field for different end point types like: ECOM Server, SPA, SPB.
*
* @param type
* The type
*/
@JsonProperty("type")
public void setType(String type) {
this.type = type;
}
/**
*
* (Required)
*
* @return
* The status
*/
@JsonProperty("status")
public ElementEndpointValidationResult.Status getStatus() {
return status;
}
/**
*
* (Required)
*
* @param status
* The status
*/
@JsonProperty("status")
public void setStatus(ElementEndpointValidationResult.Status status) {
this.status = status;
}
/**
*
* @return
* The failureReasons
*/
@JsonProperty("failureReasons")
public List getFailureReasons() {
return failureReasons;
}
/**
*
* @param failureReasons
* The failureReasons
*/
@JsonProperty("failureReasons")
public void setFailureReasons(List failureReasons) {
this.failureReasons = failureReasons;
}
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this);
}
@Override
public int hashCode() {
return new HashCodeBuilder().append(uuid).append(protocol).append(address).append(type).append(status).append(failureReasons).toHashCode();
}
@Override
public boolean equals(Object other) {
if (other == this) {
return true;
}
if ((other instanceof ElementEndpointValidationResult) == false) {
return false;
}
ElementEndpointValidationResult rhs = ((ElementEndpointValidationResult) other);
return new EqualsBuilder().append(uuid, rhs.uuid).append(protocol, rhs.protocol).append(address, rhs.address).append(type, rhs.type).append(status, rhs.status).append(failureReasons, rhs.failureReasons).isEquals();
}
public enum Status {
SUCCESS("success"),
FAILURE("failure"),
NOT_APPLICABLE("notApplicable");
private final String value;
private final static Map CONSTANTS = new HashMap();
static {
for (ElementEndpointValidationResult.Status c: values()) {
CONSTANTS.put(c.value, c);
}
}
private Status(String value) {
this.value = value;
}
@Override
public String toString() {
return this.value;
}
@JsonValue
public String value() {
return this.value;
}
@JsonCreator
public static ElementEndpointValidationResult.Status fromValue(String value) {
ElementEndpointValidationResult.Status constant = CONSTANTS.get(value);
if (constant == null) {
throw new IllegalArgumentException(value);
} else {
return constant;
}
}
}
}