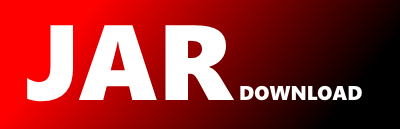
com.denimgroup.threadfix.remote.response.ResponseParser Maven / Gradle / Ivy
////////////////////////////////////////////////////////////////////////
//
// Copyright (c) 2009-2016 Denim Group, Ltd.
//
// The contents of this file are subject to the Mozilla Public License
// Version 2.0 (the "License"); you may not use this file except in
// compliance with the License. You may obtain a copy of the License at
// http://www.mozilla.org/MPL/
//
// Software distributed under the License is distributed on an "AS IS"
// basis, WITHOUT WARRANTY OF ANY KIND, either express or implied. See the
// License for the specific language governing rights and limitations
// under the License.
//
// The Original Code is ThreadFix.
//
// The Initial Developer of the Original Code is Denim Group, Ltd.
// Portions created by Denim Group, Ltd. are Copyright (C)
// Denim Group, Ltd. All Rights Reserved.
//
// Contributor(s): Denim Group, Ltd.
//
////////////////////////////////////////////////////////////////////////
package com.denimgroup.threadfix.remote.response;
import com.denimgroup.threadfix.logging.SanitizedLogger;
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
import com.google.gson.JsonSyntaxException;
import org.apache.commons.io.IOUtils;
import java.io.*;
import java.lang.reflect.Type;
import java.util.Calendar;
import java.util.Date;
import java.util.GregorianCalendar;
public class ResponseParser {
private static final SanitizedLogger LOGGER = new SanitizedLogger(ResponseParser.class);
private static Type getTypeReference() {
return new TypeReference>(){}.getType();
}
private static Gson getGson() {
GsonBuilder gsonBuilder = new GsonBuilder();
gsonBuilder.registerTypeAdapter(Calendar.class, new CalendarSerializer());
gsonBuilder.registerTypeAdapter(GregorianCalendar.class, new CalendarSerializer());
gsonBuilder.registerTypeAdapter(Date.class, new DateSerializer());
gsonBuilder.registerTypeAdapter(byte[].class, new ByteToStringSerializer()); // needed for files.
return gsonBuilder.create();
}
// TODO remove the double JSON read for efficiency
// I still think this will be IO-bound in most cases
@SuppressWarnings("unchecked") // the JSON String preservation broke this
public static RestResponse getRestResponse(String responseString, int responseCode, Class internalClass) {
LOGGER.debug("Parsing response for type " + internalClass.getCanonicalName());
RestResponse response = new RestResponse();
if (responseString != null && responseString.trim().indexOf('{') == 0) {
try {
Gson gson = getGson();
response = gson.fromJson(responseString, getTypeReference()); // turn everything into an object
String innerJson = gson.toJson(response.object); // turn the inner object back into a string
if (response.object instanceof String) {
// No need to do any more work
response.object = (T) innerJson;
LOGGER.debug("Parsed inner object as JSON String correctly.");
} else {
// turn the inner object into the correctly typed object
response.object = gson.fromJson(innerJson, internalClass);
LOGGER.debug("Parsed result into " + internalClass.getName() + " correctly.");
}
} catch (JsonSyntaxException e) {
LOGGER.error("Encountered JsonSyntaxException", e);
}
}
response.responseCode = responseCode;
response.jsonString = responseString;
LOGGER.debug("Setting response code to " + responseCode + ".");
return response;
}
public static RestResponse getRestResponse(InputStream responseStream, int responseCode, Class target) {
String inputString = null;
try {
inputString = IOUtils.toString(responseStream, "UTF-8");
} catch (IOException e) {
LOGGER.error("Unable to parse response stream due to IOException.", e);
}
return getRestResponse(inputString, responseCode, target);
}
public static RestResponse getFileResponse(InputStream in, int responseCode, File outputFile, String fileName) {
RestResponse instance = new RestResponse();
instance.responseCode = responseCode;
try {
if(outputFile.exists() || outputFile.mkdirs()) {
File f = new File(outputFile.getAbsolutePath() + '/' + fileName);
if (f.exists() || f.createNewFile()) {
FileOutputStream out = new FileOutputStream(f);
IOUtils.copy(in, out);
in.close();
out.flush();
out.close();
instance.success = true;
instance.message = "File saved successfully";
}
}
} catch (FileNotFoundException e) {
e.printStackTrace();
instance.success = false;
instance.message = "File path unreachable.";
} catch (IOException e) {
e.printStackTrace();
instance.success = false;
instance.message = "Error writing to given file path.";
}
return instance;
}
public static RestResponse getErrorResponse(String errorText, int responseCode) {
RestResponse instance = new RestResponse();
instance.message = errorText;
instance.responseCode = responseCode;
instance.success = false;
return instance;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy