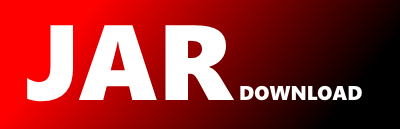
com.denimgroup.threadfix.cli.OptionsHolder Maven / Gradle / Ivy
////////////////////////////////////////////////////////////////////////
//
// Copyright (c) 2009-2016 Denim Group, Ltd.
//
// The contents of this file are subject to the Mozilla Public License
// Version 2.0 (the "License"); you may not use this file except in
// compliance with the License. You may obtain a copy of the License at
// http://www.mozilla.org/MPL/
//
// Software distributed under the License is distributed on an "AS IS"
// basis, WITHOUT WARRANTY OF ANY KIND, either express or implied. See the
// License for the specific language governing rights and limitations
// under the License.
//
// The Original Code is ThreadFix.
//
// The Initial Developer of the Original Code is Denim Group, Ltd.
// Portions created by Denim Group, Ltd. are Copyright (C)
// Denim Group, Ltd. All Rights Reserved.
//
// Contributor(s): Denim Group, Ltd.
//
////////////////////////////////////////////////////////////////////////
package com.denimgroup.threadfix.cli;
import org.apache.commons.cli.Option;
import org.apache.commons.cli.OptionBuilder;
import org.apache.commons.cli.Options;
public class OptionsHolder {
@SuppressWarnings("static-access")
public static Options getOptions() {
Options options = new Options();
Option property = OptionBuilder.withArgName( "unsafe-ssl" )
.hasArgs(1)
.withValueSeparator()
.withDescription( "unsafe-ssl to force ThreadFix to accept unsigned certificates." )
.create( "D" );
options.addOption(property);
Option teams = OptionBuilder.withLongOpt("teams")
.withDescription("Fetches a list of ThreadFix teams and applications.")
.create("t");
options.addOption(teams);
Option teamsPrettyPrint = OptionBuilder.withLongOpt("teamsPrettyPrint")
.withDescription("Fetches a human readable list of ThreadFix teams, applications, and application IDs.")
.create("tpp");
options.addOption(teamsPrettyPrint);
options.addOption(new Option("help", "Print this message" ));
Option set = OptionBuilder.withArgName("property> <[targetUrl]> <[scan profile Id]")
.hasArgs(4)
.withLongOpt("queueScan")
.withDescription("Queue a scan for the given applicationId with the given scanner type")
.create("q");
options.addOption(queueScan);
Option addAppUrl = OptionBuilder.withArgName("applicationId> <[tagType]")
.hasArgs(2)
.withLongOpt("create-tag")
.withDescription("Creates a ThreadFix Tag and returns its JSON. tagType is optional, default is Application Tag.")
.create("ctg");
options.addOption(createTag);
Option searchTag = OptionBuilder.withArgName("property> <[commentTagIds]")
.hasArgs(3)
.withLongOpt("add-comment")
.withDescription("Add comment to a vulnerability. CommentTagIds is optional, separated by comma.")
.create("ac");
options.addOption(addComment);
Option submitDefect = OptionBuilder.withArgName("applicationId> <[applicationDefectTrackerId]> <[vulnerabilityIds]> <[*]")
.hasArgs(1)
.hasOptionalArgs()
.withLongOpt("submit-defect")
.withDescription("Submit a defect to the defect tracker configured for a specific application.")
.create("sd");
options.addOption(submitDefect);
Option getDefectParameters = OptionBuilder.withArgName("applicationId> <[applicationDefectTrackerId]>")
.hasArgs(1)
.hasOptionalArgs()
.withLongOpt("get-defect-parameters")
.withDescription("Get a list of parameters from the defect tracker given an application ID.")
.create("gdp");
options.addOption(getDefectParameters);
Option newDefectTrackerParameters = OptionBuilder.withArgName("defectTrackerTypeId> ")
.hasArgs(3)
.hasOptionalArgs()
.withLongOpt("new-defect-tracker")
.withDescription("Create a new Defect Tracker with the given values.")
.create("dtn");
options.addOption(newDefectTrackerParameters);
Option listDefectTrackerProjectsParameters = OptionBuilder.withArgName("defectTrackerId")
.hasArgs(1)
.withLongOpt("list-defect-tracker-projects")
.withDescription("List the project names associated with this defect tracker.")
.create("dtp");
options.addOption(listDefectTrackerProjectsParameters);
Option listDefectTrackerTypesParameters = OptionBuilder
.withLongOpt("list-defect-tracker-types")
.withDescription("List the available defect tracker types.")
.create("dtt");
options.addOption(listDefectTrackerTypesParameters);
Option applicationSetDefectTracker = OptionBuilder.withArgName("applicationId> ")
.hasArgs(1)
.withLongOpt("unique-app-lookup")
.withDescription("Get a list of applications across teams that share the same unique ID.")
.create("ual");
options.addOption(lookupUniqueApps);
Option wafs = OptionBuilder.withLongOpt("wafs")
.withDescription("Fetches a list of ThreadFix WAFs.")
.create("w");
options.addOption(wafs);
Option uploadWafLog = OptionBuilder.withArgName("wafId> " +
" " +
" ")
.withLongOpt("scans")
.hasArgs(1)
.withDescription("Fetches a list of scans for an application.")
.create("sc");
options.addOption(getScans);
Option getScanDetail = OptionBuilder.withArgName("scanId>")
.withLongOpt("scan-details")
.hasArgs(1)
.withDescription("Fetches scan details for given scan ID.")
.create("sdt");
options.addOption(getScanDetail);
Option setCustomCweText = OptionBuilder.withArgName("cweId> ")
.withLongOpt("tag-apps")
.hasArgs(1)
.withDescription("List applications assigned to a tag.")
.create("tgap");
options.addOption(getAppsForTag);
//CICD
Option listCICDPolicies = OptionBuilder.hasArgs(0)
.withDescription("List CI/CD Policy file names.")
.withLongOpt("list-cicd-policies")
.create("lcicdp");
options.addOption(listCICDPolicies);
Option getCICDPolicy = OptionBuilder.hasArgs(1)
.withArgName("fileName")
.withDescription("Get CI/CD Policy with given name.")
.withLongOpt("get-cicd-policy")
.create("gcicdp");
options.addOption(getCICDPolicy);
Option uploadCICDPolicy = OptionBuilder.hasArgs(1)
.withArgName("filePath")
.withDescription("Upload CI/CD Policy.")
.withLongOpt("upload-cicd-policy")
.create("ucicdp");
options.addOption(uploadCICDPolicy);
Option deleteCICDPolicy = OptionBuilder.hasArgs(1)
.withArgName("fileName")
.withDescription("Upload CI/CD Policy.")
.withLongOpt("delete-cicd-policy")
.create("dcicdp");
options.addOption(deleteCICDPolicy);
return options;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy