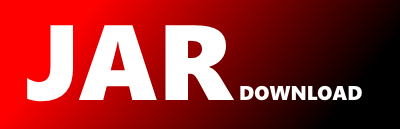
com.denimgroup.threadfix.data.entities.Organization Maven / Gradle / Ivy
////////////////////////////////////////////////////////////////////////
//
// Copyright (c) 2009-2016 Denim Group, Ltd.
//
// The contents of this file are subject to the Mozilla Public License
// Version 2.0 (the "License"); you may not use this file except in
// compliance with the License. You may obtain a copy of the License at
// http://www.mozilla.org/MPL/
//
// Software distributed under the License is distributed on an "AS IS"
// basis, WITHOUT WARRANTY OF ANY KIND, either express or implied. See the
// License for the specific language governing rights and limitations
// under the License.
//
// The Original Code is ThreadFix.
//
// The Initial Developer of the Original Code is Denim Group, Ltd.
// Portions created by Denim Group, Ltd. are Copyright (C)
// Denim Group, Ltd. All Rights Reserved.
//
// Contributor(s): Denim Group, Ltd.
//
////////////////////////////////////////////////////////////////////////
package com.denimgroup.threadfix.data.entities;
import com.denimgroup.threadfix.CollectionUtils;
import com.denimgroup.threadfix.views.AllViews;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonView;
import org.hibernate.annotations.LazyCollection;
import org.hibernate.annotations.LazyCollectionOption;
import org.hibernate.validator.constraints.NotEmpty;
import javax.persistence.*;
import javax.validation.constraints.Size;
import java.util.ArrayList;
import java.util.List;
@Entity
@Table(name = "Organization")
public class Organization extends AuditableEntity {
// These are used for caching and will require frequent updates.
private Integer infoVulnCount = 0, lowVulnCount = 0, mediumVulnCount = 0,
highVulnCount = 0, criticalVulnCount = 0, totalVulnCount = 0;
private static final long serialVersionUID = 6734388139007659988L;
private List activeApps;
private List accessControlTeamMaps;
private List events;
private List scheduledEmailReports;
public static final int NAME_LENGTH = 60;
@NotEmpty(message = "{errors.required}")
@Size(max = NAME_LENGTH, message = "{errors.maxlength} " + NAME_LENGTH + ".")
private String name;
private List applications;
private List surveyResults;
@Column(length = NAME_LENGTH, nullable = false)
@JsonView(Object.class) // This means it will be included in all ObjectWriters with Views.
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
@OneToMany(mappedBy = "organization")
@OrderBy("name")
@JsonIgnore
public List getApplications() {
return applications;
}
public void setApplications(List applications) {
this.applications = applications;
}
@OneToMany(cascade = { CascadeType.PERSIST, CascadeType.MERGE }, mappedBy = "organization", fetch = FetchType.LAZY)
@OrderBy("createdDate DESC")
@LazyCollection(LazyCollectionOption.EXTRA)
@JsonIgnore
public List getSurveyResults() {
return surveyResults;
}
public void setSurveyResults(List surveyResults) {
this.surveyResults = surveyResults;
}
@OneToMany(mappedBy = "organization", cascade = CascadeType.ALL, fetch = FetchType.LAZY)
@LazyCollection(LazyCollectionOption.EXTRA)
@JsonIgnore
public List getAccessControlTeamMaps() {
return accessControlTeamMaps;
}
@Transient
@JsonView({AllViews.TableRow.class, AllViews.GRCToolsPage.class, AllViews.RestViewTeam2_1.class, AllViews.RestViewTeams2_4.class,
AllViews.RestViewTeams2_1.class, AllViews.VulnSearchApplications.class })
@JsonProperty("applications")
public List getActiveApplications() {
if (activeApps == null && this.applications != null) {
activeApps = new ArrayList();
for (Application application : this.applications) {
if (application.isActive())
activeApps.add(application);
}
}
return activeApps;
}
// This can be used to set temporary filtered lists of apps for a team
public void setActiveApplications(List apps) {
activeApps = apps;
}
public void setAccessControlTeamMaps(List accessControlTeamMaps) {
this.accessControlTeamMaps = accessControlTeamMaps;
}
// TODO this might belong somewhere else
/*
* Index Severity 0 Info 1 Low 2 Medium 3 High 4 Critical 5 # Total vulns
*/
public void updateVulnerabilityReport() {
int info = 0, low = 0, medium = 0, high = 0, critical = 0, total = 0;
for (Application app : this.applications) {
if (app != null && app.isActive()) {
info += app.getInfoVulnCount();
low += app.getLowVulnCount();
medium += app.getMediumVulnCount();
high += app.getHighVulnCount();
critical += app.getCriticalVulnCount();
total += app.getTotalVulnCount();
}
}
setInfoVulnCount(info);
setLowVulnCount(low);
setMediumVulnCount(medium);
setHighVulnCount(high);
setCriticalVulnCount(critical);
setTotalVulnCount(total);
}
@Column
@JsonView({ AllViews.RestViewTeam2_1.class, AllViews.RestViewTeams2_1.class, AllViews.RestViewTeams2_4.class,
AllViews.TableRow.class, AllViews.ApplicationIndexView.class })
public Integer getTotalVulnCount() {
return totalVulnCount;
}
public void setTotalVulnCount(Integer totalVulnCount) {
this.totalVulnCount = totalVulnCount;
}
@Column
@JsonView({ AllViews.RestViewTeam2_1.class, AllViews.RestViewTeams2_1.class,AllViews.RestViewTeams2_4.class,
AllViews.TableRow.class, AllViews.ApplicationIndexView.class })
public Integer getInfoVulnCount() {
return infoVulnCount;
}
public void setInfoVulnCount(Integer infoVulnCount) {
this.infoVulnCount = infoVulnCount;
}
@Column
@JsonView({ AllViews.RestViewTeam2_1.class, AllViews.RestViewTeams2_1.class, AllViews.RestViewTeams2_4.class,
AllViews.TableRow.class, AllViews.ApplicationIndexView.class })
public Integer getLowVulnCount() {
return lowVulnCount;
}
public void setLowVulnCount(Integer lowVulnCount) {
this.lowVulnCount = lowVulnCount;
}
@Column
@JsonView({ AllViews.RestViewTeam2_1.class, AllViews.RestViewTeams2_1.class, AllViews.RestViewTeams2_4.class,
AllViews.TableRow.class, AllViews.ApplicationIndexView.class })
public Integer getMediumVulnCount() {
return mediumVulnCount;
}
public void setMediumVulnCount(Integer mediumVulnCount) {
this.mediumVulnCount = mediumVulnCount;
}
@Column
@JsonView({ AllViews.RestViewTeam2_1.class, AllViews.RestViewTeams2_1.class, AllViews.RestViewTeams2_4.class,
AllViews.TableRow.class, AllViews.ApplicationIndexView.class })
public Integer getHighVulnCount() {
return highVulnCount;
}
public void setHighVulnCount(Integer highVulnCount) {
this.highVulnCount = highVulnCount;
}
@Column
@JsonView({ AllViews.RestViewTeams2_1.class, AllViews.RestViewTeam2_1.class, AllViews.RestViewTeams2_4.class,
AllViews.TableRow.class, AllViews.ApplicationIndexView.class })
public Integer getCriticalVulnCount() {
return criticalVulnCount;
}
public void setCriticalVulnCount(Integer criticalVulnCount) {
this.criticalVulnCount = criticalVulnCount;
}
@Transient
@JsonView({ AllViews.ApplicationIndexView.class })
public Integer getNumApps(){
List activeApps = getActiveApplications();
return (activeApps == null) ? 0 : activeApps.size();
}
@Transient
@JsonIgnore
public List getActiveAppIds(){
List applicationIdList = CollectionUtils.list();
for (Application application : getActiveApplications()) {
if (application != null) {
applicationIdList.add(application.getId());
}
}
return applicationIdList;
}
@ManyToMany(mappedBy = "organizations", fetch = FetchType.LAZY)
@LazyCollection(LazyCollectionOption.EXTRA)
@JsonIgnore
public List getScheduledEmailReports() {
return scheduledEmailReports;
}
public void setScheduledEmailReports(List scheduledEmailReports) {
this.scheduledEmailReports = scheduledEmailReports;
}
@Override
public String toString() {
return name == null ? "Unnamed Role" : name + " (Role)";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy