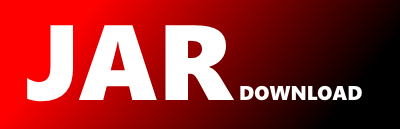
com.dell.cpsd.hal.data.provider.api.TimeoutReason Maven / Gradle / Ivy
package com.dell.cpsd.hal.data.provider.api;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyDescription;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import org.apache.commons.lang.builder.EqualsBuilder;
import org.apache.commons.lang.builder.HashCodeBuilder;
import org.apache.commons.lang.builder.ToStringBuilder;
@JsonInclude(JsonInclude.Include.NON_NULL)
@JsonPropertyOrder({
"code",
"message"
})
public class TimeoutReason {
/**
* The code provided by the service for the timeout.
* (Required)
*
*/
@JsonProperty("code")
@JsonPropertyDescription("")
private String code;
/**
* The message provided by the service for the timeout.
* (Required)
*
*/
@JsonProperty("message")
@JsonPropertyDescription("")
private String message;
/**
* No args constructor for use in serialization
*
*/
public TimeoutReason() {
}
/**
*
* @param code
* @param message
*/
public TimeoutReason(String code, String message) {
super();
this.code = code;
this.message = message;
}
/**
* The code provided by the service for the timeout.
* (Required)
*
* @return
* The code
*/
@JsonProperty("code")
public String getCode() {
return code;
}
/**
* The code provided by the service for the timeout.
* (Required)
*
* @param code
* The code
*/
@JsonProperty("code")
public void setCode(String code) {
this.code = code;
}
/**
* The message provided by the service for the timeout.
* (Required)
*
* @return
* The message
*/
@JsonProperty("message")
public String getMessage() {
return message;
}
/**
* The message provided by the service for the timeout.
* (Required)
*
* @param message
* The message
*/
@JsonProperty("message")
public void setMessage(String message) {
this.message = message;
}
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this);
}
@Override
public int hashCode() {
return new HashCodeBuilder().append(code).append(message).toHashCode();
}
@Override
public boolean equals(Object other) {
if (other == this) {
return true;
}
if ((other instanceof TimeoutReason) == false) {
return false;
}
TimeoutReason rhs = ((TimeoutReason) other);
return new EqualsBuilder().append(code, rhs.code).append(message, rhs.message).isEquals();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy