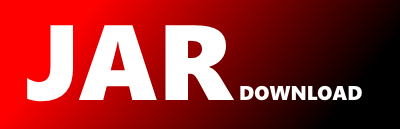
com.denimgroup.threadfix.ScannerUtils Maven / Gradle / Ivy
////////////////////////////////////////////////////////////////////////
//
// Copyright (c) 2009-2016 Denim Group, Ltd.
//
// The contents of this file are subject to the Mozilla Public License
// Version 2.0 (the "License"); you may not use this file except in
// compliance with the License. You may obtain a copy of the License at
// http://www.mozilla.org/MPL/
//
// Software distributed under the License is distributed on an "AS IS"
// basis, WITHOUT WARRANTY OF ANY KIND, either express or implied. See the
// License for the specific language governing rights and limitations
// under the License.
//
// The Original Code is ThreadFix.
//
// The Initial Developer of the Original Code is Denim Group, Ltd.
// Portions created by Denim Group, Ltd. are Copyright (C)
// Denim Group, Ltd. All Rights Reserved.
//
// Contributor(s): Denim Group, Ltd.
//
////////////////////////////////////////////////////////////////////////
package com.denimgroup.threadfix;
import com.denimgroup.threadfix.logging.SanitizedLogger;
import javax.annotation.Nonnull;
import java.math.BigInteger;
import java.security.MessageDigest;
import java.security.NoSuchAlgorithmException;
/**
* This provides a place for utilities that are useful across scan importers
* Created by mcollins on 11/10/15.
*/
public class ScannerUtils {
private static final SanitizedLogger log = new SanitizedLogger(ScannerUtils.class);
private ScannerUtils(){}
/**
* This method hashes whatever string is given to it in md5
* DON'T use when cryptographic strength is important.
* @param input
* @return
*/
public static String md5(String input) {
String result = input;
if (input != null) {
MessageDigest md;
try {
md = MessageDigest.getInstance("MD5");
} catch (NoSuchAlgorithmException e) {
throw new IllegalStateException("Can't find MD5 algorithm.", e);
}
md.update(input.getBytes());
BigInteger hash = new BigInteger(1, md.digest());
result = hash.toString(16);
while (result.length() < 32) {
result = "0" + result;
}
}
return result;
}
/**
* Hashes whatever three strings are given to it.
*
* @param type
* The generic, CWE type of vulnerability.
* @param url
* The URL location of the vulnerability.
* @param param
* The vulnerable parameter (optional)
* @return The three strings concatenated, downcased, trimmed, and hashed.
*/
@Nonnull
public static String hashFindingInfo(String type, String url, String param) {
StringBuffer toHash = new StringBuffer();
if (type != null) {
toHash = toHash.append(type.toLowerCase().trim());
}
if (url != null) {
if (url.indexOf('/') == 0 || url.indexOf('\\') == 0) {
toHash = toHash.append(url.substring(1).toLowerCase().trim());
} else {
toHash = toHash.append(url.toLowerCase().trim());
}
}
if (param != null) {
toHash = toHash.append(param.toLowerCase().trim());
}
try {
MessageDigest messageDigest = MessageDigest.getInstance("MD5");
messageDigest.update(toHash.toString().getBytes(), 0, toHash.length());
String hash = new BigInteger(1, messageDigest.digest()).toString(16);
log.debug("Hash: " + hash);
return hash;
} catch (NoSuchAlgorithmException e) {
log.error("Can't find MD5 hash function to hash finding info", e);
throw new IllegalStateException("MD5 library couldn't be loaded.");
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy