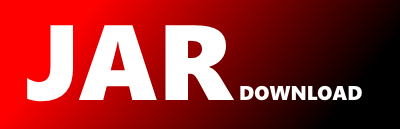
com.dell.cpsd.hdp.capability.registry.api.LookupCapabilityRegistryResultMessage Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of hdp-capability-registry-client Show documentation
Show all versions of hdp-capability-registry-client Show documentation
This repository contains the source code for the capability registry API.
This API exposes the interface through which a consumer or provider interacts with the capability registry.
package com.dell.cpsd.hdp.capability.registry.api;
import java.util.ArrayList;
import java.util.List;
import com.dell.cpsd.common.rabbitmq.annotation.Message;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import org.apache.commons.lang.builder.EqualsBuilder;
import org.apache.commons.lang.builder.HashCodeBuilder;
import org.apache.commons.lang.builder.ToStringBuilder;
/**
* CapabilityRegistryLookupResult
*
* Message with the results of the capability registry lookup.
*
* Copyright © 2017 Dell Inc. or its subsidiaries. All Rights Reserved.
* Dell EMC Confidential/Proprietary Information
*
*
*/
@JsonInclude(JsonInclude.Include.NON_NULL)
@Message(value = "com.dell.cpsd.hdp.capability.registry.lookup.result", version = "1.0")
@JsonPropertyOrder({
"hostname",
"queryResults"
})
public class LookupCapabilityRegistryResultMessage {
@JsonProperty("hostname")
private String hostname;
@JsonProperty("queryResults")
private List queryResults = new ArrayList();
/**
* No args constructor for use in serialization
*
*/
public LookupCapabilityRegistryResultMessage() {
}
/**
*
* @param hostname
* @param queryResults
*/
public LookupCapabilityRegistryResultMessage(String hostname, List queryResults) {
super();
this.hostname = hostname;
this.queryResults = queryResults;
}
/**
*
* @return
* The hostname
*/
@JsonProperty("hostname")
public String getHostname() {
return hostname;
}
/**
*
* @param hostname
* The hostname
*/
@JsonProperty("hostname")
public void setHostname(String hostname) {
this.hostname = hostname;
}
/**
*
* @return
* The queryResults
*/
@JsonProperty("queryResults")
public List getQueryResults() {
return queryResults;
}
/**
*
* @param queryResults
* The queryResults
*/
@JsonProperty("queryResults")
public void setQueryResults(List queryResults) {
this.queryResults = queryResults;
}
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this);
}
@Override
public int hashCode() {
return new HashCodeBuilder().append(hostname).append(queryResults).toHashCode();
}
@Override
public boolean equals(Object other) {
if (other == this) {
return true;
}
if ((other instanceof LookupCapabilityRegistryResultMessage) == false) {
return false;
}
LookupCapabilityRegistryResultMessage rhs = ((LookupCapabilityRegistryResultMessage) other);
return new EqualsBuilder().append(hostname, rhs.hostname).append(queryResults, rhs.queryResults).isEquals();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy