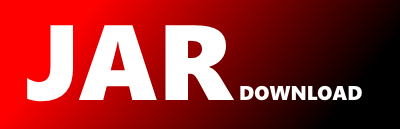
com.denimgroup.threadfix.data.entities.ChannelVulnerability Maven / Gradle / Ivy
////////////////////////////////////////////////////////////////////////
//
// Copyright (c) 2009-2016 Denim Group, Ltd.
//
// The contents of this file are subject to the Mozilla Public License
// Version 2.0 (the "License"); you may not use this file except in
// compliance with the License. You may obtain a copy of the License at
// http://www.mozilla.org/MPL/
//
// Software distributed under the License is distributed on an "AS IS"
// basis, WITHOUT WARRANTY OF ANY KIND, either express or implied. See the
// License for the specific language governing rights and limitations
// under the License.
//
// The Original Code is ThreadFix.
//
// The Initial Developer of the Original Code is Denim Group, Ltd.
// Portions created by Denim Group, Ltd. are Copyright (C)
// Denim Group, Ltd. All Rights Reserved.
//
// Contributor(s): Denim Group, Ltd.
//
////////////////////////////////////////////////////////////////////////
package com.denimgroup.threadfix.data.entities;
import com.denimgroup.threadfix.views.AllViews;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonView;
import org.hibernate.annotations.Filter;
import org.hibernate.validator.constraints.NotEmpty;
import javax.persistence.*;
import javax.validation.constraints.Size;
import java.util.Collections;
import java.util.List;
import static com.denimgroup.threadfix.CollectionUtils.list;
@Entity
@Table(name = "ChannelVulnerability")
public class ChannelVulnerability extends BaseEntity {
private static final long serialVersionUID = 2461270529594171704L;
private ChannelType channelType;
private Boolean userCreated = false;
@Column(nullable = true)
public Boolean getUserCreated() {
return userCreated != null && userCreated;
}
public void setUserCreated(Boolean userCreated) {
this.userCreated = userCreated;
}
@NotEmpty(message = "{errors.required}")
@Size(max = 150, message = "{errors.maxlength}")
private String name;
@Size(max = 150, message = "{errors.maxlength}")
private String code;
private List findings;
private List vulnerabilityMaps;
private List vulnerabilityMapHistories;
@ManyToOne
@JoinColumn(name = "channelTypeId", foreignKey = @ForeignKey(name="FKE09C785947A56B56"))
@JsonView(AllViews.MappedChannelVulnView.class)
public ChannelType getChannelType() {
return channelType;
}
public void setChannelType(ChannelType channelType) {
this.channelType = channelType;
}
@Column(length = 150, nullable = false)
@JsonView(Object.class)
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
@Column(length = 150, nullable = false)
@JsonView(AllViews.VulnerabilityDetail.class)
public String getCode() {
return code;
}
public void setCode(String code) {
this.code = code;
}
@OneToMany(mappedBy = "channelVulnerability")
@JsonIgnore
public List getFindings() {
return findings;
}
public void setFindings(List findings) {
this.findings = findings;
}
@OneToMany(mappedBy = "channelVulnerability", cascade = CascadeType.ALL)
@JsonIgnore
public List getVulnerabilityMaps() {
return vulnerabilityMaps;
}
public void setVulnerabilityMaps(List vulnerabilityMaps) {
this.vulnerabilityMaps = vulnerabilityMaps;
}
@OneToMany(mappedBy = "channelVulnerability", cascade = CascadeType.ALL)
@JsonView(AllViews.MappedChannelVulnView.class)
public List getVulnerabilityMapHistories() {
if (vulnerabilityMapHistories == null) vulnerabilityMapHistories = list();
Collections.sort(vulnerabilityMapHistories);
return vulnerabilityMapHistories;
}
public void setVulnerabilityMapHistories(List vulnerabilityMapHistories) {
this.vulnerabilityMapHistories = vulnerabilityMapHistories;
}
@Transient
@JsonView({ AllViews.VulnerabilityDetail.class, AllViews.MappedChannelVulnView.class })
public GenericVulnerability getGenericVulnerability() {
if (vulnerabilityMaps != null && vulnerabilityMaps.size() != 0
&& vulnerabilityMaps.get(0) != null) {
return vulnerabilityMaps.get(0).getGenericVulnerability();
} else {
return null;
}
}
@Transient
@JsonView({ AllViews.VulnerabilityDetail.class, AllViews.MappedChannelVulnView.class })
public boolean getEditableMapping() {
return !(this.getVulnerabilityMaps() != null
&& this.getVulnerabilityMaps().size() != 0
&& !this.getUserCreated());
}
@Override
public String toString() {
return "ChannelVulnerability{" +
"name='" + name + '\'' +
'}';
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy