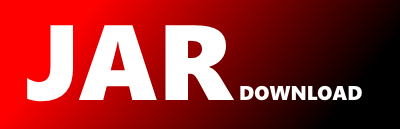
com.dell.cpsd.hdp.capability.registry.client.builder.AmqpProviderEndpointBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of hdp-capability-registry-client Show documentation
Show all versions of hdp-capability-registry-client Show documentation
This repository contains the source code for the capability registry API.
This API exposes the interface through which a consumer or provider interacts with the capability registry.
/**
* Copyright © 2017 Dell Inc. or its subsidiaries. All Rights Reserved.
* Dell EMC Confidential/Proprietary Information
*/
package com.dell.cpsd.hdp.capability.registry.client.builder;
import java.util.ArrayList;
import java.util.List;
import com.dell.cpsd.hdp.capability.registry.api.EndpointProperty;
import com.dell.cpsd.hdp.capability.registry.api.ProviderEndpoint;
/**
* This builder creates an AMQP ProviderEndpoint
.
*
* Copyright © 2017 Dell Inc. or its subsidiaries. All Rights Reserved.
* Dell EMC Confidential/Proprietary Information
*
*
* @since 1.0
*/
public class AmqpProviderEndpointBuilder
{
/*
* The constants for this builder.
*/
public static final String AMQP_PROTOCOL = "AMQP";
public static final String REQUEST_EXCHANGE = "request-exchange";
public static final String REQUEST_EXCHANGE_TYPE = "request-exchange-type";
public static final String REQUEST_ROUTING_KEY = "request-routing-key";
public static final String REQUEST_MESSAGE_TYPE = "request-message-type";
public static final String REQUEST_MESSAGE_VERSION = "request-message-version";
public static final String REQUEST_ACK_ROUTING_KEY = "request-ack-routing-key";
public static final String REQUEST_ACK_MESSAGE_TYPE = "request-ack-message-type";
public static final String REQUEST_ACK_MESSAGE_VERSION = "request-ack-message-version";
public static final String RESPONSE_EXCHANGE = "response-exchange";
public static final String RESPONSE_EXCHANGE_TYPE = "response-exchange-type";
public static final String RESPONSE_ROUTING_KEY = "response-routing-key";
public static final String RESPONSE_MESSAGE_TYPE = "response-message-type";
public static final String RESPONSE_MESSAGE_VERSION = "response-message-version";
public static final String EVENT_EXCHANGE = "event-exchange";
public static final String EVENT_EXCHANGE_TYPE = "event-exchange-type";
public static final String EVENT_ROUTING_KEY = "event-routing-key";
public static final String EVENT_MESSAGE_TYPE = "event-message-type";
public static final String EVENT_MESSAGE_VERSION = "event-message-version";
/*
* The provider endpoint type.
*/
private String type = null;
/*
* The name of the request exchange.
*/
private String requestExchange = null;
/*
* The type of the request exchange.
*/
private String requestExchangeType = null;
/*
* The request routing key
*/
private String requestRoutingKey = null;
/*
* The request message type.
*/
private String requestMessageType = null;
/*
* The request message version.
*/
private String requestMessageVersion = null;
/*
* The request ack routing key
*/
private String requestAckRoutingKey = null;
/*
* The request ack message type.
*/
private String requestAckMessageType = null;
/*
* The request ack message version.
*/
private String requestAckMessageVersion = null;
/*
* The name of the response exchange.
*/
private String responseExchange = null;
/*
* The type of the response exchange.
*/
private String responseExchangeType = null;
/*
* The response routing key.
*/
private String responseRoutingKey = null;
/*
* The response message type.
*/
private String responseMessageType = null;
/*
* The response message version.
*/
private String responseMessageVersion = null;
/*
* The name of the event exchange.
*/
private String eventExchange = null;
/*
* The type of the event exchange.
*/
private String eventExchangeType = null;
/*
* The event routing key.
*/
private String eventRoutingKey = null;
/*
* The event message type.
*/
private String eventMessageType = null;
/*
* The event message version.
*/
private String eventMessageVersion = null;
/**
* AmqpProviderEndpointBuilder constructor.
*
* @param type
* The provider endpoint type.
* @since 1.0
*/
public AmqpProviderEndpointBuilder(final String type)
{
super();
this.type = type;
}
/**
* This adds the name of the request exchange.
*
* @param requestExchange
* The name of the request exchange.
*
* @return The AmqpProviderEndpointBuilder
builder.
*
* @since 1.0
*/
public AmqpProviderEndpointBuilder requestExchange(final String requestExchange)
{
this.requestExchange = requestExchange;
return this;
}
/**
* This adds the type of the request exchange.
*
* @param requestExchangeType
* The type of the request exchange.
*
* @return The AmqpProviderEndpointBuilder
builder.
*
* @since 1.0
*/
public AmqpProviderEndpointBuilder requestExchangeType(final String requestExchangeType)
{
this.requestExchangeType = requestExchangeType;
return this;
}
/**
* This adds the request routing key.
*
* @param requestRoutingKey
* The request routing key.
*
* @return The AmqpProviderEndpointBuilder
builder.
*
* @since 1.0
*/
public AmqpProviderEndpointBuilder requestRoutingKey(final String requestRoutingKey)
{
this.requestRoutingKey = requestRoutingKey;
return this;
}
/**
* This adds the request message type.
*
* @param requestMessageType
* The request message type.
*
* @return The AmqpProviderEndpointBuilder
builder.
*
* @since 1.0
*/
public AmqpProviderEndpointBuilder requestMessageType(final String requestMessageType)
{
this.requestMessageType = requestMessageType;
return this;
}
/**
* This adds the request message version.
*
* @param requestMessageVersion
* The request message version.
*
* @return The AmqpProviderEndpointBuilder
builder.
*
* @since 1.0
*/
public AmqpProviderEndpointBuilder requestMessageVersion(final String requestMessageVersion)
{
this.requestMessageVersion = requestMessageVersion;
return this;
}
/**
* This adds the request ack routing key.
*
* @param requestAckRoutingKey
* The request ack routing key.
*
* @return The AmqpProviderEndpointBuilder
builder.
*
* @since 1.0
*/
public AmqpProviderEndpointBuilder requestAckRoutingKey(final String requestAckRoutingKey)
{
this.requestAckRoutingKey = requestAckRoutingKey;
return this;
}
/**
* This adds the request ack message type.
*
* @param requestAckMessageType
* The request ack message type.
*
* @return The AmqpProviderEndpointBuilder
builder.
*
* @since 1.0
*/
public AmqpProviderEndpointBuilder requestAckMessageType(final String requestAckMessageType)
{
this.requestAckMessageType = requestAckMessageType;
return this;
}
/**
* This adds the request ack message version.
*
* @param requestAckMessageVersion
* The request message version.
*
* @return The AmqpProviderEndpointBuilder
builder.
*
* @since 1.0
*/
public AmqpProviderEndpointBuilder requestAckMessageVersion(final String requestAckMessageVersion)
{
this.requestAckMessageVersion = requestAckMessageVersion;
return this;
}
/**
* This adds the name of the response exchange.
*
* @param responseExchange
* The name of the response exchange.
*
* @return The AmqpProviderEndpointBuilder
builder.
*
* @since 1.0
*/
public AmqpProviderEndpointBuilder responseExchange(final String responseExchange)
{
this.responseExchange = responseExchange;
return this;
}
/**
* This adds the type of the response exchange.
*
* @param responseExchangeType
* The type of the response exchange.
*
* @return The AmqpProviderEndpointBuilder
builder.
*
* @since 1.0
*/
public AmqpProviderEndpointBuilder responseExchangeType(final String responseExchangeType)
{
this.responseExchangeType = responseExchangeType;
return this;
}
/**
* This adds the response routing key.
*
* @param responseRoutingKey
* The response routing key.
*
* @return The AmqpProviderEndpointBuilder
builder.
*
* @since 1.0
*/
public AmqpProviderEndpointBuilder responseRoutingKey(final String responseRoutingKey)
{
this.responseRoutingKey = responseRoutingKey;
return this;
}
/**
* This adds the response message type.
*
* @param responseMessageType
* The response message type.
*
* @return The AmqpProviderEndpointBuilder
builder.
*
* @since 1.0
*/
public AmqpProviderEndpointBuilder responseMessageType(final String responseMessageType)
{
this.responseMessageType = responseMessageType;
return this;
}
/**
* This adds the response message version.
*
* @param responseMessageVersion
* The response message version.
*
* @return The AmqpProviderEndpointBuilder
builder.
*
* @since 1.0
*/
public AmqpProviderEndpointBuilder responseMessageVersion(final String responseMessageVersion)
{
this.responseMessageVersion = responseMessageVersion;
return this;
}
/**
* This adds the name of the event exchange.
*
* @param eventExchange
* The name of the event exchange.
*
* @return The AmqpProviderEndpointBuilder
builder.
*
* @since 1.0
*/
public AmqpProviderEndpointBuilder eventExchange(final String eventExchange)
{
this.eventExchange = eventExchange;
return this;
}
/**
* This adds the type of the event exchange.
*
* @param eventExchangeType
* The type of the event exchange.
*
* @return The AmqpProviderEndpointBuilder
builder.
*
* @since 1.0
*/
public AmqpProviderEndpointBuilder eventExchangeType(final String eventExchangeType)
{
this.eventExchangeType = eventExchangeType;
return this;
}
/**
* This adds the event routing key.
*
* @param eventRoutingKey
* The event routing key.
*
* @return The AmqpProviderEndpointBuilder
builder.
*
* @since 1.0
*/
public AmqpProviderEndpointBuilder eventRoutingKey(final String eventRoutingKey)
{
this.eventRoutingKey = eventRoutingKey;
return this;
}
/**
* This adds the event message type.
*
* @param eventMessageType
* The event message type.
*
* @return The AmqpProviderEndpointBuilder
builder.
*
* @since 1.0
*/
public AmqpProviderEndpointBuilder eventMessageType(final String eventMessageType)
{
this.eventMessageType = eventMessageType;
return this;
}
/**
* This adds the event message version.
*
* @param eventMessageVersion
* The event message version.
*
* @return The AmqpProviderEndpointBuilder
builder.
*
* @since 1.0
*/
public AmqpProviderEndpointBuilder eventMessageVersion(final String eventMessageVersion)
{
this.eventMessageVersion = eventMessageVersion;
return this;
}
/**
* This builds the ProviderEndpoint
with AMQP as the protocol.
*
* @return The ProviderEndpoint
with AMQP as the protocol.
*
* @since 1.0
*/
public ProviderEndpoint build()
{
final ProviderEndpoint providerEndpoint = new ProviderEndpoint();
providerEndpoint.setProtocol(AMQP_PROTOCOL);
providerEndpoint.setType(this.type);
final List properties = new ArrayList();
this.addEndpointProperty(properties, REQUEST_EXCHANGE, this.requestExchange);
this.addEndpointProperty(properties, REQUEST_EXCHANGE_TYPE, this.requestExchangeType);
this.addEndpointProperty(properties, REQUEST_ROUTING_KEY, this.requestRoutingKey);
this.addEndpointProperty(properties, REQUEST_MESSAGE_TYPE, this.requestMessageType);
this.addEndpointProperty(properties, REQUEST_MESSAGE_VERSION, this.requestMessageVersion);
this.addEndpointProperty(properties, REQUEST_ACK_ROUTING_KEY, this.requestAckRoutingKey);
this.addEndpointProperty(properties, REQUEST_ACK_MESSAGE_TYPE, this.requestAckMessageType);
this.addEndpointProperty(properties, REQUEST_ACK_MESSAGE_VERSION, this.requestAckMessageVersion);
this.addEndpointProperty(properties, RESPONSE_EXCHANGE, this.responseExchange);
this.addEndpointProperty(properties, RESPONSE_EXCHANGE_TYPE, this.responseExchangeType);
this.addEndpointProperty(properties, RESPONSE_ROUTING_KEY, this.responseRoutingKey);
this.addEndpointProperty(properties, RESPONSE_MESSAGE_TYPE, this.responseMessageType);
this.addEndpointProperty(properties, RESPONSE_MESSAGE_VERSION, this.responseMessageVersion);
this.addEndpointProperty(properties, EVENT_EXCHANGE, this.eventExchange);
this.addEndpointProperty(properties, EVENT_EXCHANGE_TYPE, this.eventExchangeType);
this.addEndpointProperty(properties, EVENT_ROUTING_KEY, this.eventRoutingKey);
this.addEndpointProperty(properties, EVENT_MESSAGE_TYPE, this.eventMessageType);
this.addEndpointProperty(properties, EVENT_MESSAGE_VERSION, this.eventMessageVersion);
providerEndpoint.setEndpointProperties(properties);
return providerEndpoint;
}
/*
* This adds the endpoint property with the specified name and value to the list of properties.
* @param properties The list of properties.
* @param propertyName The name of the property.
* @param propertyValue The value of the property.
* @since 1.0
*/
private void addEndpointProperty(final List properties, final String propertyName, final String propertyValue)
{
// if the property value is null, don't add it to the properties list
if (propertyValue == null)
{
return;
}
properties.add(new EndpointProperty(propertyName, propertyValue));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy