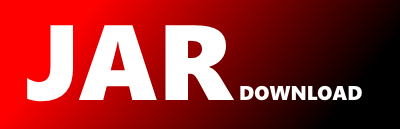
com.dell.cpsd.hdp.capability.registry.client.binder.CapabilityMatcher Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of hdp-capability-registry-client Show documentation
Show all versions of hdp-capability-registry-client Show documentation
This repository contains the source code for the capability registry API.
This API exposes the interface through which a consumer or provider interacts with the capability registry.
/**
* Copyright © 2017 Dell Inc. or its subsidiaries. All Rights Reserved.
* Dell EMC Confidential/Proprietary Information
*/
package com.dell.cpsd.hdp.capability.registry.client.binder;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.function.Predicate;
import com.dell.cpsd.hdp.capability.registry.api.Capability;
import com.dell.cpsd.hdp.capability.registry.api.CapabilityProvider;
import com.dell.cpsd.hdp.capability.registry.api.ProviderEndpoint;
import com.dell.cpsd.hdp.capability.registry.client.helper.AmqpProviderEndpointHelper;
/**
*
* Copyright © 2017 Dell Inc. or its subsidiaries. All Rights Reserved.
* Dell EMC Confidential/Proprietary Information
*
*
* @since 1.0
*/
public class CapabilityMatcher
{
/**
* The ability to match on all or any capability.
*/
public enum CardinalReduction
{
ALL,
ANY;
}
private String profile = null;
private String requestMessageType = null;
private String responseMessageType = null;
private String eventMessageType = null;
private CardinalReduction cardinalReduction = CardinalReduction.ANY;
/**
* Builder method for profile
*
* @param profile
* The profile name
* @return The capability matcher with the profile name
*/
public CapabilityMatcher withProfile(String profile)
{
this.profile = profile;
return this;
}
/**
* Builder method for cardinal reduction
*
* @param cardinalReduction
* The cardinal reduction
* @return The capability matcher with the cardinal reduction
*/
public CapabilityMatcher withCardinalReduction(CardinalReduction cardinalReduction)
{
this.cardinalReduction = cardinalReduction;
return this;
}
/**
* Builder method for request type
*
* @param messageType
* The message type
* @return The capability matcher with the request message type
*/
public CapabilityMatcher withRequestMessageType(String messageType)
{
this.requestMessageType = messageType;
return this;
}
/**
* Builder method for response type
*
* @param messageType
* The message type
* @return The capability matcher with the response message type
*/
public CapabilityMatcher withResponseMessageType(String messageType)
{
this.responseMessageType = messageType;
return this;
}
/**
* Builder method for event type
*
* @param messageType
* The message type
* @return The capability matcher with the event message type
*/
public CapabilityMatcher withEventMessageType(String messageType)
{
this.eventMessageType = messageType;
return this;
}
/**
* Match capabilities from the providers
*
* @param providers
* The capability provider list
* @return The capability data list matched
*/
public List match(List providers)
{
ProviderEndpointFilter providerEndpointFilter = new ProviderEndpointFilter().withRequestMessageType(requestMessageType)
.withResponseMessageType(responseMessageType).withEventMessageType(eventMessageType);
CapabilityFilter capabilityFilter = new CapabilityFilter().withProfile(profile).withProviderEndpointFiler(providerEndpointFilter);
final List capabilityDatas = new ArrayList<>();
if (providers != null)
{
for (CapabilityProvider provider : providers)
{
if (provider.getCapabilities() != null)
{
List capabilities = provider.getCapabilities();
if (capabilities != null)
{
for (Capability capability : capabilities)
{
if (capabilityFilter.test(capability))
{
capabilityDatas.add(new CapabilityData(provider, capability));
}
}
}
}
}
}
switch (cardinalReduction)
{
case ALL:
{
return capabilityDatas;
}
case ANY:
{
if (capabilityDatas.size() > 0)
{
return Arrays.asList(capabilityDatas.iterator().next());
}
}
}
return Collections.emptyList();
}
private class CapabilityFilter implements Predicate
{
private String profile;
private ProviderEndpointFilter providerEndpointFilter;
public CapabilityFilter withProfile(String profile)
{
this.profile = profile;
return this;
}
public CapabilityFilter withProviderEndpointFiler(ProviderEndpointFilter providerEndpointFilter)
{
this.providerEndpointFilter = providerEndpointFilter;
return this;
}
@Override
public boolean test(Capability capability)
{
boolean matched = true;
if (capability.getProviderEndpoint() == null)
{
matched = false;
}
if (matched)
{
if (providerEndpointFilter != null)
{
matched = providerEndpointFilter.test(capability.getProviderEndpoint());
}
}
if (matched)
{
if (profile != null)
{
matched = profile.equalsIgnoreCase(capability.getProfile());
}
}
return matched;
}
}
private class ProviderEndpointFilter implements Predicate
{
private String requestMessageType = null;
private String responseMessageType = null;
private String eventMessageType = null;
/**
* Builder method for request type
*
* @param messageType
* The message type
* @return The provider endpoint filter with the request message type
*/
public ProviderEndpointFilter withRequestMessageType(String messageType)
{
this.requestMessageType = messageType;
return this;
}
/**
* Builder method for response type
*
* @param messageType
* The message type
* @return The provider endpoint filter with the response message type
*/
public ProviderEndpointFilter withResponseMessageType(String messageType)
{
this.responseMessageType = messageType;
return this;
}
/**
* Builder method for event type
*
* @param messageType
* The message type
* @return The provider endpoint filter with the event message type
*/
public ProviderEndpointFilter withEventMessageType(String messageType)
{
this.eventMessageType = messageType;
return this;
}
@Override
public boolean test(ProviderEndpoint endpoint)
{
AmqpProviderEndpointHelper endpointHelper = new AmqpProviderEndpointHelper(endpoint);
boolean matched = true;
if (requestMessageType != null)
{
matched = requestMessageType.equals(endpointHelper.getRequestMessageType());
}
if (matched)
{
if (responseMessageType != null)
{
matched = responseMessageType.equals(endpointHelper.getResponseMessageType());
}
}
if (matched)
{
if (eventMessageType != null)
{
matched = eventMessageType.equals(endpointHelper.getEventMessageType());
}
}
return matched;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy