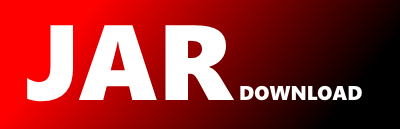
com.dell.cpsd.hdp.capability.registry.client.helper.AmqpProviderEndpointHelper Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of hdp-capability-registry-client Show documentation
Show all versions of hdp-capability-registry-client Show documentation
This repository contains the source code for the capability registry API.
This API exposes the interface through which a consumer or provider interacts with the capability registry.
The newest version!
/**
* Copyright © 2017 Dell Inc. or its subsidiaries. All Rights Reserved.
* Dell EMC Confidential/Proprietary Information
*/
package com.dell.cpsd.hdp.capability.registry.client.helper;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import com.dell.cpsd.hdp.capability.registry.api.EndpointProperty;
import com.dell.cpsd.hdp.capability.registry.api.ProviderEndpoint;
import com.dell.cpsd.hdp.capability.registry.client.builder.AmqpProviderEndpointBuilder;
/**
* This is a a helper class for a AMQP ProviderEndpoint
.
*
* Copyright © 2017 Dell Inc. or its subsidiaries. All Rights Reserved.
* Dell EMC Confidential/Proprietary Information
*
*
* @since 1.0
*/
public class AmqpProviderEndpointHelper
{
/**
* The map of endpoint properties.
*/
private Map endpointPropertyMap = null;
/**
* AmqpProviderEndpointHelper constructor.
*
* @param providerEndpoint
* The provider endpoint.
*
* @since 1.0
*/
public AmqpProviderEndpointHelper(final ProviderEndpoint providerEndpoint)
{
super();
this.endpointPropertyMap = this.extractEndpointProperties(providerEndpoint);
}
/**
* This returns the name of the request exchange.
*
* @return The name of the request exchange.
*
* @since 1.0
*/
public String getRequestExchange()
{
return this.getEndpointPropertyValue(AmqpProviderEndpointBuilder.REQUEST_EXCHANGE);
}
/**
* This returns the type of the request exchange.
*
* @return The type of the request exchange.
*
* @since 1.0
*/
public String getRequestExchangeType()
{
return this.getEndpointPropertyValue(AmqpProviderEndpointBuilder.REQUEST_EXCHANGE_TYPE);
}
/**
* This returns the request routing key.
*
* @return The request routing key.
*
* @since 1.0
*/
public String getRequestRoutingKey()
{
return this.getEndpointPropertyValue(AmqpProviderEndpointBuilder.REQUEST_ROUTING_KEY);
}
/**
* This returns the request message type.
*
* @return The request message type.
*
* @since 1.0
*/
public String getRequestMessageType()
{
return this.getEndpointPropertyValue(AmqpProviderEndpointBuilder.REQUEST_MESSAGE_TYPE);
}
/**
* This returns the request message version.
*
* @return The request message version.
*
* @since 1.0
*/
public String getRequestMessageVersion()
{
return this.getEndpointPropertyValue(AmqpProviderEndpointBuilder.REQUEST_MESSAGE_VERSION);
}
/**
* This returns the name of the response exchange.
*
* @return The name of the response exchange.
*
* @since 1.0
*/
public String getResponseExchange()
{
return this.getEndpointPropertyValue(AmqpProviderEndpointBuilder.RESPONSE_EXCHANGE);
}
/**
* This returns the type of the response exchange.
*
* @return The type of the response exchange.
*
* @since 1.0
*/
public String getResponseExchangeType()
{
return this.getEndpointPropertyValue(AmqpProviderEndpointBuilder.RESPONSE_EXCHANGE_TYPE);
}
/**
* This returns the response routing key.
*
* @return The response routing key.
*
* @since 1.0
*/
public String getResponseRoutingKey()
{
return this.getEndpointPropertyValue(AmqpProviderEndpointBuilder.RESPONSE_ROUTING_KEY);
}
/**
* This returns the response message type.
*
* @return The response message type.
*
* @since 1.0
*/
public String getResponseMessageType()
{
return this.getEndpointPropertyValue(AmqpProviderEndpointBuilder.RESPONSE_MESSAGE_TYPE);
}
/**
* This returns the response message version.
*
* @return The response message version.
*
* @since 1.0
*/
public String getResponseMessageVersion()
{
return this.getEndpointPropertyValue(AmqpProviderEndpointBuilder.RESPONSE_MESSAGE_VERSION);
}
/**
* This returns the name of the event exchange.
*
* @return The name of the event exchange.
*
* @since 1.0
*/
public String getEventExchange()
{
return this.getEndpointPropertyValue(AmqpProviderEndpointBuilder.EVENT_EXCHANGE);
}
/**
* This returns the type of the event exchange.
*
* @return The type of the event exchange.
*
* @since 1.0
*/
public String getEventExchangeType()
{
return this.getEndpointPropertyValue(AmqpProviderEndpointBuilder.EVENT_EXCHANGE_TYPE);
}
/**
* This returns the routing key for the events.
*
* @return The routing key for the events.
*
* @since 1.0
*/
public String getEventRoutingKey()
{
return this.getEndpointPropertyValue(AmqpProviderEndpointBuilder.EVENT_ROUTING_KEY);
}
/**
* This returns the event message type.
*
* @return The event message type.
*
* @since 1.0
*/
public String getEventMessageType()
{
return this.getEndpointPropertyValue(AmqpProviderEndpointBuilder.EVENT_MESSAGE_TYPE);
}
/**
* This returns the event message version.
*
* @return The event message version.
*
* @since 1.0
*/
public String getEventMessageVersion()
{
return this.getEndpointPropertyValue(AmqpProviderEndpointBuilder.EVENT_MESSAGE_VERSION);
}
/**
* This returns the value of a named endpoint property, or null.
*
* @param endpointPropertyName
* The endpoint property name
* @return The value of a named endpoint property, or null.
*
* @since 1.0
*/
protected String getEndpointPropertyValue(final String endpointPropertyName)
{
final EndpointProperty endpointProperty = this.endpointPropertyMap.get(endpointPropertyName);
if (endpointProperty == null)
{
return null;
}
return endpointProperty.getValue();
}
/**
* This converts the list of endpoint properties in the provider endpoint to a map of endpoint properties, keyed off the the property
* name.
*
* @param providerEndpoint
* The provider endpoint.
* @return The map of endpoint properties.
*
* @since 1.0
*/
protected Map extractEndpointProperties(final ProviderEndpoint providerEndpoint)
{
final Map newEndpointPropertyMap = new HashMap();
if (providerEndpoint == null)
{
return newEndpointPropertyMap;
}
final List endpointProperties = providerEndpoint.getEndpointProperties();
if (endpointProperties == null)
{
return newEndpointPropertyMap;
}
for (final EndpointProperty endpointProperty : endpointProperties)
{
if (endpointProperty != null)
{
newEndpointPropertyMap.put(endpointProperty.getName(), endpointProperty);
}
}
return newEndpointPropertyMap;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy